How to Convert a Boolean to a String Value in TypeScript
- Main Types in TypeScript
-
Use the
String()
Global Function to Convert a Boolean to a String Value in TypeScript -
Use the
toString()
Method to Convert a Boolean to a String Value in TypeScript - Use the Ternary Operator to Convert a Boolean to a String Value in TypeScript
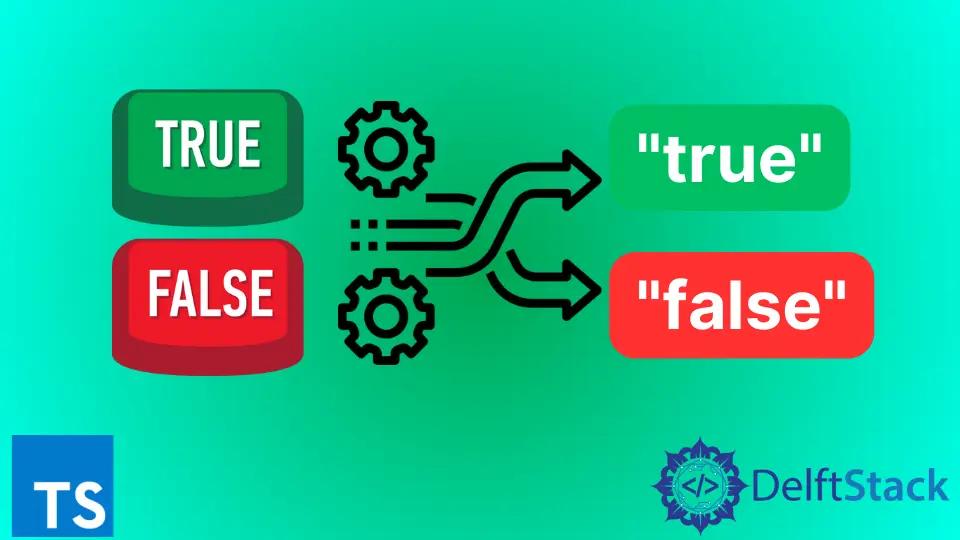
This article will discuss the various ways to convert a primitive boolean value to a string value in TypeScript.
Main Types in TypeScript
TypeScript is a strongly typed superset of the JavaScript programming language. It allows programmers to specify the types for variables, return values, and method parameter values.
Hence, TypeScript checks the validity of these values. It will allow you to catch errors in the compile-time, ensuring smooth execution of your programs.
There are two main types: Primitive types and Object types.
Primitive Types in TypeScript
The primitive types represent the type for each JavaScript primitive. One of the main JavaScript primitives is boolean, and the same name denotes the corresponding TypeScript type.
Let’s define a boolean type variable as shown in the following.
let boolValue: boolean = false;
If you check the type of boolValue
, it should be a boolean type.
console.log(typeof boolValue);
Output:
boolean
Object Types in TypeScript
There is another type called object, representing all the other JavaScript values except the primitives. There are wrapper objects available for primitives, as shown in the following.
Boolean
object - Wrapper around the primitive booleanString
object - Wrapper around the primitive stringNumber
object - Wrapper around the primitive number
It is always recommended to use the primitive types instead of object types. The above understanding is helpful when converting boolean to string type.
There are a few different ways that you can use to convert a boolean to a string value.
Use the String()
Global Function to Convert a Boolean to a String Value in TypeScript
This special function converts a given argument to a primitive string value. Other global functions available are the same as this, such as Boolean()
and Number()
.
Syntax:
String(value_to_be_converted)
The value_to_be_converted
argument can be a string, boolean, or number.
Let’s create a Boolean type variable boolVal
.
let boolVal: boolean = true;
We can use the typeof
operator to check whether the boolVal
is a boolean type.
console.log(typeof boolVal);
Output:
boolean
Now, let’s use the String()
global function to convert the boolVal
boolean value to a primitive string value. We will be assigning the returned primitive string value to another variable called convertedBoolVal
.
Finally, we will print the value and the type of the convertedBoolVal
.
let convertedBoolVal: string = String(boolVal);
console.log(convertedBoolVal);
console.log(typeof convertedBoolVal);
Output:
"true"
string
This is the most recommended way to convert a primitive boolean value to a string value.
Use the toString()
Method to Convert a Boolean to a String Value in TypeScript
In JavaScript, everything inherits from the Object type. The toString()
is one of the widely popular methods to convert a given object to its string representation.
Since the TypeScript is a superset of JavaScript, a valid JavaScript code is technically a valid TypeScript. Hence, we can use the toString()
method to convert a given boolean value to a string value.
Syntax:
boolean_value.toString()
This method returns a primitive string value.
Let’s create a boolean type variable called isTired
and assign it to false
.
let isTired: boolean = false;
We will now use the toString()
method to convert the isTired
value to a primitive string type.
let isTiredConverted: string = isTired.toString();
Finally, we’ll print the value and type of the isTiredConverted
variable. It should have been converted to a string type.
console.log(isTiredConverted);
console.log(typeof isTiredConverted);
Output:
"false"
string
Use the Ternary Operator to Convert a Boolean to a String Value in TypeScript
There is a tricky way to convert a primitive boolean value to a string with the ternary operator. The ternary operator is a short way of writing the if..else..
block.
Syntax:
condition ? value_1 : value_2
If the condition
is evaluated to be true, it will return the value_1
. Else, the value_2
is returned.
Let’s create two boolean-type variables to store two primitive boolean values.
let boolTrue: boolean = true;
let boolFalse: boolean = false;
Then we’ll use the ternary operator to convert the above two primitive boolean values to their string values. The converted values will be stored in two separate string-type variables, as shown in the following.
let boolTrueConverted: string = boolTrue ? "true" : "false";
let boolFalseConverted: string = boolFalse ? "true" : "false";
Next, we will print the types and values of boolTrueConverted
and boolFalseConverted
.
console.log(boolTrueConverted);
console.log(typeof boolTrueConverted);
console.log(boolFalseConverted);
console.log(typeof boolFalseConverted);
Output:
"true"
string
"false"
string
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.
Related Article - TypeScript String
- How to Convert String to Boolean in TypeScript
- How to Check if a Variable Is a String in TypeScript
- How to Check if a String Has a Certain Text in TypeScript
- How to Have Multiline Strings in TypeScript
- How to Compare Strings in TypeScript