TypeScript에서 변수가 문자열인지 확인
- TypeScript 유형
-
typeof
연산자를 사용하여 TypeScript에서 변수가 문자열인지 확인 -
instanceof
연산자를 사용하여 TypeScript에서 변수가 문자열인지 확인 -
Object.prototype
을 사용하여 TypeScript에서 변수가 문자열인지 확인
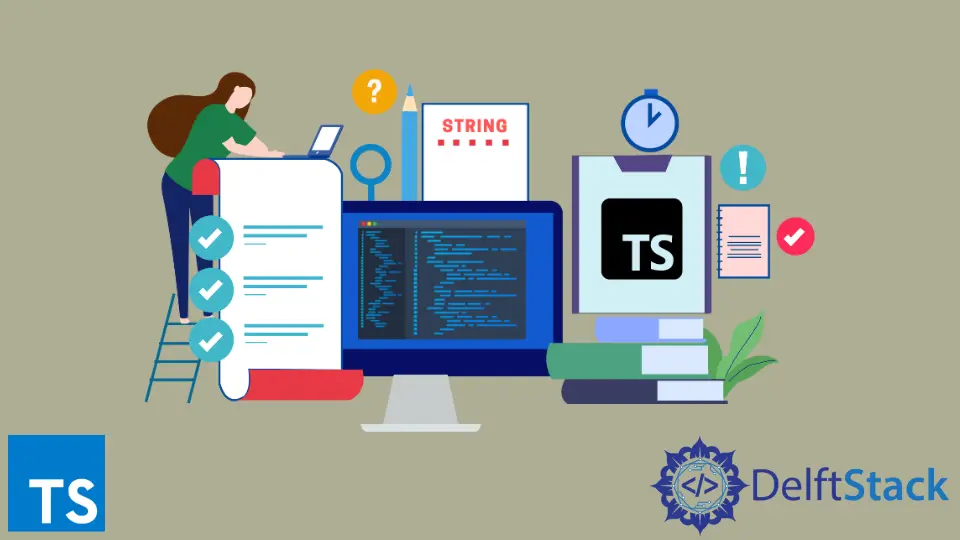
이 기사에서는 TypeScript에서 변수가 문자열인지 확인하는 방법에 대해 설명합니다.
TypeScript 유형
TypeScript는 컴파일 시간에 유형 검사가 수행되는 JavaScript 언어의 상위 집합입니다. 변수, 함수 또는 인수가 선언될 때마다 TypeScript를 사용하면 유형을 명시적으로 할당할 수 있으므로 미리 오류를 식별하는 데 도움이 됩니다.
// variable declaration and assignment
let stringTypeVariable: string = 'Hello world';
let booleanTypeVariable: boolean = true;
let numberTypeVariable: number = 10;
// function declaration
function calculateSum(numOne: number, numTwo: number): number {
return numOne + numTwo;
}
TypeScript의 문자열
유형
TypeScript 문자열 유형은 문자 모음을 보유합니다. 일련의 문자가 작은따옴표나 큰따옴표로 묶인 기본 데이터 유형입니다.
텍스트 데이터는 문자열 형식으로 저장할 수 있습니다. TypeScript에 문자열을 저장할 수 있는 두 가지 방법이 있습니다.
- 기본 유형으로 저장
- 객체 인스턴스로 저장
문자열을 기본 유형으로 저장
일반적으로 기본 문자열 유형은 문자열 리터럴을 보유합니다. 기본 문자열은 개체 인스턴스보다 권장됩니다.
let stringTypeName: string = 'John Doe';
let stringTypeId: string = "User001";
문자열을 개체 인스턴스로 저장
TypeScript는 기본 문자열 유형을 추가 도우미 메서드로 래핑하는 String 개체 인스턴스를 지원합니다. String 개체 인스턴스의 프로토타입 체인에는 String.prototype
이 있습니다.
let stringVal: String = new String('This is a String object');
let anotherStrVal: String = String('Another String object');
주어진 변수에 문자열이 있는지 확인하는 몇 가지 방법이 있습니다. 일반적으로 typeof
연산자는 기본 문자열 유형을 확인하는 데 사용되며 instanceof
연산자는 String 개체 인스턴스와 함께 사용할 수 있습니다.
그 외에 Object.prototype
속성을 사용하여 주어진 유형이 문자열인지 여부를 확인할 수 있습니다.
typeof
연산자를 사용하여 TypeScript에서 변수가 문자열인지 확인
typeof
는 지정된 피연산자의 데이터 유형을 반환하는 TypeScript 단항 연산자입니다.
통사론:
typeof <operand>
연산자는 피연산자의 유형을 나타내는 문자열을 반환합니다.
기본 문자열 userName
을 생성해 보겠습니다.
let userName: string = 'Ricky hardy';
다음으로 typeof
연산자를 사용하여 userName
변수의 데이터 유형을 확인합니다. 다음과 같이 콘솔에 인쇄해 보겠습니다.
console.log(typeof userName);
출력:
string
따라서 typeof
연산자는 다음과 같이 조건부 코드 조각 내에서 기본 유형 문자열을 확인하는 데 사용할 수 있습니다.
if (typeof userName === 'string') {
// logic to be executed when the type is a string
} else {
// logic to be executed for non-string types
}
instanceof
연산자를 사용하여 TypeScript에서 변수가 문자열인지 확인
instanceof
연산자는 주어진 객체의 프로토타입 체인에서 작동하고 프로토타입 속성이 거기에 나타나는지 확인합니다. 지정된 객체가 자식인지 지정된 생성자의 인스턴스인지 확인합니다.
통사론:
<object> instanceof <constructor/type>
이 연산자는 부울 값을 반환합니다. 또한 연산자는 기본 개체가 아닌 개체 인스턴스만 허용합니다.
새 String 개체 vehicleBrand
를 인스턴스화해 보겠습니다.
let vehicleBrand: String = new String("AUDI");
다음으로 instanceof
연산자를 사용하여 vehicleBrand
가 문자열 유형인지 확인합니다.
console.log(vehicleBrand instanceof String);
출력:
true
예상대로 vehicleBrand
는 String 개체이기 때문에 연산자는 true
를 반환합니다.
Object.prototype
을 사용하여 TypeScript에서 변수가 문자열인지 확인
Object.prototype
은 TypeScript에서 문자열 유형을 식별하는 보다 일반적인 방법으로도 사용할 수 있습니다. 이 속성에는 toString()
메서드가 포함되어 있습니다. 여기서 call
메서드를 사용하고 지정된 변수의 프로토타입 유형을 확인할 수 있습니다.
통사론:
Object.prototype.toString.call(<variable/object>);
다음 형식의 문자열을 반환해야 합니다.
"[object String]"
OR
"[object Array]"
OR
.
.
.
새로운 변수 newStringValue
를 생성해 보겠습니다.
let newStringValue: string = 'This is a new string';
다음으로 Object.prototype
속성을 사용하여 newStringValue
변수 생성자 유형을 확인합니다.
console.log(Object.prototype.toString.call(newStringValue));
출력:
[object String]
예상대로 유형은 문자열입니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.