How to Make a Counter in Arduino
- Understanding the Basics of Arduino Counters
- Setting Up the Arduino Environment
- Displaying the Counter on an LED
- Resetting the Counter
- Conclusion
- FAQ
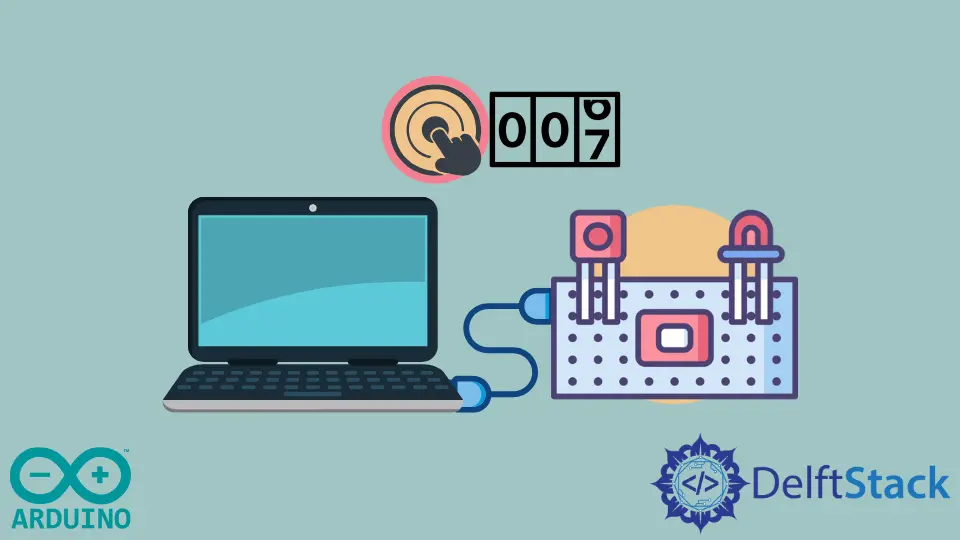
Creating a counter using Arduino is an exciting project that can help you understand the fundamentals of programming and electronics. Whether you are a novice or an experienced maker, setting up a counter can be both fun and educational.
In this article, we will explore how to create a simple counter using Arduino loops. You will learn how to increment a value, display it on an LED or serial monitor, and even reset it based on specific conditions. By the end of this guide, you will have a solid grasp of how to implement a counter in your Arduino projects. Let’s dive in!
Understanding the Basics of Arduino Counters
Before we jump into the code, let’s break down what a counter does. In simple terms, a counter is a variable that keeps track of a numerical value, incrementing or decrementing it based on specific inputs or conditions. In Arduino, counters are often used in loops to create dynamic and interactive applications.
For our example, we will create a basic counter that increments a value each time a button is pressed. This will involve using loops and conditional statements to manage the counter’s behavior. Now, let’s get started with the code.
Setting Up the Arduino Environment
First things first, you need to set up your Arduino environment. Make sure you have the Arduino IDE installed on your computer. Once you have that ready, you can start writing your code.
Here’s a simple example of a counter that increments every time a button is pressed:
const int buttonPin = 2;
int counter = 0;
void setup() {
pinMode(buttonPin, INPUT);
Serial.begin(9600);
}
void loop() {
if (digitalRead(buttonPin) == HIGH) {
counter++;
Serial.println(counter);
delay(500);
}
}
Output:
1
2
3
...
In this code, we define a button pin and initialize a counter variable. In the setup()
function, we set the button pin as an input and start the serial communication. The loop()
function continuously checks if the button is pressed. When it is, the counter increments by one, and the new value is printed to the Serial Monitor.
This simple counter program demonstrates how to use loops and conditions effectively in Arduino. The delay function prevents the counter from incrementing too rapidly, ensuring that each button press is registered clearly.
Displaying the Counter on an LED
Now that we have a basic counter, let’s enhance it by displaying the counter value on an LED. This can be achieved using a 7-segment display or a simple LED setup. For simplicity, we will use an LED to indicate when the counter increments.
Here’s how you can modify the previous code:
const int buttonPin = 2;
const int ledPin = 13;
int counter = 0;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
if (digitalRead(buttonPin) == HIGH) {
counter++;
Serial.println(counter);
digitalWrite(ledPin, HIGH);
delay(500);
digitalWrite(ledPin, LOW);
}
}
Output:
1
2
3
...
In this version, we add an LED pin that turns on each time the counter increments. The digitalWrite()
function is used to control the LED, providing a visual cue for each button press. This modification not only makes the project more interactive but also helps you understand how to control multiple outputs in Arduino.
Resetting the Counter
In many applications, you may want to reset the counter to zero after it reaches a certain value or upon a specific button press. This can be easily achieved by adding another button or using a condition to reset the counter.
Here’s an updated version of the code that resets the counter when it reaches 10:
const int buttonPin = 2;
const int resetPin = 3;
const int ledPin = 13;
int counter = 0;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(resetPin, INPUT);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
if (digitalRead(buttonPin) == HIGH) {
counter++;
Serial.println(counter);
digitalWrite(ledPin, HIGH);
delay(500);
digitalWrite(ledPin, LOW);
}
if (counter >= 10) {
counter = 0;
Serial.println("Counter reset");
}
}
Output:
1
2
3
...
Counter reset
In this code, we introduce a reset condition. If the counter reaches 10, it resets to zero, and a message is printed to the Serial Monitor. This feature is useful for applications where you need to track counts over a specific range, making your project more versatile.
Conclusion
Creating a counter in Arduino is a fantastic way to get hands-on experience with programming and electronics. By utilizing loops, conditional statements, and various outputs, you can design interactive projects that respond to user inputs. Whether you’re just starting out or looking to refine your skills, building a counter is a rewarding endeavor. With the knowledge gained from this article, you can expand your projects further, integrating more complex features and functionalities. Happy coding!
FAQ
-
What components do I need to create a counter in Arduino?
You will need an Arduino board, a push button, an LED, and some jumper wires. -
Can I use a different type of display instead of an LED?
Yes, you can use a 7-segment display or an LCD to show the counter value. -
How do I reset the counter when it reaches a specific value?
You can add a condition in your code to reset the counter when it reaches that value. -
Is it possible to create a counter that decrements instead of increments?
Yes, you can modify the code to decrease the counter value based on button presses. -
Can I use multiple buttons for different functions in my counter?
Absolutely! You can add more buttons and define different actions for each one in your code.