How to Check if a String Has a Certain Text in TypeScript
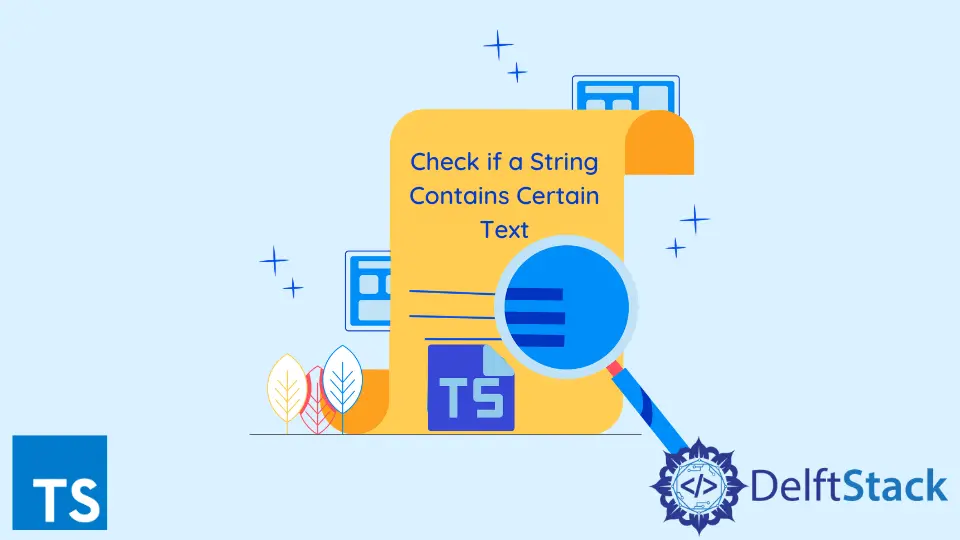
In the world of programming, string manipulation is a common task. Whether you’re validating user input, processing data, or simply searching through text, knowing how to check if a string contains a certain text in TypeScript is essential.
This tutorial will guide you through various methods to achieve this, ensuring that you can handle string checks effectively in your TypeScript projects. With clear code examples and detailed explanations, you’ll gain a solid understanding of how to work with strings in TypeScript. Let’s dive into the different methods you can use to check for specific text within strings.
Using the includes
Method
One of the most straightforward ways to check if a string contains a certain substring in TypeScript is by using the includes
method. This method returns a boolean value indicating whether the substring is found within the string. It’s case-sensitive, so be mindful of that when performing your checks.
Here’s a simple example:
const text: string = "Hello, TypeScript world!";
const searchText: string = "TypeScript";
const containsText: boolean = text.includes(searchText);
console.log(containsText);
Output:
true
In this code, we define a string called text
and a substring called searchText
. The includes
method checks if searchText
exists within text
. The result, stored in containsText
, is true
because “TypeScript” is indeed part of the original string. This method is not only easy to use but also very readable, making your code more maintainable.
Using the indexOf
Method
Another method to check for a substring in a string is the indexOf
method. This method returns the index of the first occurrence of the specified substring. If the substring is not found, it returns -1
. This can be useful if you also want to know the position of the substring within the string.
Here’s how you can use it:
const text: string = "Learning TypeScript is fun!";
const searchText: string = "TypeScript";
const index: number = text.indexOf(searchText);
const containsText: boolean = index !== -1;
console.log(containsText);
Output:
true
In this example, we again have a string text
and a substring searchText
. We use indexOf
to find the position of searchText
within text
. If the result is not -1
, it means the substring exists, and we set containsText
to true
. This method provides not just the presence of the substring but also its position, which can be valuable in various scenarios.
Using Regular Expressions
For more complex string checks, you might want to use regular expressions. TypeScript, being a superset of JavaScript, supports regex natively. This method allows for advanced pattern matching, making it a powerful tool for string manipulation.
Here’s an example of how to use regex to check for a substring:
const text: string = "TypeScript is a powerful language.";
const searchText: string = "TypeScript";
const regex: RegExp = new RegExp(searchText);
const containsText: boolean = regex.test(text);
console.log(containsText);
Output:
true
In this instance, we create a regular expression from searchText
and use the test
method to check if it exists in text
. The test
method returns true
if a match is found. Regular expressions are incredibly versatile, allowing you to perform more sophisticated checks, such as case-insensitive searches or pattern matching.
Conclusion
Checking if a string contains a certain text in TypeScript can be accomplished in several ways, each suited for different scenarios. Whether you choose the straightforward includes
method, the informative indexOf
method, or the powerful regex approach, understanding these techniques will enhance your string manipulation capabilities. As you continue to work with TypeScript, these methods will become invaluable tools in your development toolkit.
FAQ
- What is the difference between
includes
andindexOf
?
Theincludes
method returns a boolean indicating whether the substring is found, whileindexOf
returns the position of the substring or-1
if not found.
-
Are the methods case-sensitive?
Yes, bothincludes
andindexOf
methods are case-sensitive. If you need a case-insensitive check, consider using regular expressions. -
Can I use regular expressions in TypeScript?
Yes, TypeScript supports regular expressions, just like JavaScript. You can use them for advanced string checks. -
What should I use for simple substring checks?
For simple checks, theincludes
method is recommended due to its readability and ease of use. -
How do I check for multiple substrings?
You can use a loop or an array method likesome
orevery
to check for multiple substrings within a string.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn