Arduino memset() 函数
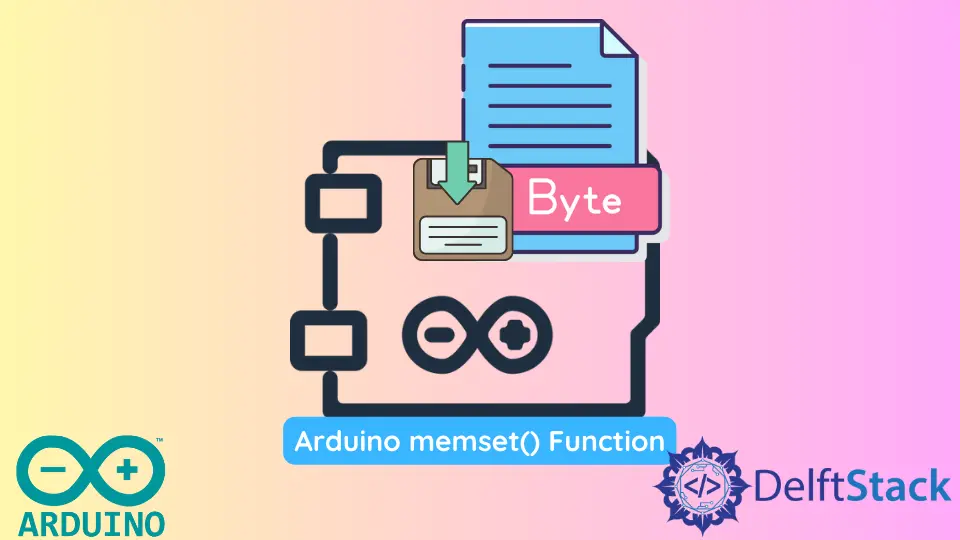
本教程将讨论使用 Arduino 中的 memset()
函数将值的字节设置为目标。
Arduino 中的 memset()
函数
memset()
函数将值的字节设置为 Arduino 中的目标。该函数从给定值中获取特定数量的字节并将其存储在目标中。
语法:
memset(destination, value, N_bytes);
在上述语法中,memset()
函数将获取给定值的第一个 N_bytes
并将其存储在 destination
中。如果值是整数或任何其他数据类型,它将被转换为字节数据类型。
我们将创建一个整数数组并使用 memset()
函数更改其值。
代码:
int ch1[4] = {1, 2, 3, 4};
void setup() {
Serial.begin(9600);
memset(ch1, 2, 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
Serial.println(ch1[i]);
}
}
void loop() {}
输出:
514
514
514
514
我们定义了一个整数数组并使用 memset()
函数将所有四个元素替换为整数 2
的字节。我们使用循环在 Arduino IDE 的串行监视器窗口上打印数组的值。
我们还可以使用 int()
函数将字节值转换为整数。我们必须将每个数组值存储到一个字节变量中,然后将其传递给 int()
函数以将其转换为整数。
让我们将上面的数组值转换为整数。
代码:
int ch1[4] = {1, 2, 3, 4};
void setup() {
Serial.begin(9600);
memset(ch1, 2, 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
int c = int(b);
Serial.println(c);
}
}
void loop() {}
输出:
2
2
2
2
字节值已转换为整数,但它们并未存储在原始数组中。我们也可以使用 memset()
函数对字符串和字符执行相同的操作。
我们可以定义一个字符数组,并使用 memset()
函数将它们替换为另一个字符。
使用 memset()
函数后,我们将获得一个包含字符的字节值的数组,但我们可以使用 char()
函数将该值转换回字符。
我们必须将每个字节值存储在一个字节变量中,然后将其传递给 char()
函数以将其转换为字符。让我们定义一个整数数组,存储字符值,然后用另一个替换它们。
代码:
int ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
memset(ch1, '.', 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
char c = char(b);
Serial.println(c);
}
}
void loop() {}
输出:
.
.
.
.
在第三个参数中,我们相乘的整数定义了我们要在数组中替换的值的数量。如果我们不想替换所有值而只想替换数组中的一些值,我们必须在 memset()
函数的第一个参数中添加一个整数。
假设我们只想替换给定数组的第二个和第三个值。我们必须在第一个参数中添加 1
,并在第三个参数中将其乘以 2
。
代码:
int ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
memset(ch1 + 1, '.', 2 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
char c = char(b);
Serial.println(c);
}
}
void loop() {}
输出:
a
.
.
d
仅替换数组的中间元素。我们还可以使用循环将值存储在数组中。
在下面的示例中,让我们使用 Arduino 中的循环来执行上述操作。
代码:
char ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
for (int i = 1; i < 3; i++) {
ch1[i] = '.';
}
for (int i = 0; i < 4; i++) {
Serial.println(ch1[i]);
}
}
void loop() {}
输出:
a
.
.
d
在上面的代码中,我们将数组定义为 char
数据类型,使用循环替换元素,并使用另一个循环打印数组元素。如果我们使用 int
来声明给定的数组,字符将被转换为 ASCII 表示。
我们必须使用循环来打印数组的值,但是在字符串的情况下我们可以不使用循环来打印它。我们可以使用循环替换字符串中存在的字符。
我们必须使用索引获取要替换的字符,然后使用另一个字符替换它们。让我们定义一个字符串并用另一个替换它的一些字符。
代码:
String ch1 = "hello world";
void setup() {
Serial.begin(9600);
for (int i = 1; i < 5; i++) {
ch1[i] = '.';
}
Serial.println(ch1);
}
void loop() {}
输出:
h.... world
给定字符串的某些字符被另一个字符替换。在 memset()
函数的情况下,我们使用 int
数据类型定义给定数组,因为该函数不适用于其他数据类型数组。