Arduino memset() 函式
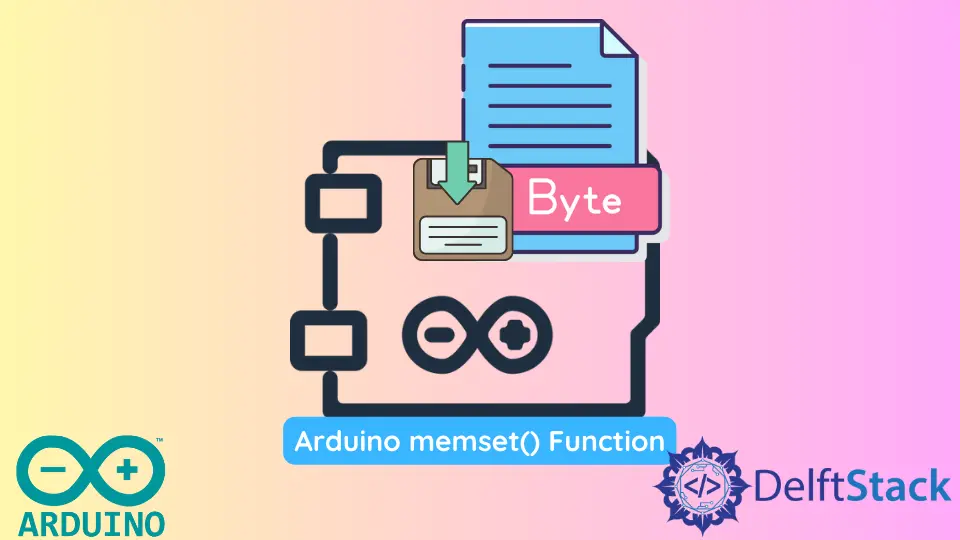
本教程將討論使用 Arduino 中的 memset()
函式將值的位元組設定為目標。
Arduino 中的 memset()
函式
memset()
函式將值的位元組設定為 Arduino 中的目標。該函式從給定值中獲取特定數量的位元組並將其儲存在目標中。
語法:
memset(destination, value, N_bytes);
在上述語法中,memset()
函式將獲取給定值的第一個 N_bytes
並將其儲存在 destination
中。如果值是整數或任何其他資料型別,它將被轉換為位元組資料型別。
我們將建立一個整數陣列並使用 memset()
函式更改其值。
程式碼:
int ch1[4] = {1, 2, 3, 4};
void setup() {
Serial.begin(9600);
memset(ch1, 2, 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
Serial.println(ch1[i]);
}
}
void loop() {}
輸出:
514
514
514
514
我們定義了一個整數陣列並使用 memset()
函式將所有四個元素替換為整數 2
的位元組。我們使用迴圈在 Arduino IDE 的序列監視器視窗上列印陣列的值。
我們還可以使用 int()
函式將位元組值轉換為整數。我們必須將每個陣列值儲存到一個位元組變數中,然後將其傳遞給 int()
函式以將其轉換為整數。
讓我們將上面的陣列值轉換為整數。
程式碼:
int ch1[4] = {1, 2, 3, 4};
void setup() {
Serial.begin(9600);
memset(ch1, 2, 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
int c = int(b);
Serial.println(c);
}
}
void loop() {}
輸出:
2
2
2
2
位元組值已轉換為整數,但它們並未儲存在原始陣列中。我們也可以使用 memset()
函式對字串和字元執行相同的操作。
我們可以定義一個字元陣列,並使用 memset()
函式將它們替換為另一個字元。
使用 memset()
函式後,我們將獲得一個包含字元的位元組值的陣列,但我們可以使用 char()
函式將該值轉換回字元。
我們必須將每個位元組值儲存在一個位元組變數中,然後將其傳遞給 char()
函式以將其轉換為字元。讓我們定義一個整數陣列,儲存字元值,然後用另一個替換它們。
程式碼:
int ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
memset(ch1, '.', 4 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
char c = char(b);
Serial.println(c);
}
}
void loop() {}
輸出:
.
.
.
.
在第三個引數中,我們相乘的整數定義了我們要在陣列中替換的值的數量。如果我們不想替換所有值而只想替換陣列中的一些值,我們必須在 memset()
函式的第一個引數中新增一個整數。
假設我們只想替換給定陣列的第二個和第三個值。我們必須在第一個引數中新增 1
,並在第三個引數中將其乘以 2
。
程式碼:
int ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
memset(ch1 + 1, '.', 2 * sizeof(ch1[1]));
for (int i = 0; i < 4; i++) {
byte b = ch1[i];
char c = char(b);
Serial.println(c);
}
}
void loop() {}
輸出:
a
.
.
d
僅替換陣列的中間元素。我們還可以使用迴圈將值儲存在陣列中。
在下面的示例中,讓我們使用 Arduino 中的迴圈來執行上述操作。
程式碼:
char ch1[4] = {'a', 'b', 'c', 'd'};
void setup() {
Serial.begin(9600);
for (int i = 1; i < 3; i++) {
ch1[i] = '.';
}
for (int i = 0; i < 4; i++) {
Serial.println(ch1[i]);
}
}
void loop() {}
輸出:
a
.
.
d
在上面的程式碼中,我們將陣列定義為 char
資料型別,使用迴圈替換元素,並使用另一個迴圈列印陣列元素。如果我們使用 int
來宣告給定的陣列,字元將被轉換為 ASCII 表示。
我們必須使用迴圈來列印陣列的值,但是在字串的情況下我們可以不使用迴圈來列印它。我們可以使用迴圈替換字串中存在的字元。
我們必須使用索引獲取要替換的字元,然後使用另一個字元替換它們。讓我們定義一個字串並用另一個替換它的一些字元。
程式碼:
String ch1 = "hello world";
void setup() {
Serial.begin(9600);
for (int i = 1; i < 5; i++) {
ch1[i] = '.';
}
Serial.println(ch1);
}
void loop() {}
輸出:
h.... world
給定字串的某些字元被另一個字元替換。在 memset()
函式的情況下,我們使用 int
資料型別定義給定陣列,因為該函式不適用於其他資料型別陣列。