Interface vs. Class in TypeScript
- Differences Between Classes and Interfaces in TypeScript
- When to Use Classes vs. Interfaces in TypeScript
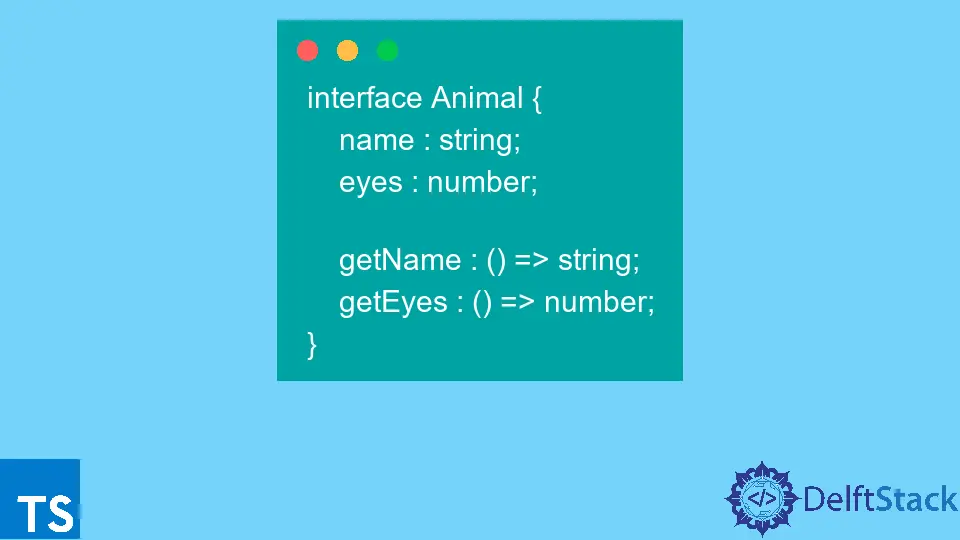
TypeScript is a strongly typed language and supports complex and primitive types. It is a superset of JavaScript programming language, and as a result, it also has the different features present in JavaScript, such as Object-Oriented Programming (OOP) concepts and functional programming concepts.
TypeScript becomes extremely useful for organizations having large codebases. TypeScript helps prevent run time errors which would be inevitable in the case of JavaScript for wrongly inferred types.
Proper tools and IDE supporting TypeScript can suggest code completions, detect errors in types, automatically fix errors, and more through various interfaces defined by the user or by types inferred by the TypeScript compiler.
This tutorial will focus on the differences between classes and interfaces and will demonstrate when to use which to model some data.
Differences Between Classes and Interfaces in TypeScript
Classes are essentially required to hold the implementation of different objects with different properties and methods associated with them. Classes may have initialized properties and have implementations of various methods inside them and can be used to initialize or create different objects.
Interfaces are only used for holding the type information of various attributes corresponding to an object. It is generally used for type checking and thus preventing runtime errors and cannot be used for object instantiation.
class Animal {
name : string;
eyes : number;
constructor( name : string, eyes : number) {
this.name = name;
this.eyes = eyes;
}
getName() : string {
return this.name;
}
getEyes() : number {
return this.eyes;
}
}
The above code block shows the implementation of a TypeScript class that contains the blueprint of the different attributes associated with the Animal
class and has some methods. The same data can be modeled using an interface.
interface Animal {
name : string;
eyes : number;
getName : () => string;
getEyes : () => number;
}
Thus according to the interface, it has all the possibilities of type checking on any object implementing the interface, but unlike classes, it has no way of initializing the properties of a class and cannot have any implementation for the functions in a class.
When to Use Classes vs. Interfaces in TypeScript
Classes are used as an object blueprint containing default values, different method implementations, or while using the new
keyword to create different instances of the same class. Moreover, with classes, the code will take up space at runtime, unlike interfaces removed by the TypeScript compiler.
Classes have constructors for adding some initializing code and initializing data variables in the class, unlike the interfaces.
Thus classes are used to represent some complex data models whose objects may need to be created and often are required to be extended and may be required to have initialization logic attached to it.
Meanwhile, interfaces are used to have a simple data modeling, consisting of only the types of the attributes associated with an object.
They can also act as data interface of some classes, say class Tiger
implements Animal
- the class Tiger
will have all the type checking support with the same attributes as present in the interface Animal
.
Related Article - TypeScript Class
- How to Get Class Name of an Object in TypeScript
- How to Implement Class Constants in TypeScript
- Static Class in TypeScript