How to Interface Default Value in TypeScript
-
What Is
Interface
in TypeScript -
Use Optional Properties to Set
Interface
Default Values inTypeScript
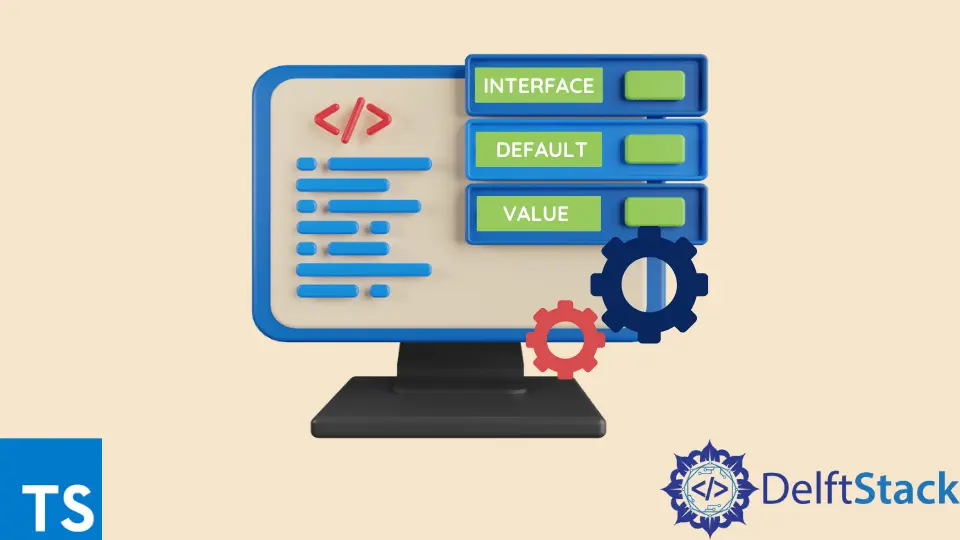
This tutorial provides an alternative solution to define Interface
default values in TypeScript. It gives coding examples and outputs and knowledge about what interfaces are and why they are used.
What Is Interface
in TypeScript
TypeScript has a core principle of type checking that focuses on a value’s shape; sometimes, this is called duck typing or structural subtyping. Interfaces
in TypeScript fill the role of defining contracts within the code and the code outside of the project.
The following is how you define Interface
in TypeScript.
interface LabeledVal {
label: string;
}
function printLabelTraces(labeledObject: LabeledVal) {
console.log(labeledObject.label);
}
let myObject = { size: 10, label: "Size 10 Object" };
printLabelTraces(myObject);
The above code produces the following output.
This Interface
describes the requirement of having the label
property, that is, a string. The Interface
LabeledVal
is a name we can use to describe the Interface
implemented by the object we pass to printLabelTraces
as we might have in other languages.
If the object we have passed to the function successfully meets the requirements listed in the Interface
, it’s allowed.
Let’s now look at how to define Interface
default values in TypeScript
.
Use Optional Properties to Set Interface
Default Values in TypeScript
Since all the properties are not required to be present in an object implementing the interface, Optional Properties is the popular option available for providing pattern-like option bags where an object to a function only has a couple of properties filled in. Consider the following example of a code.
interface SquareConfig {
color?: string;
width?: number;
}
function Square(config: SquareConfig): { color: string; area: number } {
let newSquare = { color: "white", area: 100 };
if (config.color) {
newSquare.color = config.color;
}
if (config.width) {
newSquare.area = config.width * config.width;
}
return newSquare;
}
let SquareVal = Square({ color: "black" });
console.log(SquareVal);
In this code, the SquareVal
takes the return value from the function Square
, which has a parameter type of interface
SquareConfig
, which has an optional value that can be assigned or not depending upon the situation of usage. The above code produces the following output.
The way to write the Interfaces with optional properties is the same as writing the normal interface with ?
with the optional property in the declaration at the end of the property name. This prevents the use of properties that are not part of the interface.
Consider an example of the mistyped name of the color
property in Square
; we would get an error message.
// @errors: 2551
interface SquareConfig {
color?: string;
width?: number;
}
function Square(config: SquareConfig): { color: string; area: number } {
let newSquare = { color: "white", area: 100 };
if (config.clor) {
// Error: Property 'clor' does not exist on type 'SquareConfig'
newSquare.color = config.clor;
}
if (config.width) {
newSquare.area = config.width * config.width;
}
return newSquare;
}
let SquareVal = Square({ color: "black" });
There is no other way for providing default values for interfaces or type aliases as they are compile-time only, and for the default values, we need runtime support. The above optional properties provide an alternative solution to the problem.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn