How to Check if a Variable Is a String in TypeScript
- TypeScript Type Declarations
-
How to Check if a Variable Is a String in TypeScript Using the
typeof
Operator -
How to Check if a Variable Is a String in TypeScript Using the
instanceof
Operator -
How to Check if a Variable Is a String in TypeScript Using
Object.prototype
-
How to Check if a Variable Is a String in TypeScript Using Type Guard With
typeof
-
How to Check if a Variable Is a String in TypeScript Using Type Guard With
instanceof
-
How to Check if a Variable Is a String in TypeScript Using
as
Type Assertion - Conclusion
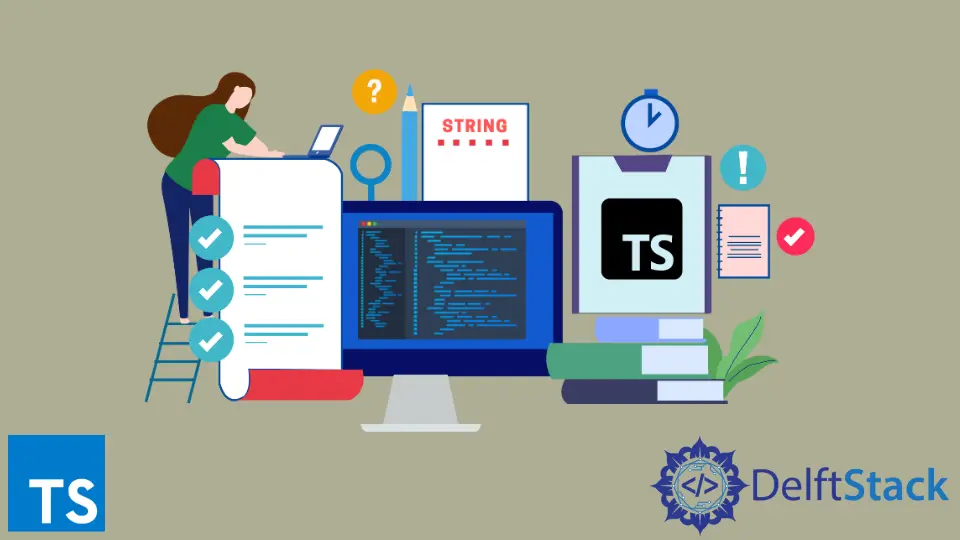
In TypeScript, a statically-typed superset of JavaScript, ensuring the correct types of variables is crucial for maintaining code integrity and preventing unexpected runtime errors. One common scenario developers encounter is TypeScript type checking to verify if a variable is of string type.
In this article, we’ll explore various approaches to tackle this task, each method providing a unique perspective on how to determine if a variable is indeed a string in TypeScript.
From the straightforward use of the typeof
operator to the versatility of Type Guards with instanceof
, we’ll delve into practical examples and explanations to equip you with the knowledge needed to make informed decisions when verifying string types.
TypeScript Type Declarations
In TypeScript, we have the capability to explicitly designate types for variables, functions, and arguments during their declaration, facilitating advanced error detection. For example:
let stringTypeVariable: string = 'Hello world';
let booleanTypeVariable: boolean = true;
let numberTypeVariable: number = 10;
function calculateSum(numOne: number, numTwo: number): number {
return numOne + numTwo;
}
String Type in TypeScript
In the TypeScript language, the string type signifies a grouping of characters enclosed within either single or double quotation marks. This data type can be stored in two distinct manners: as a primitive data type or as an object instance.
Storing String as a Primitive Type
For holding string literals, it is recommended to utilize primitive strings:
let stringTypeName: string = 'John Doe';
let stringTypeId: string = "User001";
By adhering to primitive string types, we prioritize a clean and direct approach to handling string literals, reinforcing the essence of simplicity in their code.
Storing String as an Object Instance
TypeScript extends its support to String
object instances, enveloping the primitive string type with additional utility methods:
let stringVal: String = new String('This is a String object');
let anotherStrVal: String = String('Another String object');
To ascertain whether a variable contains a string, various methods can be employed, including the typeof
operator, the instanceof
operator, and the Object.prototype
property.
