How to Define a Global Variable in Arduino
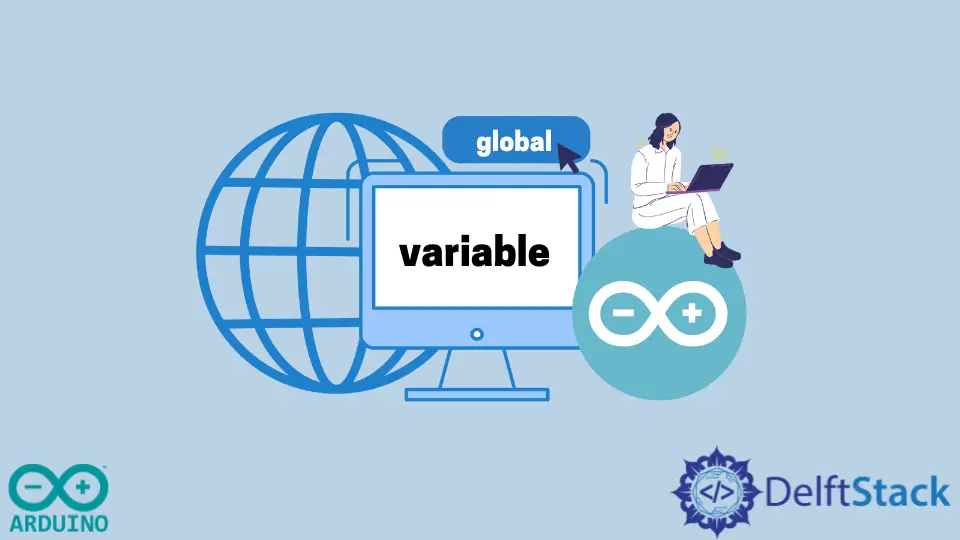
This tutorial will discuss defining a variable as global in Arduino by initializing it at the top of all other functions.
Define a Global Variable in Arduino
In Arduino, we can define different kinds of variables. The global variable is a variable that can be accessed inside any function in the code.
If we define a variable inside a function, the variable will only be used in the scope of that function. If we try to use it inside another function, Arduino will give an error saying that the variable is not defined in this scope.
If we define a variable outside a function and at the top of all the functions present inside the Arduino code, it will be a global variable that can be accessed inside any function. If the global variable is not defined as constant, we can also change its value at any point inside a function.
For example, let us define a global variable and change its value inside the setup()
function.
Example code:
int global = 10;
void setup() {
global = 20;
Serial.begin(9600);
Serial.println(global);
}
void loop() {}
Output:
20
In the above example code, we defined the variable global
and initialized it with a value of 10
. We used the serial monitor of Arduino to display the value on the serial monitor window.
The Serial.begin()
function initialized the serial monitor with the given baud
rate or speed. And the Serial.println()
function is used to print the given value to the serial monitor window.
Suppose we define the variable below the setup()
function. In that case, we will be unable to use it inside the setup()
function, but we will be able to use it inside the loop()
function or any other function defined below the global
variable.
Note that global variables can be changed from the scope of any function, so use them with caution.
If we do not want to define a global variable but want to declare it inside a function and use it inside other functions, we can pass the variables as input arguments to that function.
For example, let us define two variables inside the setup()
function, add them inside another function, return the result to the setup()
function, and print it on the serial monitor.
Example code:
void setup() {
int a = 10;
int b = 20;
int c = add(a, b);
Serial.begin(9600);
Serial.println(c);
}
void loop() {}
int add(int aa, int bb) {
int result = aa + bb;
return result;
}
Output:
30
In the above code, we defined two integer variables, a
and b
, passed them inside the add()
function to find their summation, returned the result and saved it inside the c
variable. In this example, we are not changing the original variables; we are just using their value inside another function.