Function Interface in TypeScript
- What is a Function Interface?
- Defining a Function Interface
- Using Function Interfaces in Callbacks
- Conclusion
- FAQ
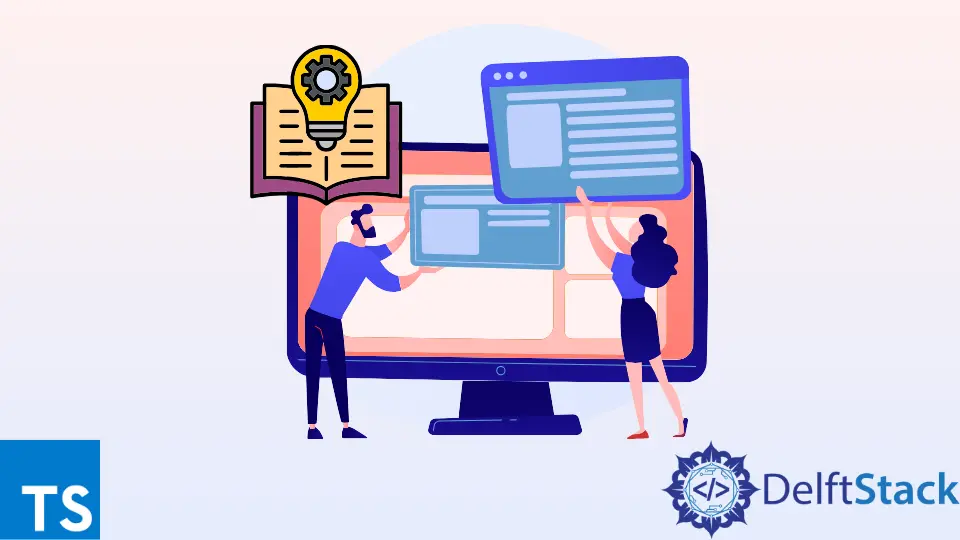
TypeScript is a powerful superset of JavaScript that adds static typing to the language. One of its standout features is the ability to define interfaces, which can be used to describe the shape of an object or function.
In this article, we will delve into the concept of function interfaces in TypeScript, demonstrating how to use them effectively. By the end, you will have a solid understanding of how to define and implement function interfaces, enhancing both your coding skills and your ability to create more maintainable code. Whether you’re a beginner or an experienced developer, this guide will provide valuable insights into leveraging TypeScript’s interface capabilities.
What is a Function Interface?
In TypeScript, a function interface is a way to define the structure of a function. This includes the types of its parameters and its return type. By using interfaces, developers can create contracts that functions must adhere to, ensuring consistency and reducing errors in code.
For instance, if you have a function that takes two numbers and returns their sum, you can define an interface that specifies this requirement. This not only helps with type checking but also improves code readability and maintainability.
Here’s a simple example of defining a function interface:
interface SumFunction {
(a: number, b: number): number;
}
const add: SumFunction = (x, y) => x + y;
In this example, we define an interface called SumFunction
that describes a function taking two numbers and returning a number. We then create a function add
that implements this interface.
Output:
add(5, 10) returns 15
The use of interfaces in this way helps to ensure that any function adhering to the SumFunction
interface will follow the same structure, making it easier to work with and understand.
Defining a Function Interface
To define a function interface in TypeScript, follow these steps:
- Create the Interface: Start by declaring an interface that describes the function signature. Specify the parameter types and the return type.
- Implement the Interface: Create a function that adheres to the defined interface. This ensures that the function matches the expected structure.
Here’s a more detailed example where we define an interface for a function that processes user data:
interface UserProcessor {
(userId: string, userName: string): string;
}
const processUser: UserProcessor = (id, name) => {
return `User ID: ${id}, User Name: ${name}`;
};
In this case, the UserProcessor
interface specifies that any function implementing it must accept a string for both the user ID and the user name and return a string. The processUser
function follows this contract, ensuring that it adheres to the expected structure.
Output:
processUser("123", "John Doe") returns "User ID: 123, User Name: John Doe"
This approach is particularly useful in larger applications where functions may have complex interactions. By using interfaces, you can ensure that functions are called with the correct parameters, reducing the likelihood of runtime errors.
Using Function Interfaces in Callbacks
Function interfaces are especially useful when dealing with callbacks. In many cases, you may need to pass functions as arguments to other functions. By defining a function interface, you can ensure that these callbacks meet specific criteria.
Consider a scenario where you want to filter an array of numbers. You can define a function interface for the callback that specifies the expected parameter and return types:
interface FilterCallback {
(value: number): boolean;
}
const filterNumbers = (arr: number[], callback: FilterCallback): number[] => {
return arr.filter(callback);
};
const isEven: FilterCallback = (num) => num % 2 === 0;
const numbers = [1, 2, 3, 4, 5, 6];
const evenNumbers = filterNumbers(numbers, isEven);
In this example, the FilterCallback
interface defines a function that takes a number and returns a boolean. The filterNumbers
function accepts an array and a callback, filtering the array based on the callback’s logic. The isEven
function implements the FilterCallback
interface, checking if a number is even.
Output:
evenNumbers returns [2, 4, 6]
This pattern is incredibly powerful, as it allows you to create reusable and type-safe functions that can work with various callback implementations. By leveraging function interfaces, your code becomes more modular and easier to maintain.
Conclusion
Function interfaces in TypeScript are a valuable tool for developers looking to create robust and maintainable code. By defining the structure of functions, you can ensure consistency across your application and reduce the risk of errors. Whether you’re handling simple functions or complex callbacks, understanding how to use function interfaces will enhance your TypeScript skills and improve your overall coding practices. As you continue to work with TypeScript, consider integrating function interfaces into your projects to reap the benefits of type safety and improved readability.
FAQ
-
What is a function interface in TypeScript?
A function interface in TypeScript defines the structure of a function, including its parameters and return type. -
Why should I use function interfaces?
Function interfaces help ensure consistency and type safety in your code, making it easier to maintain and understand. -
Can I use function interfaces for callbacks?
Yes, function interfaces are particularly useful for defining the structure of callback functions, ensuring they meet specific criteria. -
How do I implement a function interface?
To implement a function interface, create a function that matches the defined structure, including the correct parameter types and return type. -
Are function interfaces only for functions?
While primarily used for functions, interfaces in TypeScript can also describe the shape of objects and other structures, providing flexibility in your code design.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn