How to Have Multiline Strings in TypeScript
- Understanding Multiline Strings
- Method 1: Using Template Literals
- Method 2: String Concatenation
- Method 3: Using Array Join
- Conclusion
- FAQ
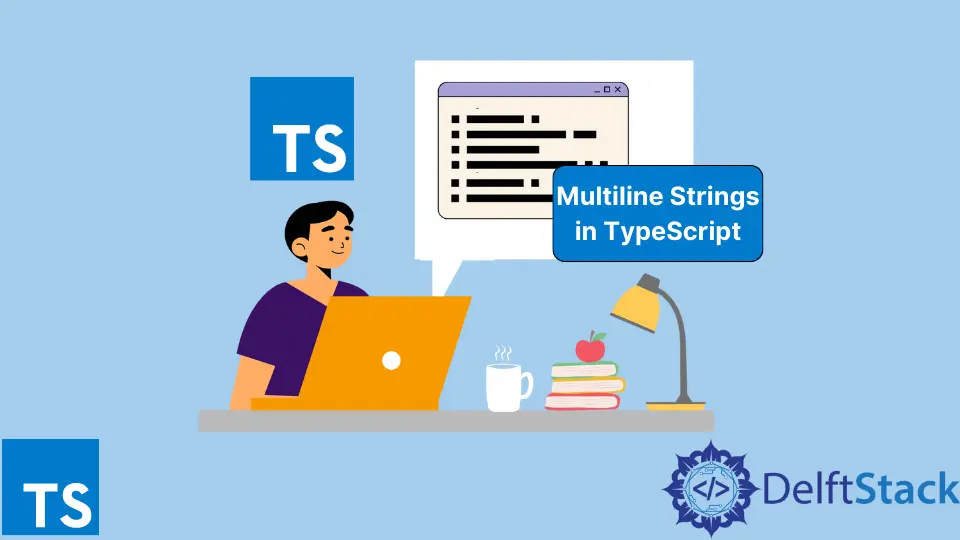
TypeScript, a superset of JavaScript, offers robust features that enhance the development experience. One such feature is the ability to work with multiline strings, which can significantly improve code readability and maintainability.
In this tutorial, we will explore various methods to create multiline strings in TypeScript, including template literals and string concatenation. Whether you’re building a web application or a Node.js service, understanding how to manage multiline strings effectively will streamline your coding process. Let’s dive into the different approaches you can take to handle multiline strings in TypeScript.
Understanding Multiline Strings
Before we delve into the methods, it’s essential to understand why multiline strings are beneficial. In many programming scenarios, especially when dealing with HTML or JSON data, you may find yourself needing to format strings over multiple lines. This not only enhances readability but also allows you to maintain the structure of your data without cumbersome escape characters. TypeScript makes this process straightforward, enabling you to focus on writing clean and efficient code.
Method 1: Using Template Literals
One of the most popular ways to create multiline strings in TypeScript is by using template literals. Template literals are enclosed by backticks (`
) and allow for string interpolation, which means you can embed expressions inside the string. This feature makes it easy to create complex strings without the need for concatenation.
Here’s how you can use template literals for multiline strings:
const multilineString = `This is a multiline string
that spans across multiple lines.
You can even include variables like this: ${2 + 2}.`;
console.log(multilineString);
Output:
This is a multiline string
that spans across multiple lines.
You can even include variables like this: 4.
Using template literals is not only concise but also intuitive. You can create strings that maintain their formatting, making your code cleaner and easier to read. Additionally, you can incorporate expressions directly within the string, which adds flexibility and dynamism to your code.
Method 2: String Concatenation
Another method to create multiline strings in TypeScript is through string concatenation. Although this approach is less modern compared to template literals, it remains a viable option, especially for those who are accustomed to traditional JavaScript practices. You can concatenate strings using the +
operator and include newline characters (\n
) to create new lines.
Here’s an example of how to use string concatenation for multiline strings:
const multilineString = "This is a multiline string\n" +
"that spans across multiple lines.\n" +
"You can also include variables like this: " + (2 + 2) + ".";
console.log(multilineString);
Output:
This is a multiline string
that spans across multiple lines.
You can also include variables like this: 4.
While string concatenation works well, it can become cumbersome, especially with longer strings. You have to manage the +
operators and ensure that newline characters are placed correctly. However, it does provide a straightforward way to build strings without the need for backticks, making it accessible for developers familiar with earlier JavaScript syntax.
Method 3: Using Array Join
A more advanced technique to create multiline strings is to use an array and the join
method. This method allows you to store each line of your string as an element in an array and then join them together with a newline character. This approach can be particularly useful when dealing with dynamic content or when you want to construct a string programmatically.
Here’s how you can implement this method:
const lines = [
"This is a multiline string",
"that spans across multiple lines.",
"You can even include variables like this: " + (2 + 2) + "."
];
const multilineString = lines.join("\n");
console.log(multilineString);
Output:
This is a multiline string
that spans across multiple lines.
You can even include variables like this: 4.
Using the array join method can enhance code organization and readability, especially when you have multiple lines of text. It allows you to define each line separately and easily manage the order of lines. This method is also beneficial when you need to build strings dynamically based on conditions or input.
Conclusion
In this tutorial, we explored various methods for handling multiline strings in TypeScript, including template literals, string concatenation, and the array join method. Each approach has its own advantages, and the best choice often depends on the specific requirements of your project. By mastering these techniques, you can write cleaner, more maintainable code that enhances both your productivity and your application’s performance. So, whether you’re crafting complex strings for HTML or simply formatting text for output, these methods will serve you well.
FAQ
-
What are template literals in TypeScript?
Template literals are string literals enclosed by backticks that allow for multiline strings and string interpolation. -
Can I use string concatenation for multiline strings in TypeScript?
Yes, you can use string concatenation with the+
operator and newline characters (\n
) to create multiline strings. -
What is the advantage of using the array join method?
The array join method allows you to manage each line of a string separately, making it easier to construct dynamic strings. -
Are there any performance differences between these methods?
Generally, the performance differences are negligible for small strings, but template literals are usually preferred for their readability and ease of use. -
Can I include variables in multiline strings?
Yes, you can include variables in multiline strings using template literals or string concatenation.