How to Return Array From Function in Arduino
- Modify an Existing Array to Return Array From Function in Arduino
- Use Static Arrays to Return Array From Function in Arduino
- Use Dynamic Memory Allocation to Return Array From Function in Arduino
- Use Static Global Arrays to Return Array From Function in Arduino
- Use Reference Parameters to Return Array From Function in Arduino
- Conclusion
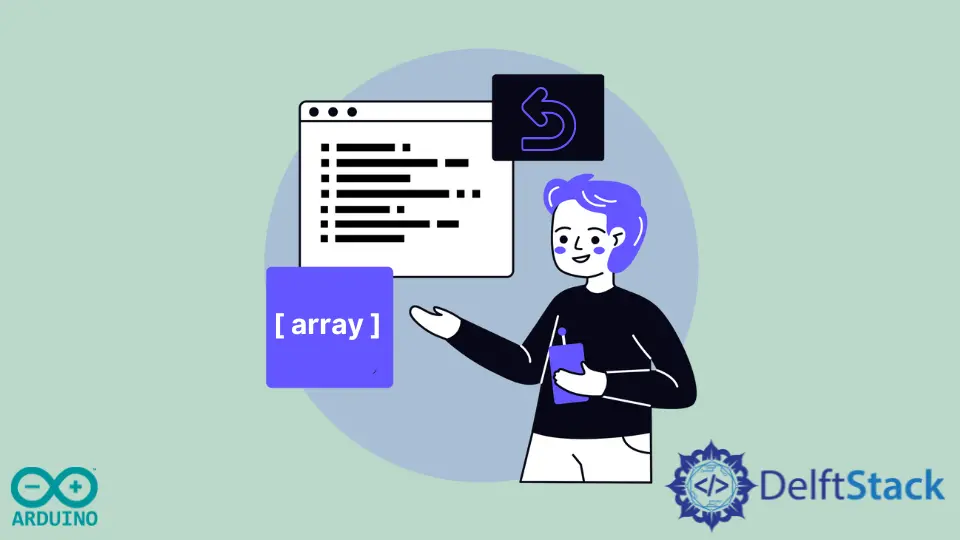
Returning an array from a function in Arduino might seem challenging due to the limitations of C/C++ regarding passing and returning arrays by value. However, several techniques and strategies can be employed to effectively handle this situation.
Let’s explore various methods to return arrays from functions in Arduino, along with illustrative code examples for each approach. This tutorial will also discuss returning an array from a function by modifying an existing array in Arduino.
Modify an Existing Array to Return Array From Function in Arduino
In Arduino, we can initialize an array with a given size; after initializing an array, we can add or replace the values of the array using a function.
If we want to initialize and create an array inside a function and then return it when the function is called, we have to use the dynamic memory allocation, which is done using the malloc()
and free()
functions, and we also have to use the pointers in Arduino.
The problem is if we create and return an array from a function using dynamic memory allocation, the result might change because of memory leakage and dangling pointers. So, it is best to initialize an array and change its values using a function rather than returning a whole array from a function.
For example, let’s initialize an array with a constant integer size and then create a function to change the array values. See the code below.
const int size = 10;
int MyArray[size];
void CreateArray() {
for (int i = 0; i < size; i++) {
MyArray[i] = i;
}
}
void setup() {
Serial.begin(9600);
CreateArray();
for (int i = 0; i < size; i++) {
Serial.println(MyArray[i]);
}
}
void loop() {}
Output:
0
1
2
3
4
5
6
7
8
9
In the above code, we initialized the array using a constant integer because we have to use a constant integer to initialize an array, and if we don’t use a constant integer, Arduino will show an error. We have not stored any values in the array, but we can also store values when we initialize an array.
If we don’t store any values in an array, the array will be initialized with NULL
values. We created the CreateArray()
function to store values in the array.
The CreateArray()
return type is void
, meaning we don’t want to return anything because we only want to store values in the array. We can change the return type if we want to return something; like, if we want to return an integer value, we can define the int
return type.
We used a for
loop to store values inside the array, but we can also manually store values inside the array. We called the CreateArray()
function inside the setup()
function to change the array’s values before we used it to print them.
We used the serial monitor of Arduino to print the values of the array, and as we can see in the output, ten values are present in the array.
Use Static Arrays to Return Array From Function in Arduino
One way to return an array from a function in Arduino is by using static arrays. Although it’s not possible to directly return an array by value in C/C++, returning a pointer to a statically declared array is feasible. This pointer can be used to access the array elements outside the function scope.
A static array in C/C++ is an array whose memory is allocated at compile time, persists throughout the program’s execution, and has a fixed size that cannot be changed during runtime. Leveraging static arrays allows us to return a pointer to the array from within a function, enabling access to the array elements outside the function scope.
The approach involves declaring an array with the static
keyword inside a function. This array’s scope extends beyond the function’s execution, making it accessible externally through a returned pointer.
Let’s consider an example demonstrating the utilization of a function to return a static array:
// Function to return a static array
int* returnArrayStatic() {
static int arr[5] = {1, 2, 3, 4, 5};
return arr;
}
void setup() {
Serial.begin(9600);
// Call the function and get the array
int* returnedArray = returnArrayStatic();
// Print the elements of the returned array
for (int i = 0; i < 5; i++) {
Serial.print(returnedArray[i]);
Serial.print(" ");
}
}
void loop() {}
In this example, the returnArrayStatic()
function creates a static integer array arr
containing five elements initialized with values from 1
to 5
. The static
keyword ensures that the array persists beyond the function’s scope.
The same function returns a pointer to the static array arr
.
