How to Get Class Name of an Object in TypeScript
- TypeScript Classes and Objects
-
Use the
instanceof
Operator to Get the Class Name of an Object in TypeScript -
Use
Object.getPrototypeOf()
to Get Class Name of an Object in TypeScript -
Use the
constructor
Property to Get Class Name of an Object in TypeScript - Use a Custom Property to Get the Class Name of an Object in TypeScript
- Conclusion
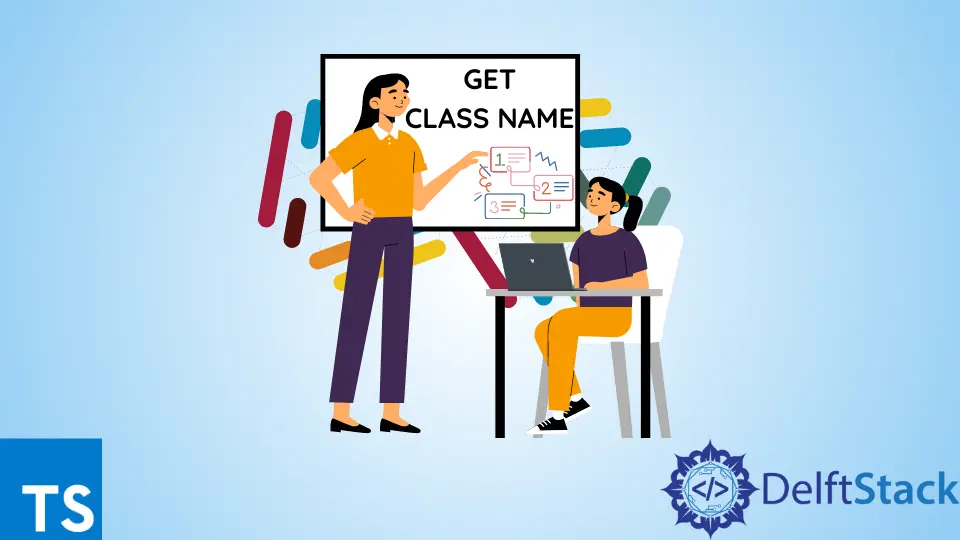
In TypeScript, obtaining the class name of an object at runtime is a common task for various purposes, such as debugging, logging, or dynamic behavior based on object types.
To achieve this, TypeScript offers multiple approaches, including using the instanceof
operator, Object.getPrototypeOf()
, the constructor
property, or even custom properties. These techniques allow developers to determine the class name of an object, enhancing code readability and maintainability.
In this guide, we’ll explore these methods in detail, providing code examples and explanations for each approach. But first, let’s understand what classes and objects are in TypeScript.
TypeScript Classes and Objects
Classes in TypeScript serve as blueprints for creating objects. They encapsulate data (properties) and behavior (methods) related to a specific entity.
Classes help in organizing code by defining the structure and behavior of objects. Objects, also known as instances, are created from classes.
They represent individual entities with specific attributes and behaviors defined by their class. These objects can interact with each other and perform actions based on their defined methods.
Let’s look at the following code to understand further.
class Animal {
// properties/fields
name: string;
color: string;
// constructor
constructor(name: string) {
this.name = name;
}
// methods/functions
eat(): void {
console.log("I am eating");
}
}
In this code, the fields are defined to store the name
and color
of an Animal
object. A TypeScript constructor
is used to initialize the object’s properties. It is a special function that can be parameterized.
With the Animal
class in place, it is possible to create objects from that. TypeScript uses the new
keyword to create new objects from a given class.
Since TypeScript is a strongly typed language, the type of the new Animal
instances will be in the Animal
type.
let dog: Animal = new Animal('rocky');
As you can see, the Animal
class’ constructor has been called when creating the Animal
instance. The Animal
class constructor accepts one string parameter, and we had to pass it while creating the object.
Obtaining the class name of an object dynamically can be beneficial in scenarios where you need to perform actions based on the type of object you’re dealing with. This information is particularly valuable when working with inheritance, polymorphism, and scenarios involving complex object hierarchies.
Use the instanceof
Operator to Get the Class Name of an Object in TypeScript
The instanceof
operator is a built-in operator in JavaScript and TypeScript that allows you to determine whether an object is an instance of a particular class or constructor function.
It returns true
if the object is an instance of the specified class and false
otherwise. This operator is essential for implementing polymorphic behavior and ensuring that objects adhere to expected interfaces.
Syntax:
object instanceof class
object
: The instance you want to check.class
: The class or constructor function you’re testing against.
The instanceof
operator is primarily used for type-checking, but it can also be used to retrieve the class name. The code below demonstrates the use of the instanceof
operator and the constructor
property to retrieve the class name of an object at runtime in TypeScript.
class Person {
name: string;
constructor(name: string) {
this.name = name;
}
}
const john = new Person("John Doe");
if (john instanceof Person) {
const className = (john.constructor as any).name;
console.log(`Class Name: ${className}`);
}
Here’s a breakdown of the code:
We define a Person
class with a name
property and a constructor that takes a name
parameter and assigns it to the name
property.
Then, we create an instance of the Person
class with the name "John Doe"
and assign it to the variable john
.
Next, we use the instanceof
operator to check if john
is an instance of the Person
class. This operator returns true
if john
is an instance of Person
.
Inside the if
block, we access the constructor
property of john
using (john.constructor as any).name
. This returns the class name of john
.
The output of console.log()
will be:
Class Name: Person
Use Object.getPrototypeOf()
to Get Class Name of an Object in TypeScript
Object.getPrototypeOf()
is a built-in JavaScript and TypeScript function that returns the prototype (i.e., the class) of an object. By leveraging this function, you can access information about the object’s inheritance chain, including its constructor function.
Syntax:
Object.getPrototypeOf(obj)
Here, obj
is the object for which you want to retrieve the prototype.
The function Object.getPrototypeOf()
takes one argument, which is the object you want to inspect. It returns the prototype of the specified object.
For example, if you have an object myObject
, you can use Object.getPrototypeOf()
to get its prototype like this:
const myObject = {}; // Create an object
const prototype = Object.getPrototypeOf(myObject);
prototype
will now hold the prototype of myObject
.
Keep in mind that Object.getPrototypeOf()
is a static method of the Object
class, so you don’t need to create an instance of Object
to use it.
The code below demonstrates the use of Object.getPrototypeOf()
to retrieve the class name of an object at runtime in TypeScript.
class Person {
name: string;
constructor(name: string) {
this.name = name;
}
}
const john = new Person("John Doe");
const prototype = Object.getPrototypeOf(john);
const className = prototype.constructor.name;
console.log(`Class Name: ${className}`); // Output: Class Name: Person
Here’s a breakdown of the code:
We define a Person
class with a name
property and a constructor that takes a name
parameter and assigns it to the name
property.
Next, we create an instance of the Person
class with the name "John Doe"
and assign it to the variable john
.
We use Object.getPrototypeOf(john)
to retrieve the prototype of the john
object. This prototype is the Person
class itself.
We then access the constructor
property of the prototype, which refers to the constructor function that created the object.
Finally, we retrieve the name
property of the constructor function, which gives us the class name "Person"
.
The output of console.log()
will be:
"Class Name: Person"
Use the constructor
Property to Get Class Name of an Object in TypeScript
In TypeScript, the constructor
property is an inherent property of every class. It refers to the constructor function that was used to create an instance of the class.
The syntax to access the constructor
property is as follows:
class ClassName {
// Class members and methods
constructor(parameters) {
// Constructor logic
}
}
const instance = new ClassName();
const constructorFunction = instance.constructor;
ClassName
: The name of the class.constructor
: The class constructor method.parameters
: The parameters that can be passed to the constructor when creating an instance of the class.instance
: An instance of the classClassName
.constructorFunction
: Holds the reference to the constructor function.
Keep in mind that the constructor
property is available on instances of classes, not on the class itself. So, you can access it after creating an instance of the class, as shown in the syntax above.
Every JavaScript/TypeScript object has a constructor
property that refers to the constructor function used to create the object. Accessing the name
property of this constructor provides the class name.
The code below demonstrates a straightforward and effective way to retrieve the class name of an object in TypeScript using the constructor
property.
class Person {
name: string;
constructor(name: string) {
this.name = name;
}
}
const john = new Person("John Doe");
const className = john.constructor.name;
console.log(`Class Name: ${className}`); // Output: Class Name: Person
Here’s a breakdown of the code:
We define a Person
class with a name
property and a constructor that takes a name
parameter and assigns it to the name
property.
Then, we create an instance of the Person
class with the name "John Doe"
and assign it to the variable john
.
We access the constructor
property of john
using john.constructor
. This returns the constructor function that was used to create john
.
Finally, we access the name
property of the constructor function. This gives us the class name "Person"
.
The output of console.log()
will be:
Class Name: Person
Use a Custom Property to Get the Class Name of an Object in TypeScript
Creating a custom property for class name retrieval offers a reusable and organized method. It allows developers to encapsulate this functionality within the class itself, enhancing code maintainability and readability.
You can explicitly set a property on your class to store the class name. This custom property can be populated in the constructor.
For example:
class Product {
className: string;
constructor() {
this.className = this.constructor.name;
}
}
const myProduct = new Product();
console.log(`Class Name: ${myProduct.className}`); // Class Name: Product
Here’s how it works:
We define a Product
class with a className
property.
In the constructor, we set this.className
to this.constructor.name
. This means that when an instance of Product
is created, its className
property will be set to the name of the class, which in this case is "Product"
.
We then create an instance of the Product
class named myProduct
.
Finally, we use console.log()
to output the value of myProduct.className
, which will be "Product"
.
Class Name: Product
Conclusion
In TypeScript, dynamically retrieving the class name of an object is a versatile task with a variety of applications, including debugging, logging, and implementing dynamic behaviors. We explored several methods, each with its strengths and use cases.
First, we had the instanceof
Operator that provides a straightforward way to determine if an object is an instance of a specific class. While it’s primarily used for type-checking, it can also be used to retrieve the class name.
Then, we had the Object.getPrototypeOf()
method that allows us to retrieve the prototype of an object, providing access to the constructor function. It’s useful when we need more detailed information about an object’s inheritance chain.
Next, the constructor
Property refers to the constructor function used to create an instance. Accessing its name
property provides a quick way to get the class name.
Lastly, the Custom Property offers a structured and reusable approach, enhancing code maintainability and readability.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.