How to Interface Array of Objects in TypeScript
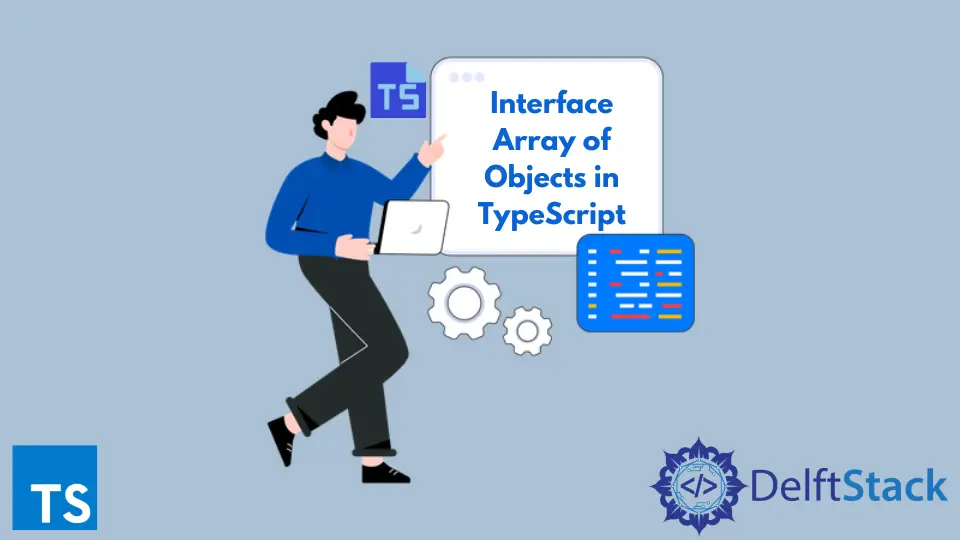
We will introduce how to interface an array of objects and the different methods to interface the array of an object with examples in TypeScript.
Interface Array of Objects in TypeScript
We defined an interface for an array of objects; we explained the interface for the type of each object. Put the array to be Type[]
.
For example:
# typescript
const arr: Worker[] =[];
All the objects added to the array must obey the type, or the type checker gives an error.
# typescript
interface student {
studentId: number;
studentName: string;
}
const arr: Employee[] = [
{ studentId: 1, studentName: 'Maham' },
{ studentId: 2, studentName: 'Maryam' },
];
In the above example, the type of an array of objects is used as an interface. Each object has an Id
of type number
and a name
of type string
.
We’re trying to add an object of a different type to an array that causes the type checker an error.
# typescript
interface Company {
CompanyId: number;
CompanyName: string;
[key: string]: any;
}
This can be very helpful when assigning the array as null. If we assign a null array, TypeScript supposes its type to be any[]
.
# typescript
const arr2: Student[] = [
{ StudentId: 1, StudentName: 'Maham' },
{ StudentId: 2, StudentName: 'Maryam', wage: 100 },
];
From the above example, we know we could add elements of any
type to an array, and we had not gotten any help from the type checker.
Rather we should express the type of all null arrays.
# typescript
interface Student {
StudentId: number;
StudentName: string;
}
const arr: Student[] = [
{ StudentId: 1, StudentName: 'Maham' },
{ StudentId: 2, StudentName: 'Maryam' },
];
arr.push({ Wage: 100 });
We can only add the objects that obey the Worker
type to the arr
array.
Suppose we do not have the names of all of the properties in the object before time. We used an index signature in the interface.
# typescript
const worker = [];
An index signature is useful when we cannot know all the names of a type’s properties and the value of the shape over time.
# typescript
interface Worker {
WorkerId: number;
WorkerName: string;
}
const arr: Worker[] = [];
From the above example, we can see the index signature {[key: string]: string}
. It expressed a key-value structure that, when indexed with a string
, returned to the value of the type string
.
We also know that each element in the array will have an id
of the type number
and the name
of the type string
. It can also have other characteristics.
Where the key is a string, and the value in it could be of any type.
While using this approach, we should expressly add all of the properties to the interface that we know about ahead of time.
It’s ideal to limit our use of the type checker as much as possible.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn