Arduino Int to Char
-
Convert an
int
to achar
Using the Assignment Operator in Arduino -
Convert an
int
to achar
Using theitoa
Function in Arduino -
Convert an
int
to achar
Using thesprintf
in Arduino - Conclusion
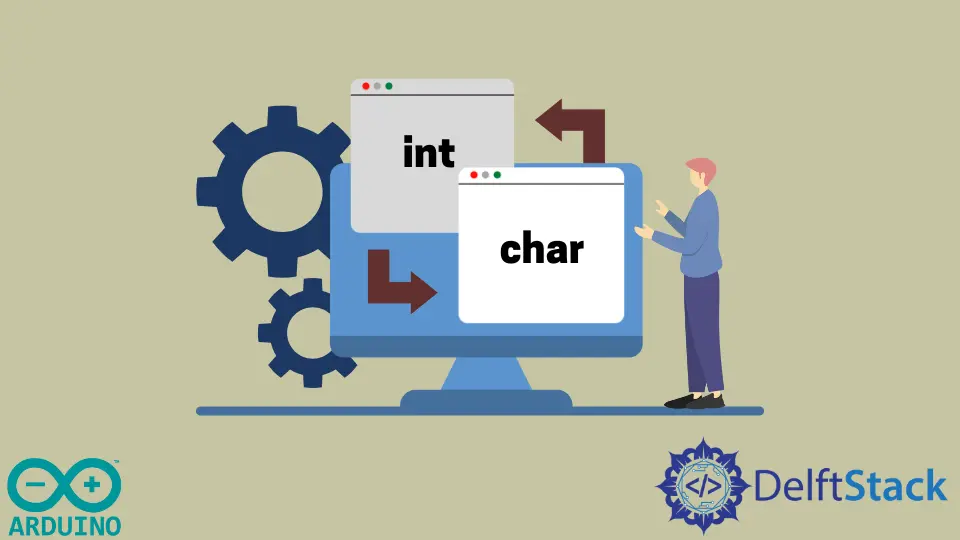
Often, when working with Arduino, you may need to convert data types to achieve specific functionality. One common conversion task is converting an int
(integer) to a char
(character) or a String
.
This can be essential when you want to display numerical data on an LCD screen or send it over a communication protocol like UART or SPI.
In this article, we will explore various methods to convert an int
to a char
in Arduino.
Convert an int
to a char
Using the Assignment Operator in Arduino
The assignment operator, represented by the equal sign (=
), is a fundamental concept in programming. It is used to assign a value to a variable.
When converting an int
to a char
, we can take advantage of this operator to achieve the desired result.
Here’s how to convert an int
to a char
in Arduino using the assignment operator:
First, declare an int
variable to hold the integer value you want to convert.
int myInt = 97; // Replace 97 with your desired integer value
Then, declare a char
variable to store the converted value. Ensure it is big enough to accommodate the expected result.
In this example, we’ll use a char
array (a character array) to store the converted value.
char charArray[6]; // Make sure it is large enough to hold the converted value
Next, use the assignment operator to convert the int
to a char
. You can do this by simply assigning the int
variable to the char
variable.
charArray = myInt;
However, you’ll notice that this code will result in an error because you can’t directly assign an int
to a char
array using the assignment operator. To work around this, you can use a typecast.
To perform the conversion, typecast the int
to a char
. This explicitly tells the compiler to treat the int
as a char
while assigning it to the char
variable.
charArray = (char)myInt;
Now, you have successfully converted the int
to a char
, and you can use the charArray
variable as needed.
Here’s the complete code:
int myInt = 97; // Replace 97 with your desired integer value
char charArray[6]; // Make sure it is large enough to hold the converted value
charArray = (char)myInt; // Convert the int to a char
// Now you can use the charArray as a char with the converted value
Remember to adjust the size of your char
array according to the largest value you expect to convert, and ensure that the converted value fits within the specified range for a char
.
Convert an int
to a char
Using the itoa
Function in Arduino
The itoa
function is a handy utility available in Arduino that stands for “integer to ASCII”. It allows you to convert an int
to a character array, also known as a string, making it suitable for a wide range of applications.
