How to Get Arduino Length of Array using the sizeof() Function
-
Understanding the
sizeof()
Function - Practical Example of Using sizeof()
- Using sizeof() with Multi-Dimensional Arrays
- Common Pitfalls When Using sizeof()
- Conclusion
- FAQ
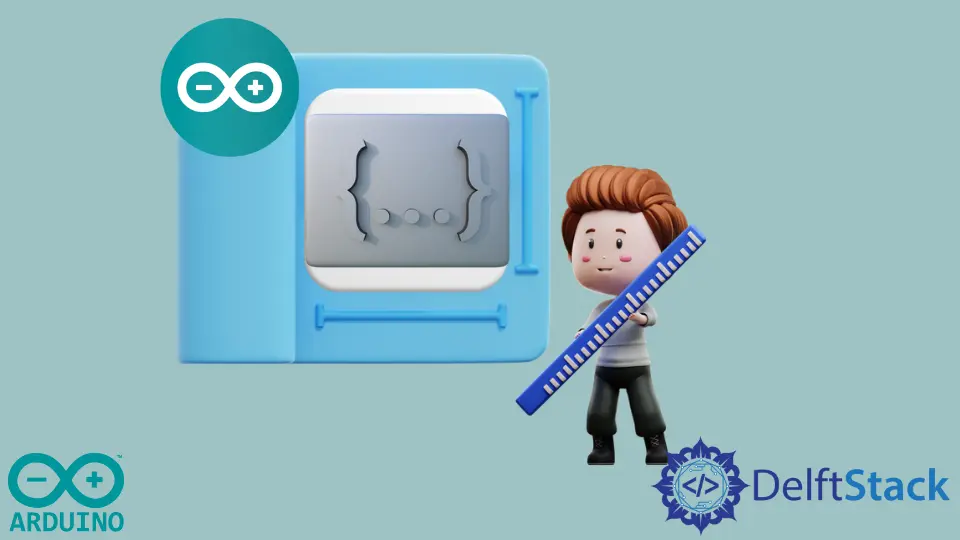
Understanding how to manipulate arrays is essential for any Arduino enthusiast. One of the most common tasks is determining the length of an array. In Arduino programming, the sizeof()
function is a handy tool that allows you to find out how many elements are in an array. This function calculates the total size of the array in bytes and divides it by the size of an individual element to give you the total count.
In this article, we’ll explore how to effectively use the sizeof()
function to get the length of arrays in your Arduino projects. Whether you’re a beginner or an experienced coder, this guide will provide you with clear examples and explanations to enhance your understanding.
Understanding the sizeof()
Function
The sizeof()
function in Arduino is a built-in operator that returns the size of a variable or data type in bytes. When applied to an array, it can be used to determine the total number of elements it contains. The syntax is straightforward: sizeof(array) / sizeof(array[0])
. Here, sizeof(array)
gives the total size of the array in bytes, while sizeof(array[0])
provides the size of the first element in the array. By dividing these two values, you get the length of the array.
Let’s look at an example to clarify how this works.
int myArray[] = {10, 20, 30, 40, 50};
int length = sizeof(myArray) / sizeof(myArray[0]);
In this snippet, we define an integer array myArray
with five elements. The length
variable will store the result of the sizeof()
operation, which calculates how many elements are in myArray
.
Output:
5
In this case, the output 5
indicates that there are five elements in myArray
.
Practical Example of Using sizeof()
To further illustrate the use of the sizeof()
function, let’s consider a more complex example involving an array of floating-point numbers. This will help you understand how to apply the concept in different scenarios.
float sensorReadings[] = {23.5, 25.1, 22.8, 24.0, 26.3, 27.5};
int numReadings = sizeof(sensorReadings) / sizeof(sensorReadings[0]);
Here, we have an array called sensorReadings
that stores six floating-point values. By applying the sizeof()
function as before, we can find out how many readings we have.
Output:
6
The output 6
shows that our sensorReadings
array contains six values. This method is particularly useful when dealing with dynamic data or when the size of the array may change during the program’s execution.
Using sizeof() with Multi-Dimensional Arrays
The sizeof()
function can also be used with multi-dimensional arrays. This is a bit more complex but equally important for managing data in Arduino. Let’s break it down with an example.
int matrix[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
int rows = sizeof(matrix) / sizeof(matrix[0]);
int cols = sizeof(matrix[0]) / sizeof(matrix[0][0]);
In this code, we define a 3x4 integer matrix. We can find the number of rows and columns using the sizeof()
function. The variable rows
will hold the number of rows, while cols
will hold the number of columns.
Output:
Rows: 3
Cols: 4
The output indicates that our matrix consists of 3 rows and 4 columns. This method is particularly useful for handling complex data structures in your Arduino projects, allowing you to efficiently manage and manipulate arrays.
Common Pitfalls When Using sizeof()
While the sizeof()
function is a powerful tool, there are common pitfalls to be aware of. One of the most significant issues arises when you pass an array to a function. In C and C++, when you pass an array to a function, it decays into a pointer, and sizeof()
will not return the expected size. Instead, it will return the size of the pointer type, which is usually 4 or 8 bytes depending on the architecture.
To avoid this issue, it’s a good practice to pass the size of the array as an additional parameter to the function. Here’s a quick example of how to handle this:
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
Serial.println(arr[i]);
}
}
void setup() {
int myArray[] = {1, 2, 3, 4, 5};
printArray(myArray, sizeof(myArray) / sizeof(myArray[0]));
}
In this example, we define a function printArray
that takes an array and its size as parameters. This way, we ensure that we always have the correct size available for processing the array.
Output:
1
2
3
4
5
This output shows that the function successfully prints each element of the array. By passing the size explicitly, we avoid the potential pitfalls of the sizeof()
function when used with arrays.
Conclusion
The sizeof()
function is a powerful ally when working with arrays in Arduino programming. It allows you to easily determine the length of arrays, whether they are one-dimensional or multi-dimensional. By understanding how to effectively use this function, you can enhance your coding skills and make your Arduino projects more efficient. Remember to be cautious when passing arrays to functions, as this can lead to unexpected results. With the knowledge gained from this article, you’re now equipped to handle arrays with confidence in your future Arduino endeavors.
FAQ
-
What is the
sizeof()
function in Arduino?
thesizeof()
function returns the size of a variable or data type in bytes, and when used with arrays, it helps determine the number of elements. -
Can I use sizeof() with multi-dimensional arrays?
Yes, you can use sizeof() with multi-dimensional arrays to calculate the number of rows and columns. -
What happens when I pass an array to a function?
When an array is passed to a function, it decays into a pointer, and sizeof() will return the size of the pointer, not the array. -
How can I avoid issues with sizeof() in functions?
You can avoid issues by passing the size of the array as an additional parameter to the function. -
Is sizeof() the only way to determine the length of an array in Arduino?
While sizeof() is the most common method, you can also use other techniques, such as maintaining a separate variable to track the array length.