How to Check Interface Type in TypeScript
- Type Guards in TypeScript
-
Use
typeof
to Check Types in TypeScript -
Use
instanceof
in TypeScript -
Use the
in
Keyword to Check for Types in TypeScript
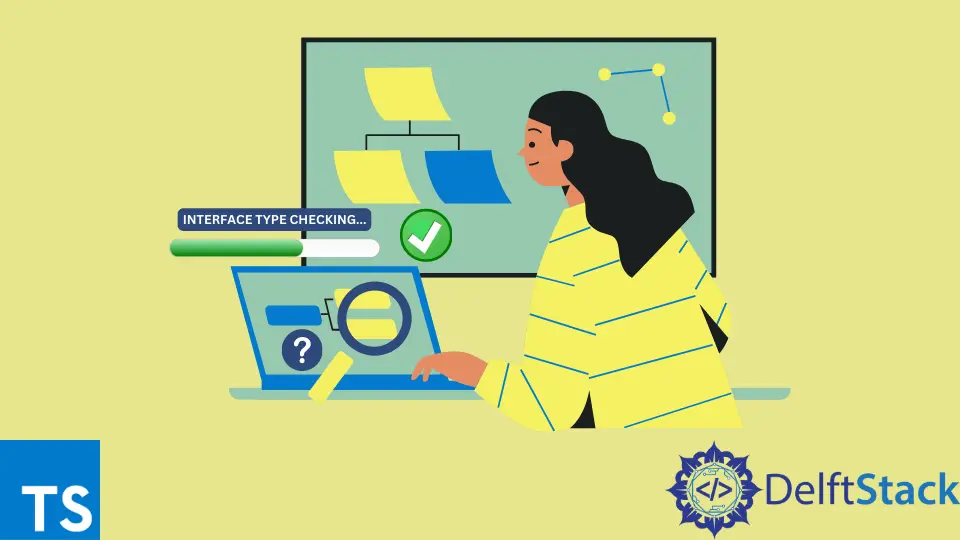
Interfaces are an important part of TypeScript for associating types to variables and elements in TypeScript to ensure type safety and thus avoiding runtime errors. Classes and other interfaces can implement interfaces, and thus this can be used for dynamic behaviors in the actual code.
Thus, knowing the actual interface type can sometimes be required to ensure the type safety, just like the instanceof
keyword in Java. This tutorial will demonstrate how the types of interfaces can be checked to ensure type safety.
Type Guards in TypeScript
A type guard is a check on the different types present in TypeScript to ensure type safety. It is in the form of conditional statements.
It is especially needed when a certain type is a composition of one or more types. Various keywords are available through which interfaces can be checked, such as typeof
, instanceof
, in
, and one can even make custom type guards.
interface Animal {
name : string;
legs : number;
eyes : number;
}
interface Dog extends Animal {
bark : () => void
}
interface Cat extends Animal {
meow : () => void;
}
function getAnimal() : Dog | Cat {
let choice = Math.random();
if ( choice > 0.5){
const dog : Dog = {
name : 'Labrador',
legs : 4,
eyes : 2,
bark : () => console.log("Woof")
}
return dog;
}else {
const cat : Cat = {
name : 'Labrador',
legs : 4,
eyes : 2,
meow : () => console.log("Meow")
}
return cat;
}
}
const animal = getAnimal();
// Property 'meow' does not exist on type 'Dog | Cat'.
animal.meow();
// Property 'bark' does not exist on type 'Dog | Cat'.
animal.bark();
In the above example, the properties meow
or the bark
functions cannot be resolved as the TypeScript compiler is unsure what to infer. The getAnimal
function returns a union of two types, Dog | Cat
, which confuses the compiler.
Type guards or type checks must be introduced to make the compiler understand the appropriate type.
if ( 'meow' in animal){
animal.meow();
} else if ( 'bark' in animal){
animal.bark();
}
The above is an example of how type checks can be enforced to ensure type safety. The meow
and the bark
attributes are checked if they are present in the animal
object and are called accordingly.
Use typeof
to Check Types in TypeScript
The typeof
keyword can be used to determine the types of variables; however, it has a very limited scope. It can be used for checking primitive types.
The values returned by the typeof
keyword can be of string
, number
, bigint
, boolean
, symbol
, undefined
, object
or function
.
The typeof
keyword returns all the complex types and null
values as an object.
const displayBill = ( amount : string | number ) => {
if ( typeof amount === 'string') {
amount = Number(amount);
}
let tax = (18.5 * amount) / 100;
console.log('Bill : '+ amount + " $");
console.log('Tax : '+ tax + " $");
console.log('Total Payable : '+ (amount + tax) + " $");
}
displayBill(23.5);
Output:
"String conversion being done!"
"Bill : 23.5 $"
"Tax : 4.3475 $"
"Total Payable : 27.8475 $"
Thus in the above example, the typeof
keyword has been used to check on the type of the variable amount
, and conversion has been done after the check. This check is necessary for calculating the tax
variable, which requires the amount
to be of type number
.
Use instanceof
in TypeScript
The instanceof
keyword checks for variables corresponding to some classes. The following type guard is used for those variables, which are instantiated as class objects.
class User {
name : string;
amountDue : number;
constructor( name : string, amount : number){
this.name = name;
this.amountDue = amount;
}
}
class UserCredit extends User {
constructor( user : User) {
super(user.name, user.amountDue);
}
generateCredit(amount : number) {
this.amountDue += amount;
return this.amountDue;
}
}
class UserDebit extends User {
constructor( user : User) {
super(user.name, user.amountDue);
}
settleDebt(){
this.amountDue = 0;
}
}
const TransactionSystem = () => {
const user : User = {
name : 'Alex',
amountDue : 0
}
const option = Math.random();
if ( option > 0.5) {
// settle debts
const userDebt = new UserDebit(user);
userDebt.settleDebt();
return userDebt;
} else {
// increase credit
const userCredit = new UserCredit(user);
userCredit.generateCredit(500);
return userCredit;
}
}
const UserTransaction = TransactionSystem();
if ( UserTransaction instanceof UserDebit) {
console.log('Credit balance successfully debited');
} else if (UserTransaction instanceof UserCredit) {
console.log("Credit limit increased")
}
Use the in
Keyword to Check for Types in TypeScript
The in
can be used to check if a certain attribute is present in a type or an interface and thus can be used with a conditional statement like if-else
to check for types and thus act upon and execute further actions. The following shows an example of how this can be achieved.
interface Person {
name : string;
age : number;
}
const person : Person = {
name : "Alex",
age : 30
}
console.log("name" in person); // true
console.log("address" in person); // false