在 TypeScript 中获取类名
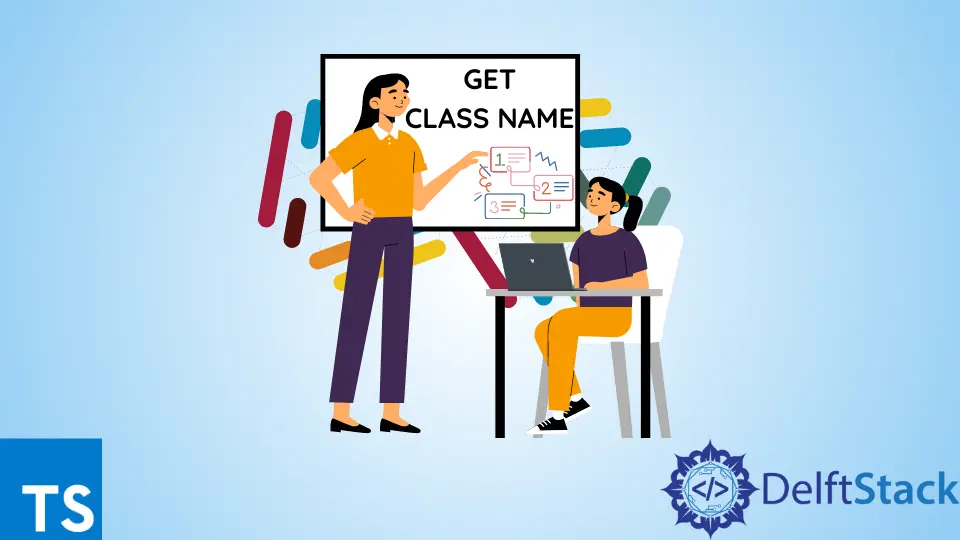
旧 JavaScript 更倾向于函数式编程。随着作为 JavaScript 超集 TypeScript 的发展,它开始支持面向对象的编程功能。
面向对象编程背后的基本术语是程序由交互的现实世界实体组成。
TypeScript 类和对象
TypeScript 支持带有 class
概念的面向对象编程。类是用于创建类实例的真实世界实体的蓝图。
TypeScript 类是从 ES6 引入的。一个类由字段、方法和构造函数组成。
class Animal {
// properties/fields
name: string;
color: string;
// constructor
constructor(name: string) {
this.name = name;
}
// methods/functions
eat(): void {
console.log("I am eating");
}
}
这些字段被定义为存储 Animal
对象的名称和颜色。TypeScript 构造函数用于初始化对象的属性。
它是一个可以参数化的特殊函数,如上例所示。
有了 Animal
类,就可以从中创建对象。TypeScript 使用 new
关键字从给定的类创建新对象。
由于 TypeScript 是一种强类型语言,新的 Animal
实例的类型将是 Animal
类型。
let dog: Animal = new Animal('rocky');
如你所见,创建 Animal
实例时已调用 Animal
类的构造函数。Animal
类构造函数接受一个字符串参数,我们必须在创建对象时传递它。
在运行时获取 TypeScript 对象的类型
在某些情况下,你需要在运行时知道 TypeScript 对象的类型。假设我们有一个名为 Vehicle
的类。
class Vehicle {
vehicleNo: string;
vehicleBrand: string
constructor(vehicleNo: string, vehicleBrand: string) {
this.vehicleNo = vehicleNo;
this.vehicleBrand = vehicleBrand;
}
}
让我们创建一个新的 Vehicle
对象,miniCar
。
let miniCar: Vehicle = new Vehicle('GH-123', 'AUDI');
miniCar
是 vehicle
对象,其类型是 Vehicle
。我们可以使用该类的 name
属性检查特定类的名称。
语法:
<class_name>.name
让我们检查类 Vehicle
的名称。
console.log(Vehicle.name);
输出:
Vehicle
TypeScript 对象的构造函数
如前所述,每个 TypeScript 类都包含一个构造函数,该构造函数可能是程序员编写的自定义构造函数或默认构造函数。它是一种特殊类型的 TypeScript 函数。
因此,我们可以获得对对象的 constructor
函数的引用。
语法:
Object.constructor
让我们获取对 miniCar
对象的构造函数的引用,如下所示。
let miniCarConstructorRef: any = miniCar.constructor;
接下来,我们将把 miniCarConstructorRef
变量值记录到控制台。
[Function: Vehicle]
正如预期的那样,它引用了 miniCar
对象的构造函数。
TypeScript 允许你使用 name
属性获取对象的构造函数名称。
语法:
Object.constructor.name
让我们使用上面的语法来获取 miniCar
对象的类名。
let classNameOfTheObject = miniCarConstructorRef.name;
最后,我们将把 classNameOfTheObject
变量值记录到控制台。
console.log(classNameOfTheObject);
输出:
Vehicle
这是检查对象所属类的推荐方法。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.