In the setup()
function, the returned array pointer is obtained by calling returnArrayStatic()
. The elements of the returned array are then printed using a for
loop.
Output:
1 2 3 4 5
Use Dynamic Memory Allocation to Return Array From Function in Arduino
Dynamic memory allocation refers to the process of allocating memory during program execution rather than during compile time.
In C/C++, malloc
and free
functions from the stdlib.h
library are commonly used for dynamic memory allocation. Arduino, being based on C/C++, allows the use of these functions for dynamic memory management.
The malloc
function allocates a block of memory of a specified size and returns a pointer to the first byte of the allocated memory block. The function allocates memory using malloc
and populates the array elements accordingly to return an array from a function in Arduino using dynamic memory allocation.
malloc
Syntax:
#include <stdlib.h> // For C
// or
#include <cstdlib> // For C++
// Allocate memory for a single element or an array
void* malloc(size_t size);
malloc
: This allocates a block of memory ofsize
bytes and returns a pointer to the first byte of the allocated memory block.size
: This specifies the size (in bytes) of the memory block to be allocated.
Example:
// Function to return a dynamically allocated array
int* returnArrayDynamic(int size) {
int* arr = (int*)malloc(size * sizeof(int));
// Populate the array elements
for (int i = 0; i < size; i++) {
arr[i] = i + 1;
}
return arr;
}
void setup() {
Serial.begin(9600);
// Call the function and get the array
int* returnedArray = returnArrayDynamic(5);
// Print the elements of the returned array
for (int i = 0; i < 5; i++) {
Serial.print(returnedArray[i]);
Serial.print(" ");
}
// Free dynamically allocated memory
free(returnedArray);
}
void loop() {}
The code defines a function returnArrayDynamic
in Arduino, allocating memory for an integer array based on a specified size using malloc
. The allocated array is populated with values incrementing from 1
to size
.
In the setup()
function, the code calls returnArrayDynamic(5)
to create an array of size 5
, prints its elements via serial communication, and frees the dynamically allocated memory using free()
to manage memory efficiently and prevent leaks.
Output:
1 2 3 4 5
The code exemplifies dynamic memory allocation, array initialization, retrieval, and proper memory deallocation within an Arduino sketch.
Use Static Global Arrays to Return Array From Function in Arduino
Static global arrays in Arduino sketches are declared outside of any function, making them accessible globally throughout the sketch. By utilizing these arrays within functions, modifications made to the array elements persist after the function’s execution, enabling indirect access to the modified array outside the function.
Let’s consider an example showcasing the use of a static global array and a function to modify this array’s elements:
// Declare a global array
int globalArray[5];
// Function to modify the global array
void modifyGlobalArray() {
for (int i = 0; i < 5; i++) {
globalArray[i] = i + 1;
}
}
void setup() {
Serial.begin(9600);
// Call the function to modify the global array
modifyGlobalArray();
// Print the elements of the modified global array
for (int i = 0; i < 5; i++) {
Serial.print(globalArray[i]);
Serial.print(" ");
}
}
void loop() {}
Output:
1 2 3 4 5
In this example, the globalArray
is declared outside any function, making it accessible globally within the sketch.
The modifyGlobalArray
function iterates through the globalArray
and modifies its elements. In this case, the function assigns consecutive numbers from 1
to 5
to the array elements.
Inside the setup()
function, modifyGlobalArray()
is called to modify the globalArray
. Subsequently, the elements of the modified array are printed to the serial monitor for verification.
As the globalArray
is modified within the modifyGlobalArray()
function, these changes persist after the function completes its execution. The modified array can be accessed and utilized throughout the sketch.
Use Reference Parameters to Return Array From Function in Arduino
In C/C++, passing an array to a function usually involves passing a pointer to the array’s first element. However, using reference parameters (&
) enables functions to manipulate the original array directly, avoiding the need to return the modified array explicitly.
Consider a scenario where you want to modify an array within a function and retain those modifications outside the function scope. Here’s an example demonstrating how reference parameters facilitate this in Arduino:
// Function to modify the array using reference parameter
void modifyArray(int (&arr)[5]) {
for (int i = 0; i < 5; i++) {
arr[i] = i + 1;
}
}
void setup() {
Serial.begin(9600);
int arr[5];
// Call the function to modify the array
modifyArray(arr);
// Print the elements of the modified array
for (int i = 0; i < 5; i++) {
Serial.print(arr[i]);
Serial.print(" ");
}
}
void loop() {}
Output:
1 2 3 4 5
In this example, the modifyArray
is a function that accepts an array of integers as a reference parameter. Inside this function, a for
loop modifies the elements of the array, assigning values from 1
to 5
.
The setup()
function in the Arduino sketch initializes the Serial communication and creates an integer array arr
. Inside the setup()
function, modifyArray()
is called, passing arr
as an argument.
The modified elements of arr
are then printed using Serial communication.
Conclusion
The article delves into various techniques for returning arrays from functions in Arduino, addressing the challenge posed by C/C++ limitations in passing and returning arrays by value.
The methods covered include:
- Modifying Existing Arrays: Changing array values within functions rather than returning the entire array.
- Using Static Arrays: Returning a pointer to a statically declared array to access elements outside the function’s scope.
- Dynamic Memory Allocation: Allocating and returning arrays with variable sizes during program execution.
- Static Global Arrays: Modifying arrays within functions, retaining changes outside the function’s scope.
- Reference Parameters: Directly manipulating arrays within functions using reference parameters.
Each method is exemplified through detailed code snippets, showcasing its application in Arduino sketches. These diverse approaches offer programmers versatile strategies to manage arrays effectively within Arduino environments, catering to different programming needs and scenarios.