Static Class in TypeScript
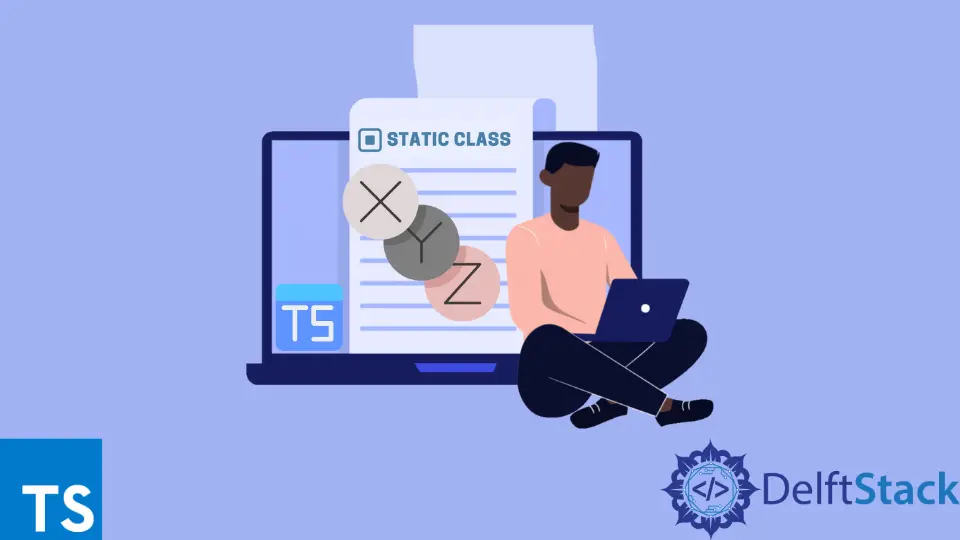
This tutorial will discuss the guidelines for defining a static class in TypeScript and the practices used among the developers and the TypeScript community.
Static Class in TypeScript
A static class
can be defined as a sealed class that cannot be inherited besides being inherited from an Object. static class
cannot be instantiated, which means you cannot create the instance variable from the Static Class reference.
In TypeScript, we don’t have a construct called the static class
, and a class with only one instance is often represented as a regular object. We don’t need static class
syntax in TypeScript because a top-level function (or even an object) will do the job just as well.
Code:
// Unnecessary "static" class
class staticClass {
static something() {
console.log("Something")
}
}
let obj = new staticClass();
// will produce an error.
console.log(obj.something)
// Preferred (alternative 1)
function something() {}
// Preferred (alternative 2)
const helperObject = {
dosomething() {
console.log("Helper object","1")
},
};
console.log(helperObject.dosomething(),"2")
Calling the static class something()
method will generate an error as it is static, while the alternative to this approach is we can use the object for performing the same thing. The output for both approaches produces the following outputs, respectively.
Output:
Since the static class
is not available, we look at how to define the alternate of the static class
.
Abstract Class in TypeScript
Abstract classes in TypeScript are used for inheritance where other classes may derive from them. We cannot instantiate the abstract class
means you cannot create an object of this class.
This will fulfill the criteria for the static class
in TypeScript. An abstract class
may include one or more abstract
methods or properties.
The class that extends an abstract class
made a contract that it will implement all its parent class methods or otherwise be declared abstract. The following example is an abstract
class that declares one abstract method called find()
and includes the display
method.
Code:
abstract class Person {
name: string;
constructor(name: string) {
this.name = name;
}
displayName(): void {
console.log(this.name);
}
abstract find(string: any): Person;
}
class Employee extends Person {
employeeCode: number;
constructor(name: string, code: number) {
super(name); // must call super()
this.employeeCode = code;
}
find(name: string): Person { // execute AJAX request to find an employee from a db
return new Employee(name, 1);
}
}
let newEmp: Person = new Employee("James", 100);
newEmp.displayName(); //James
let emp2: Person = newEmp.find('Steve');
The above code has a Person
class declared as abstract with one instance variable name
, standard method displayName()
, which displays the name
, and an abstract method implemented by any child class that extends the Person
Class.
After the object is created from the parent reference Person
class of the child class Employee
, the desired implementation is performed.
Output:
Let’s consider the following example in which it is shown that the abstract class can also have abstract properties.
Code:
abstract class Person {
abstract name: string;
display(): void{
console.log(this.name);
}
}
class Employee extends Person {
name: string;
empCode: number;
constructor(name: string, code: number) {
super(); // must call super()
this.empCode = code;
this.name = name;
}
}
let emp: Person = new Employee("James", 100);
emp.display(); //James
This 2nd code snippet produces the same output as the 1st code under the abstract class topic.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn