How to Implement Class Constants in TypeScript
- Constants in TypeScript
-
Implement Class Constants Using
readonly
in TypeScript -
Implement Class Constants Using
readonly
Withstatic
in TypeScript
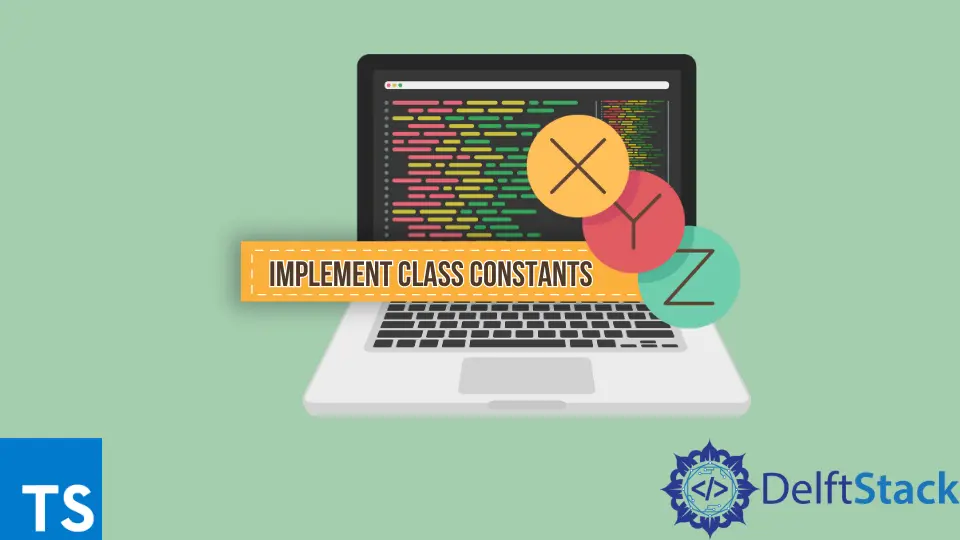
This article teaches how to implement class constants using the readonly
keyword and the static
keyword with the readonly
keyword.
Constants in TypeScript
Constants in programming languages are the fixed values that, once assigned, the value cannot be changed. Class constants are constant fields inside a class that are not alterable once assigned.
In TypeScript, creating a class is a straightforward task. Below is the syntax of how we can create a class in TypeScript.
class className {
// Block of code
}
The above is the simple method to create a class in TypeScript. We can follow a few methods to create a class with constant fields or properties with values that are not alterable or changeable.
In TypeScript, it is essential to remember that a class member cannot have the const
keyword. An inline declaration of a constant of a class member is impossible in TypeScript.
If we try to do so, the compiler will throw a compilation error. To make a class constant, we can implement a few options available in TypeScript discussed in the following sections.
Implement Class Constants Using readonly
in TypeScript
After creating a class to make the class properties or fields non-alterable, it is possible to use the readonly
keyword, which was introduced in TypeScript 2.0, to define class fields or properties as non-alterable. If we try to alter the properties, an error message would appear.
Example:
class DisplayOutput{
readonly name : string = "Only using readonly";
displayOutput() : void{
console.log(this.name);
}
}
let instance = new DisplayOutput();
instance.displayOutput();
Output:
Only using readonly
When only readonly
is used, it does not fully act as a constant. This is due to the assignment being allowed at the constructor.
Implement Class Constants Using readonly
With static
in TypeScript
Another method we can use to make class fields or properties non-alterable is the readonly
keyword with the static
keyword. The advantage of this compared to just using readonly
is that assignment will not be allowed in the constructor and therefore only be at one place.
Example:
class DisplayOutput{
static readonly username : string = "Using both static and readonly keywords";
static displayOutput() : void {
console.log(DisplayOutput.username);
}
}
DisplayOutput.displayOutput();
Output:
Using both static and readonly keywords
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.