Here’s how you can use the itoa
function to convert an int
to a char[]
:
itoa(integer, charArray, base);
integer
: Theint
variable you want to convert.charArray
: The character array where the converted string will be stored.base
: The numeric base (e.g.,10
for decimal,16
for hexadecimal).
Now, let’s discuss how to use the itoa
function to perform the conversion.
Start by declaring an int
variable to hold the integer value you wish to convert. Replace 42
with your desired integer.
int myInt = 42;
Then, create a character array (char
array) to store the converted string. Make sure to size it appropriately to accommodate the expected result.
In this example, we’ll use a char
array of 6
characters. It’s essential to allocate enough space for the converted string, considering the length of your integer and the base you choose.
char charArray[6];
Next, use the itoa
function to convert the int
to a character array. Pass the myInt
variable, the charArray
where the result should be stored, and the numeric base you want (usually 10
for decimal).
itoa(myInt, charArray, 10);
You’ve successfully converted the int
to a character array using the itoa
function, and you can now utilize the charArray
as a character string.
Here’s the complete code:
int myInt = 42; // Replace 42 with your desired integer value
char charArray[6]; // Make sure it is large enough to hold the converted value
itoa(myInt, charArray, 10); // Convert the int to a char array
// Now you can use charArray as a char with the converted value
This method simplifies the process of converting an int
to a character array in Arduino, making it particularly useful for projects where you need to work with numerical data in a string format. Just remember to adjust the size of your char
array based on the expected range of values and the desired numeric base.
Convert an int
to a char
Using the sprintf
in Arduino
sprintf
is a powerful C/C++ function that allows you to format and store data in a character array. It’s commonly used for creating strings by combining different data types, including integers, floats, and characters.
In the context of Arduino, sprintf
is handy for converting an int
to a character array.
Here’s the basic syntax for using sprintf
to convert an int
to a char[]
:
sprintf(charArray, format, integer);
charArray
: The character array where the converted string will be stored.format
: A format string that defines how the integer should be converted.integer
: Theint
variable you want to convert.
To use sprintf
to convert an int
to a char[]
in Arduino, begin by declaring an int
variable to hold the integer value you want to convert. Replace 42
with your desired integer.
int myInt = 42;
Next, create a character array (char
array) to store the converted string. Make sure to size it appropriately to accommodate the expected result.
In this example, we’ll use a char
array of 6
characters. It’s essential to allocate enough space for the converted string, considering the length of your integer and the format you choose.
char charArray[6];
Then, use the sprintf
function to convert the int
to a character array. Pass the charArray
where the result should be stored, the format string, and the myInt
variable.
For converting an int
to a decimal string, use the format string %d
. The %d
format specifier indicates that you are converting an integer to a decimal string.
sprintf(charArray, "%d", myInt);
You’ve now successfully converted the int
to a character array using sprintf
. The charArray
contains the formatted string representation of your integer, which you can use in your Arduino project.
Here’s the complete code:
int myInt = 42; // Replace 42 with your desired integer value
char charArray[6]; // Make sure it is large enough to hold the converted value
sprintf(charArray, "%d", myInt); // Convert the int to a char array
// Now you can use charArray as a character array with the converted value
Using sprintf
to convert an int
to a character array in Arduino is a versatile and robust method, particularly useful when you need to format and manipulate numerical data for various applications. Remember to adjust the size of your char
array based on the expected range of values and the chosen format specifier, and you’ll be able to work with integer values in character format effectively.
Conclusion
In this article, we explored three methods for converting an int
to a char
in Arduino: using the assignment operator, the itoa
function, and the sprintf
function. Each method offers its unique advantages and can be useful in different scenarios.
Using the assignment operator allows for a simple and direct conversion, but it requires typecasting the int
to char
to avoid errors. The itoa
function streamlines the conversion process, making it easy to convert an int
to a character array, and it’s particularly handy when you need to work with numerical data in a string format.
Finally, the sprintf
function provides powerful formatting capabilities, enabling you to create custom representations of your integer values in a character array.
When choosing a method for your Arduino project, consider the specific requirements of your application. Ensure that the size of your char
array is sufficient to hold the converted value, and use the appropriate format specifier with sprintf
to format the string according to your needs.
These conversion methods give you the flexibility to work with numerical data in character format, enhancing your ability to display, communicate, and manipulate data in your Arduino projects.