在 TypeScript 中檢查一個變數是否是一個字串
- TypeScript 型別
-
在 TypeScript 中使用
typeof
運算子檢查變數是否為字串 -
在 TypeScript 中使用
instanceof
運算子檢查變數是否為字串 -
在 TypeScript 中使用
Object.prototype
檢查變數是否為字串
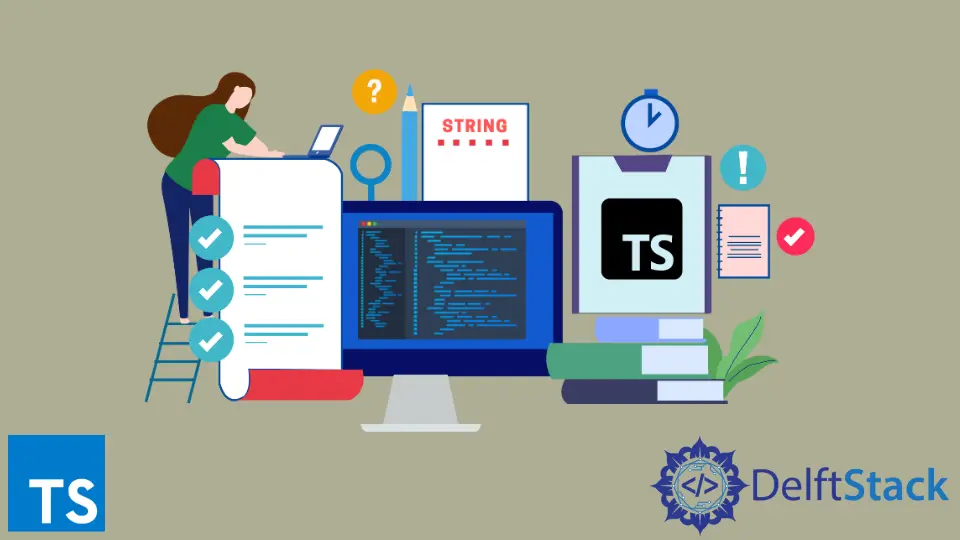
本文將討論在 TypeScript 中檢查變數是否為字串。
TypeScript 型別
TypeScript 是 JavaScript 語言的超集,型別檢查在編譯時進行。每當宣告變數、函式或引數時,TypeScript 允許我們顯式分配型別,這有助於提前識別錯誤。
// variable declaration and assignment
let stringTypeVariable: string = 'Hello world';
let booleanTypeVariable: boolean = true;
let numberTypeVariable: number = 10;
// function declaration
function calculateSum(numOne: number, numTwo: number): number {
return numOne + numTwo;
}
TypeScript 中的 string
型別
TypeScript 字串型別包含一組字元。它是一種原始資料型別,其中一個字元序列包含在單引號或雙引號之間。
文字資料可以儲存在字串型別中。有兩種方法可以在 TypeScript 中儲存字串:
- 儲存為原始型別
- 儲存為物件例項
將字串儲存為原始型別
通常,原始字串型別包含字串文字。建議使用原始字串而不是物件例項。
let stringTypeName: string = 'John Doe';
let stringTypeId: string = "User001";
將字串儲存為物件例項
TypeScript 支援 String 物件例項,它使用附加的輔助方法包裝原始字串型別。String 物件例項將在其原型鏈中具有 String.prototype
。
let stringVal: String = new String('This is a String object');
let anotherStrVal: String = String('Another String object');
有幾種方法可以檢查給定變數是否包含字串。通常,typeof
運算子用於檢查原始字串型別,instanceof
運算子可用於字串物件例項。
除此之外,Object.prototype
屬性可用於檢查給定型別是否為字串。
在 TypeScript 中使用 typeof
運算子檢查變數是否為字串
typeof
是一個 TypeScript 一元運算子,它返回指定運算元的資料型別。
語法:
typeof <operand>
運算子返回一個表示運算元型別的字串。
讓我們建立一個原始字串 userName
。
let userName: string = 'Ricky hardy';
接下來,我們將使用 typeof
運算子來檢查 userName
變數的資料型別。讓我們將它列印到控制檯,如下所示:
console.log(typeof userName);
輸出:
string
因此,typeof
運算子可用於檢查條件程式碼片段中的原始型別字串,如下所示:
if (typeof userName === 'string') {
// logic to be executed when the type is a string
} else {
// logic to be executed for non-string types
}
在 TypeScript 中使用 instanceof
運算子檢查變數是否為字串
instanceof
運算子對給定物件的原型鏈進行操作,並檢查原型屬性是否出現在那裡。它檢查指定物件是子物件還是給定建構函式的例項。
語法:
<object> instanceof <constructor/type>
該運算子返回一個布林值。此外,運算子只接受物件例項,而不接受原語。
讓我們例項化一個新的字串物件 vehicleBrand
。
let vehicleBrand: String = new String("AUDI");
接下來,我們將使用 instanceof
運算子來檢查 vehicleBrand
是否為字串型別。
console.log(vehicleBrand instanceof String);
輸出:
true
正如預期的那樣,運算子返回 true
,因為 vehicleBrand
是一個字串物件。
在 TypeScript 中使用 Object.prototype
檢查變數是否為字串
Object.prototype
也可以用作在 TypeScript 中識別字串型別的更通用的方法。該屬性包含 toString()
方法,我們可以在其中使用 call
方法並檢查指定變數原型的型別。
語法:
Object.prototype.toString.call(<variable/object>);
這應該返回以下格式的字串:
"[object String]"
OR
"[object Array]"
OR
.
.
.
讓我們建立一個新變數 newStringValue
。
let newStringValue: string = 'This is a new string';
接下來,我們將使用 Object.prototype
屬性檢查 newStringValue
變數建構函式型別。
console.log(Object.prototype.toString.call(newStringValue));
輸出:
[object String]
正如預期的那樣,型別是字串。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.