How to Check if a Variable Is a String in TypeScript Using the typeof
Operator
One straightforward method to determine whether a variable is a string is by utilizing the typeof
operator. The typeof
operator in TypeScript is a unary operator that returns a string representing the type of its operand. When applied to a variable, it helps identify the data type of that variable.
The syntax of the typeof
operator in TypeScript is quite simple and resembles its usage in JavaScript. Its general syntax is as follows:
typeof operand
Here, operand
is the expression or variable for which you want to determine the type. The typeof
operator returns a string representing the data type of the operand.
In the context of checking if a variable is a string, you would typically use it in a conditional statement like this:
if (typeof myVariable === 'string') {
// Code to handle the case where myVariable is a string
} else {
// Code to handle the case where myVariable is not a string
}
In this example, myVariable
is the operand, and the typeof
operator is used to check if its type is equal to the string literal 'string'
.
Check if a Variable Is a String Using typeof
Example
Let’s dive into a complete working example to illustrate how to use the typeof
operator to check if a variable is a string in TypeScript:
// Declare a variable of type string
let myVariable: string = 'John Doe';
// Check typeof string
if (typeof myVariable === 'string') {
console.log('myVariable is a string.');
} else {
console.log('myVariable is not a string.');
}
In the above example, we declare a variable myVariable
and assign it the string value 'John Doe'
. Following that, we use an if
statement with the typeof
operator to check if the data type of myVariable
is equal to the string literal 'string'
.
If the condition evaluates to true
, it means that myVariable
is indeed a string, and the code inside the if
block will be executed. Otherwise, the else
block will be executed, indicating that myVariable
is not a string.
Code Output
If myVariable
is not a string, the output will be:
This output demonstrates that the typeof
operator successfully identified myVariable
as a string, triggering the corresponding logic within the if
block. This approach provides a concise and effective way to check for string types in TypeScript.
How to Check if a Variable Is a String in TypeScript Using the instanceof
Operator
The instanceof
operator is another powerful tool for type checking in TypeScript, especially when dealing with object instances. The instanceof
operator checks whether an object is an instance of a particular class or constructor.
Its syntax is straightforward and also closely resembles its usage in JavaScript:
object instanceof constructor
Here, object
is the object instance you want to check, and constructor
is the constructor function or class that you want to check whether object is an instance of. The instanceof
operator returns a boolean value (true
or false
) based on whether the object is an instance of the specified constructor.
In the context of checking if a variable is a string, you would typically use it in a conditional statement like this:
if (myVariable instanceof String) {
// Code to handle the case where myVariable is a String object
} else {
// Code to handle the case where myVariable is not a String object
}
Here, myVariable
is the object you want to check, and String
is the constructor for the String
object.
Check if a Variable Is a String Using instanceof
Example
Let’s explore a complete code example to illustrate how to use the instanceof
operator to check if a variable is a string in TypeScript:
// Declare a String object instance
let stringObject: String = new String('This is a String object');
// TypeScript check if string using the instanceof operator
console.log('stringObject is a String object.');
} else {
console.log('stringObject is not a String object.');
}
In this example, we declare a variable stringObject
and assign it a new instance of the String
object with the value 'This is a String object'
. We then use an if
statement with the instanceof
operator to check if stringObject
is an instance of the String
object.
If the condition evaluates to the boolean value true
, it means that stringObject
is indeed a String
object, and the code inside the if
block will be executed. Otherwise, the else
block will be executed, indicating that stringObject
is not a String
object.
Code Output
This output demonstrates that the instanceof
operator successfully identified stringObject
as an instance of the String
object, triggering the corresponding logic within the if
block. Using the instanceof
operator provides a robust way to check for string types, especially when dealing with String
object instances in TypeScript.
How to Check if a Variable Is a String in TypeScript Using Object.prototype
The Object.prototype
property provides another versatile approach to identifying variable types. When it comes to checking if a variable is a string, we can leverage the Object.prototype.toString
method along with the call
method to examine the variable’s prototype and ascertain its type.
The syntax to use Object.prototype
to check the data type of a variable involves calling the toString
method on it and then using the call
method to apply it to the variable in question.
Here’s the general syntax:
Object.prototype.toString.call(variable)
In this syntax:
Object.prototype
refers to the prototype object for all objects.toString
is the method that returns a string representation of the object.call(variable)
is used to invoke thetoString
method on the specified variable.
When specifically checking if a variable is a string, you would typically compare the result to the expected string representation for strings, which is '[object String]'
. Here’s an example:
if (Object.prototype.toString.call(myVariable) === '[object String]') {
// Code for when the variable is a string
} else {
// Code for when the variable is not a string
}
This comparison ensures that the myVariable
is of type string by checking the string representation produced by Object.prototype.toString.call
.
Check if a Variable Is a String Using Object.prototype
Example
Let’s delve into a complete working example to demonstrate how to use Object.prototype
to check if a variable is a string in TypeScript:
// Declare a variable of string data type
let myVariable: string = 'Hello, TypeScript!';
// Use Object.prototype.toString to check if the variable is a string
if (Object.prototype.toString.call(myVariable) === '[object String]') {
console.log('myVariable is a string.');
} else {
console.log('myVariable is not a string.');
}
In the above code example, we declare a given variable myVariable
and assign it the string value 'Hello, TypeScript!'
. We then use an if
statement with Object.prototype.toString.call
to check if the result of calling toString
on myVariable
matches the expected string '[object String]'
.
If the condition evaluates to true
, it indicates that myVariable
is indeed a string, and the code inside the if
block will be executed. Otherwise, the else
block will be executed, indicating that myVariable
is not a string.
Code Output
This output demonstrates that using Object.prototype
successfully identified myVariable
as a string, triggering the corresponding logic within the if
block. Employing Object.prototype
offers a generic and reliable way to check for string types in TypeScript.
How to Check if a Variable Is a String in TypeScript Using Type Guard With typeof
When it comes to checking if a given variable is a string, a custom type guard function utilizing the typeof
operator is a useful approach. This allows for a more modular and reusable way to ascertain the type of a variable.
A type guard in TypeScript is a user-defined function whose return type is a type predicate. In this scenario, we create a type guard function that utilizes the typeof
operator to explicitly check if the provided variable is of type string.
If the type guard returns true, TypeScript narrows down the variable type to a string within the corresponding code block.
Check if a Variable Is a String Using Type Guard With typeof
Example
Let’s take a look at a complete working example:
// Define a type guard function for strings
function isString(value: any): value is string {
return typeof value === 'string';
}
// Declare a variable of type string
let myVariable: string = 'Hello, TypeScript!';
// Use the type guard function to check if the variable is a string
if (isString(myVariable)) {
console.log('myVariable is a string.');
} else {
console.log('myVariable is not a string.');
}
In the above code example, we define a custom type guard function named isString
. This function takes a parameter value
of type any
, indicating that it can accept a variable of any type.
Inside the function, the line return typeof value === 'string';
checks if the typeof
the provided value
is equal to the string literal 'string'
. The return type of this function is specified as value is string
, which is a type predicate indicating that if the function returns true
, TypeScript should narrow down the type of the variable to string
.
After defining the type guard function, we declare a variable named myVariable
of type string and assign it the value 'Hello, TypeScript!'
. Subsequently, we use an if
statement to invoke the isString
type guard function, passing myVariable
as an argument.
If the type guard function returns true
, it means that myVariable
is indeed a string, and the code inside the if
block will be executed. In this case, it logs the message 'myVariable is a string.'
to the console.
If the type guard function returns false
, indicating that myVariable
is not a string, the code inside the else
block will be executed, logging the message 'myVariable is not a string.'
to the console.
Code Output
This output demonstrates that the type guard function with typeof
successfully identified myVariable
as a string, triggering the corresponding logic within the if
block. Utilizing type guards enhances type safety and provides a clean and modular way to check for variable types in TypeScript.
How to Check if a Variable Is a String in TypeScript Using Type Guard With instanceof
As defined above, type guards in TypeScript allow developers to create functions that narrow down the type of a variable within a certain block of code. When using instanceof
, the type guard checks if the variable is an instance of a specific constructor function, in this case, the String
constructor.
If the type guard returns true
, TypeScript recognizes the variable as a string within the corresponding code block.
