How to Convert String to Boolean in TypeScript
- Create a TypeScript Project
- Test the String Using a Regular Expression
- Use the Strict Equality Operator
-
Use the
parse()
Method of JSON API -
Use the
switch
Statement - Conclusion
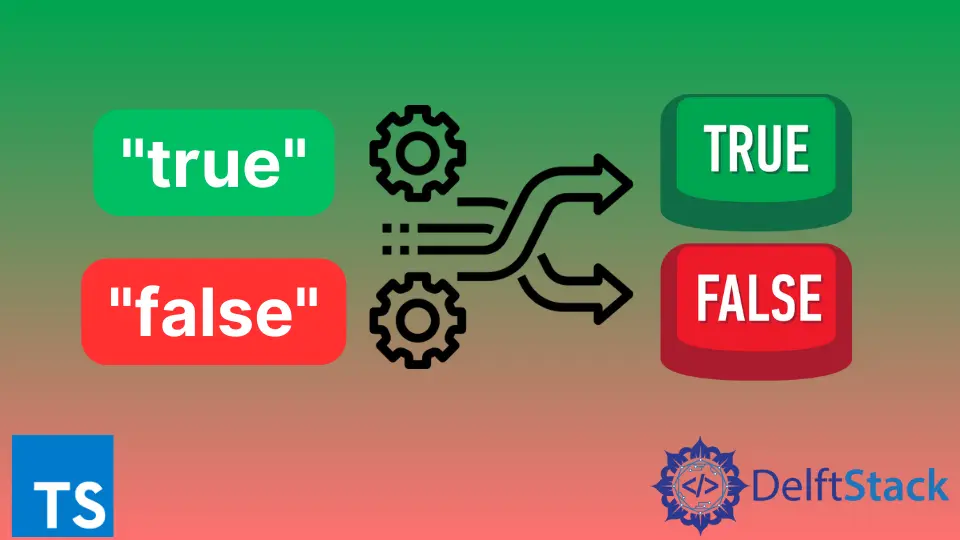
Conversion of one data type to another is a common programming approach used when creating applications as it helps to get the output in a different format given the same input.
For example, when working with numeric data, you might want to retrieve a request parameter of type number from a request, and since the request is of type string, we can use the parseInt()
or parseFloat()
methods to convert the request parameter to type Number
.
This approach is not only limited to numeric values, but it also provides us with other ways that we can leverage to convert one data type to another data type. In this tutorial, we will learn the different ways we can use to convert a string data type to a Boolean data type.
Create a TypeScript Project
Open WebStorm IDEA
and select File
> New
> Project
. On the window that opens, select Node.js
on the left side, then on the right side, change the project name
from untitled
to string-to-boolean
or enter any name preferred.
Ensure you have installed node
before creating this project to ensure the two remaining sections, Node interpreter
and Package manager
, is automatically added from the file system.
Once the project has been generated, open a new terminal window using the keyboard shortcut Alt+F12 and use the following command to create a configuration file named tsconfig.json
.
~/WebstormProjects/string-to-boolean$ tsc --init
Ensure the contents of the file are as shown below. The only change we have made is adding the noEmitOnError: true
to ensure the JavaScript files are not created if an error occurs during the transpile of TypeScript code.
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"noEmitOnError": true,
"strict": true,
"noFallthroughCasesInSwitch": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true
}
}
Test the String Using a Regular Expression
Create a file named Main.ts
under the folder string-to-kotlin
and copy and paste the following code into the file.
const trueFlag = "true";
const falseFlag = "false";
function usingTestMethod(){
const flag = (/true/i).test(trueFlag);
console.log(flag);
}
usingTestMethod();
In this code, we have created two string flags and a method named usingTestMethod()
; the string flags contain the values true
and false
, respectively. They are declared global variables since they will be used in all the examples in this tutorial.
The usingTestMethod()
uses the test()
method of RegExp
interface to create the regular expression /true/i
that returns true
if the string matches the regular expression.
Note that if the string does not match the regular expression, we will get a false
Boolean result. Since the string flag contains the string true
, the regular expression will match and return true
.
Run this code using the following command.
~/WebstormProjects/string-to-boolean$ tsc && node Main.js
The tsc
command transpile the TypeScript code to JavaScript code and generates a file named Main.js
. The node
command executes the Main.js
file, and the value returned by the code is logged to the console.
true
Use the Strict Equality Operator
Copy and paste the following code into the Main.ts
file after the usingTestMethod()
function.
function usingStrictEqualityOperator(){
const flag = (trueFlag === "true");
console.log(flag);
}
usingStrictEqualityOperator();
In this method, we have created a method named usingStrictEqualityOperator()
that uses the strict equality operator ===
to test whether the string flag containing the value true
is strictly equal to the string we pass as the argument.
Why not use the equal operator ==
? This is to avoid type coercion that might return a different result than the expected one.
Since the two strings are equal, the strict equality operator will return the value of true
. Run this code using the command we used in the previous example and ensure the output is as shown below.
true
Use the parse()
Method of JSON API
Copy and paste the following code into the Main.ts
file after the usingStrictEqualityOperator()
method.
function usingJSONParser(){
const flag = JSON.parse(falseFlag);
console.log(flag);
}
usingJSONParser();
In this code, we have created a method named usingJSONParser()
that uses the parse()
method of the JSON interface to parse the text provided as the argument to a Boolean value.
In our case, we have passed the variable falseFlag
that contains a string flag with the value "true"
, and the parse
method returns a Boolean value of true
from this string.
Note that depending on the requirement, we can return an Object
, Array
, string
, or number
.
Run this code using the command we used in the previous example and ensure the output is as shown below.
false
Use the switch
Statement
Copy and paste the following code into the Main.ts
file after the usingJSONParser()
method.
function flagResult(flag: any){
switch (flag){
case true:
case "true":
case 1:
case "1":
case "on":
case "yes":
return true;
default:
return false;
}
}
function usingSwitchStatement(){
const flag = flagResult(trueFlag);
console.log(flag);
}
usingSwitchStatement();
In this code, we have created two methods named flagResult()
and usingSwitchStatement()
. The flagResult()
method accepts one parameter of type any
; depending on the argument passed to the method, a Boolean value of true
or false
is returned.
The method returns a value of true
for any of the arguments true
, "true"
, 1
, on
, or yes
; otherwise, a value of false
is returned.
The usingSwitchStatement()
uses this method to get the Boolean value of the string flag trueFlag
, which was declared at the beginning of this tutorial. The variable flag
contains the result of passing the trueFlag
to the flagResult()
method.
Run this code using the command we used in the previous example and ensure the output is as shown below.
true
Conclusion
This tutorial taught us how to convert a string value to a Boolean value. The topics we have covered include testing a string using a regular expression, using the strict equality operator, using the parse()
method of JSON API, and finally, we learned how to use the switch
statement.
Note that libraries can help us realize the same objective, and a developer should be open to using any other approach we have not covered in this tutorial.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub