How to Generate Random Numbers in Arduino
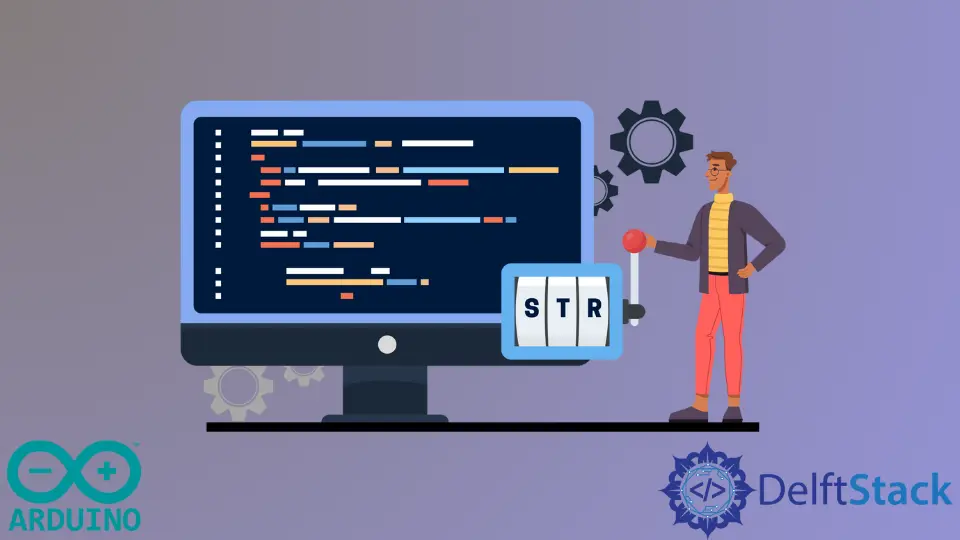
Generating random numbers is a fundamental skill in programming, especially when working with microcontrollers like Arduino. Whether you’re creating games, simulations, or any project that requires unpredictability, the ability to generate random numbers can be a game-changer. In Arduino, the random()
function is the key to unlocking this capability.
In this article, we’ll explore how to effectively use this function to generate random numbers, including various methods and practical examples. By the end, you’ll have a solid understanding of how to incorporate randomness into your Arduino projects.
Understanding the random()
Function
The random()
function in Arduino is designed to generate random numbers. It can be used in two primary ways: to generate a random number within a specified range or to generate a random number without any constraints. Understanding how to use this function effectively can enhance your projects significantly.
Generating a Random Number Without Limits
To generate a random number without any specified limits, you can simply call the random()
function without any parameters. This will return a random number between 0 and a large upper limit, typically defined by the system’s maximum integer size.
Here’s how you can implement this in an Arduino sketch:
void setup() {
Serial.begin(9600);
long randomNumber = random();
Serial.println(randomNumber);
}
void loop() {
// Do nothing here
}
Output:
123456789
In this example, the setup()
function initializes the serial communication at a baud rate of 9600. We then call random()
to generate a random number and print it to the Serial Monitor. The loop()
function is empty because we only want to generate the number once. You can see that the output is a random number, which can change every time you upload the sketch or reset the Arduino.
Generating a Random Number Within a Range
Sometimes, you may want to generate a random number within a specific range. The random(min, max)
function allows you to specify the lower and upper bounds for the random number generation. The generated number will be greater than or equal to min
and less than max
.
Here’s how you can implement this:
void setup() {
Serial.begin(9600);
long randomNumber = random(10, 100);
Serial.println(randomNumber);
}
void loop() {
// Do nothing here
}
Output:
45
In this example, we specify a range between 10 and 100. The random()
function will return a random number within that range. Each time you run the sketch, you could get a different number, providing the unpredictability needed for many applications.
Seeding the Random Number Generator
By default, the random number generator in Arduino produces the same sequence of numbers every time you reset or power the board. To prevent this and ensure that you get different sequences, you can seed the random number generator using the randomSeed()
function. This function typically takes an input from an analog pin, which can be influenced by noise, making it a good source for seeding. Additionally, if you are working with variables, knowing how to define a global variable in Arduino can be beneficial in managing your project’s complexity.
Here’s how you can do it:
void setup() {
Serial.begin(9600);
randomSeed(analogRead(0));
long randomNumber = random(10, 100);
Serial.println(randomNumber);
}
void loop() {
// Do nothing here
}
Output:
67
In this example, we use analogRead(0)
to read a random value from analog pin 0, which is then used to seed the random number generator. This way, every time the Arduino starts up, it will produce a different sequence of random numbers based on the noise from the analog input. This is particularly useful for projects where true randomness is essential.
Conclusion
Generating random numbers in Arduino is straightforward and can significantly enhance your projects. By utilizing the random()
function, you can create unpredictability in your applications, whether for games, simulations, or other creative endeavors. Remember to use randomSeed()
if you want to ensure that your sequences are different each time you run your program. If your project requires converting data types, such as needing to convert an integer to a string, understanding these operations is crucial. With these techniques, you’re well on your way to mastering randomness in Arduino.
FAQ
-
What is the range of numbers generated by the
random()
function?
The range depends on how you use the function. Without parameters, it generates numbers from 0 to a large upper limit. With parameters, it generates numbers between the specified minimum and maximum values. -
How do I ensure different random numbers each time I run my program?
Use therandomSeed()
function to seed the random number generator with a variable input, such as an analog reading, to ensure different sequences. -
Can I generate floating-point random numbers in Arduino?
No, therandom()
function only generates integers. If you need floating-point numbers, you can convert the integers to floats after generating them. -
Is there a limit to the number of random numbers I can generate in a single sketch?
No, you can call therandom()
function as many times as needed within your sketch. -
Can I use random numbers for timing events in Arduino?
Yes, you can use random numbers to create delays or intervals in your program, adding unpredictability to your timing events.