Check if a Variable Is a String Using Type Guard With instanceof
Example
Let’s explore a complete working example to illustrate type checking using Type Guard with instanceof
in TypeScript:
// Define a type guard function for strings
function isString(value: any): value is string {
return value instanceof String || typeof value === 'string';
}
// Declare a variable of type string
let myVariable: string = 'Hello, TypeScript!';
// Use the type guard function to check if the variable is a string
if (isString(myVariable)) {
console.log('myVariable is a string.');
} else {
console.log('myVariable is not a string.');
}
In the above code, we introduce another type guard function called isString
. Similar to the previous example, this function takes a parameter named value
with a type of any
, making it flexible enough to accept variables of any type.
Inside the function, the line return value instanceof String || typeof value === 'string';
performs a type check to determine whether the value
is either an instance of the String
object or if its typeof
is equal to the string literal 'string'
. The return
statement specifies the function’s return type as value is string
, indicating to TypeScript that if the function returns true
, the variable should be considered of type string.
After defining the type guard function, we declare a variable named myVariable
of type string and assign it the value 'Hello, TypeScript!'
. Following this, we use an if
statement to invoke the isString
type guard function, passing myVariable
as an argument.
If the type guard function returns true
, indicating that myVariable
is a string, the code inside the if
block will be executed. In this case, it logs the message 'myVariable is a string.'
to the console.
On the other hand, if the type guard function returns false
, suggesting that myVariable
is not a string, the code inside the else
block will be executed, logging the message, 'myVariable is not a string.'
to the console.
Code Output
This output demonstrates that the type guard function with instanceof
successfully identified myVariable
as a string, triggering the corresponding logic within the if
block. Utilizing type guards provides a robust and concise way to check for variable types in TypeScript.
How to Check if a Variable Is a String in TypeScript Using as
Type Assertion
TypeScript provides type assertions, a way for developers to tell the compiler that they have more information about a variable’s type than the compiler can infer. The as
keyword is used to perform such type assertions.
In the context of checking if a variable is a string, we can use as
to assume that a variable is of type string, bypassing the static type checking of TypeScript.
Check if a Variable Is a String Using as
Example
// Define a variable of unknown type
const myVar: any = "Hello, TypeScript!";
// Use `as` type assertion to assume the variable is a string
const isString = (typeof myVar === "string") ? myVar as string : undefined;
// Check if the variable is indeed a string
if (isString !== undefined) {
console.log("myVar is a string");
} else {
console.log("myVar is not a string.");
}
In this example, we start by declaring a variable myVar
with the type any
, indicating that its type is unknown.
We then use the typeof
operator to check if the variable is of type string. If it is, we use the as
type assertion to inform TypeScript that we are treating myVar
as a string.
The isString
variable is assigned the result of the type assertion. If myVar
is indeed a string, isString
will contain the string value; otherwise, it will be assigned undefined
.
Finally, we use a simple if
statement to check if isString
is not undefined
, indicating that myVar
is a string. The result is then logged to the console.
Code Output
If myVar
is not a string, the output will be:
This approach using the as
type assertion allows developers to selectively bypass TypeScript’s static type checking when they have additional knowledge about the variable’s type, providing flexibility while maintaining a level of type safety.
Conclusion
In conclusion, ensuring proper type handling is fundamental in TypeScript to maintain code integrity and prevent runtime errors. We have explored various approaches to check if a variable is of string type.
From the straightforward use of the typeof
operator to determine the type of a variable to the powerful instanceof
operator for checking String
object instances, each method offers a unique perspective. Additionally, leveraging Object.prototype
and type guards with both typeof
and instanceof
provides versatile and robust ways to ascertain string types.
We demonstrated practical examples for each approach, illustrating how we can effectively implement these techniques in our TypeScript projects. Whether through direct type assertions or more sophisticated type guards, understanding these methods equips us with the knowledge to make informed decisions when dealing with string types in TypeScript.
Ultimately, the choice of method depends on the specific requirements of the project and the nature of the variables being handled. By having a comprehensive understanding of these techniques, we can enhance the reliability and maintainability of our TypeScript code.
To further improve your TypeScript coding skills, a relevant next topic you can learn is Arrays in TypeScript.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.