TypeScript에서 클래스 이름 가져오기
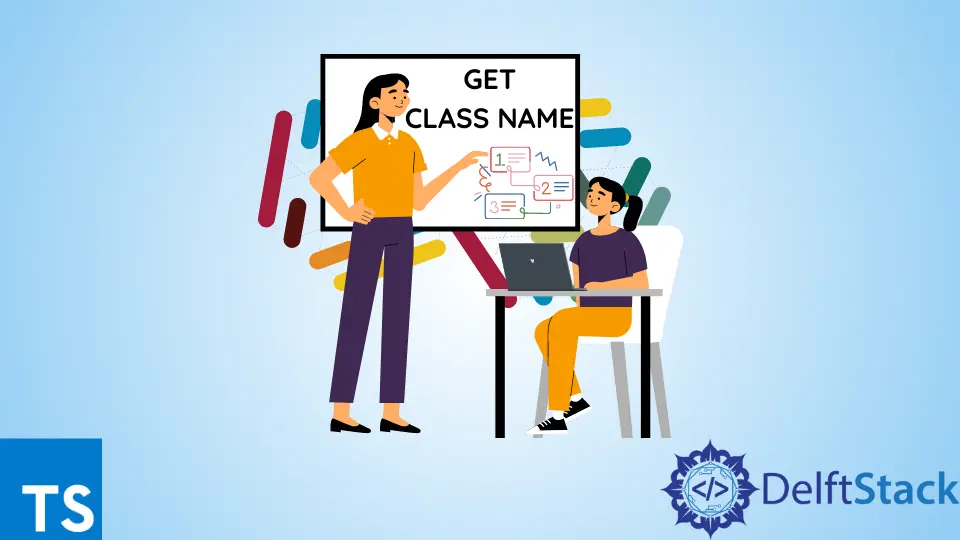
이전 JavaScript는 함수형 프로그래밍에 더 가깝습니다. JavaScript의 상위 집합인 TypeScript의 발전과 함께 객체 지향 프로그래밍 기능을 지원하기 시작했습니다.
객체 지향 프로그래밍의 기본 용어는 프로그램이 상호 작용하는 실제 개체로 구성된다는 것입니다.
TypeScript 클래스 및 객체
TypeScript는 class
개념으로 객체 지향 프로그래밍을 지원합니다. 클래스는 클래스 인스턴스를 만드는 데 사용되는 실제 엔터티의 청사진입니다.
TypeScript 클래스는 ES6부터 도입되었습니다. 클래스는 필드, 메서드 및 생성자로 구성됩니다.
class Animal {
// properties/fields
name: string;
color: string;
// constructor
constructor(name: string) {
this.name = name;
}
// methods/functions
eat(): void {
console.log("I am eating");
}
}
필드는 Animal
개체의 이름과 색상을 저장하도록 정의됩니다. TypeScript 생성자는 객체의 속성을 초기화하는 데 사용됩니다.
위의 예와 같이 매개변수화할 수 있는 특수 함수입니다.
Animal
클래스를 사용하면 해당 클래스에서 개체를 만들 수 있습니다. TypeScript는 new
키워드를 사용하여 주어진 클래스에서 새 객체를 생성합니다.
TypeScript는 강력한 형식의 언어이므로 새로운 Animal
인스턴스의 유형은 Animal
유형이 됩니다.
let dog: Animal = new Animal('rocky');
보시다시피 Animal
인스턴스를 생성할 때 Animal
클래스 생성자가 호출되었습니다. Animal
클래스 생성자는 하나의 문자열 매개변수를 허용하며 객체를 생성하는 동안 전달해야 했습니다.
런타임에 TypeScript 개체의 유형 가져오기
런타임에 TypeScript 객체의 유형을 알아야 하는 몇 가지 시나리오가 있습니다. Vehicle
이라는 클래스가 있다고 가정해 보겠습니다.
class Vehicle {
vehicleNo: string;
vehicleBrand: string
constructor(vehicleNo: string, vehicleBrand: string) {
this.vehicleNo = vehicleNo;
this.vehicleBrand = vehicleBrand;
}
}
새 Vehicle
개체 miniCar
를 만들어 보겠습니다.
let miniCar: Vehicle = new Vehicle('GH-123', 'AUDI');
miniCar
는 vehicle
객체이고 그 유형은 Vehicle
입니다. 해당 클래스의 name
속성을 사용하여 특정 클래스의 이름을 확인할 수 있습니다.
통사론:
<class_name>.name
Vehicle
클래스의 이름을 확인합시다.
console.log(Vehicle.name);
출력:
Vehicle
TypeScript 객체의 생성자
언급했듯이 각 TypeScript 클래스는 프로그래머가 작성한 사용자 정의 생성자 또는 기본 생성자일 수 있는 생성자로 구성됩니다. TypeScript 함수의 특별한 유형입니다.
따라서 객체의 constructor
함수에 대한 참조를 얻을 수 있습니다.
통사론:
Object.constructor
다음과 같이 miniCar
객체의 생성자에 대한 참조를 가져오겠습니다.
let miniCarConstructorRef: any = miniCar.constructor;
다음으로 miniCarConstructorRef
변수 값을 콘솔에 기록합니다.
[Function: Vehicle]
예상대로 miniCar
객체의 생성자를 참조합니다.
TypeScript를 사용하면 name
속성을 사용하여 개체의 생성자 이름을 가져올 수 있습니다.
통사론:
Object.constructor.name
위의 구문을 사용하여 miniCar
개체의 클래스 이름을 가져오겠습니다.
let classNameOfTheObject = miniCarConstructorRef.name;
마지막으로 classNameOfTheObject
변수 값을 콘솔에 기록합니다.
console.log(classNameOfTheObject);
출력:
Vehicle
이것은 객체가 속한 클래스를 확인하기 위해 권장되는 방법입니다.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.