TypeScript 中的接口默认值
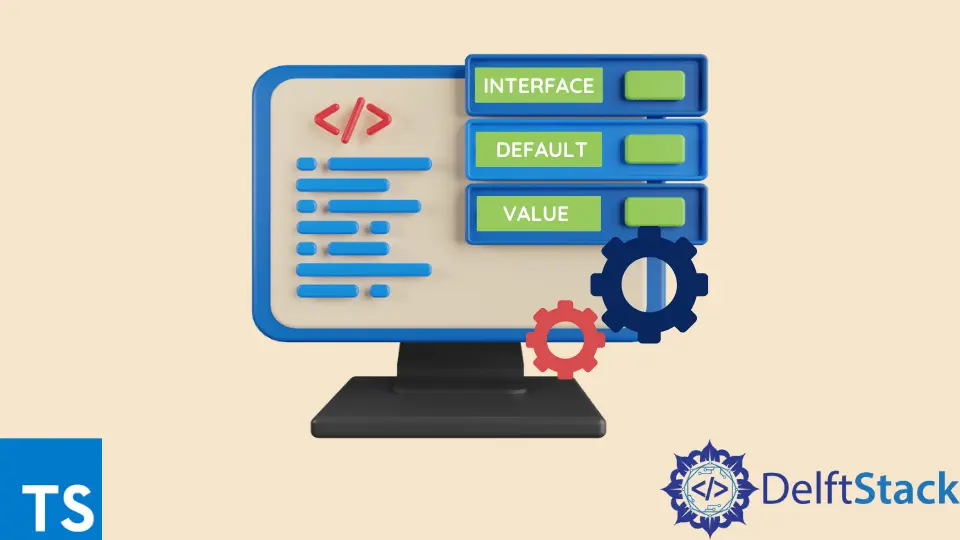
本教程提供了另一种在 TypeScript 中定义 Interface
默认值的解决方案。它提供了编码示例和输出以及有关接口是什么以及使用它们的原因的知识。
TypeScript 中的接口
是什么
TypeScript 有一个类型检查的核心原则,它专注于值的形状;有时,这称为鸭子类型或结构子类型。TypeScript 中的接口
扮演在代码内和项目外定义合约的角色。
以下是如何在 TypeScript 中定义 Interface
。
interface LabeledVal {
label: string;
}
function printLabelTraces(labeledObject: LabeledVal) {
console.log(labeledObject.label);
}
let myObject = { size: 10, label: "Size 10 Object" };
printLabelTraces(myObject);
上面的代码产生以下输出。
这个接口
描述了具有 label
属性的要求,即一个字符串。Interface
LabeledVal
是一个名称,我们可以用它来描述我们传递给 printLabelTraces
的对象实现的 Interface
,就像我们在其他语言中可能拥有的那样。
如果我们传递给函数的对象成功满足接口
中列出的要求,则它是允许的。
现在让我们看看如何在 TypeScript
中定义 Interface
默认值。
使用可选属性在 TypeScript
中设置 Interface
默认值
由于不需要所有属性都存在于实现接口的对象中,因此 Optional Properties 是流行的选项,可用于提供类似模式的选项包,其中函数的对象仅填充了几个属性。考虑以下示例的一个代码。
interface SquareConfig {
color?: string;
width?: number;
}
function Square(config: SquareConfig): { color: string; area: number } {
let newSquare = { color: "white", area: 100 };
if (config.color) {
newSquare.color = config.color;
}
if (config.width) {
newSquare.area = config.width * config.width;
}
return newSquare;
}
let SquareVal = Square({ color: "black" });
console.log(SquareVal);
在此代码中,SquareVal
从函数 Square
获取返回值,该函数具有 interface``SquareConfig
的参数类型,它具有一个可选值,可以根据使用情况分配或不分配。上面的代码产生以下输出。
使用可选属性编写接口的方式与使用 ?
编写普通接口相同。在属性名称末尾的声明中带有可选属性。这可以防止使用不属于接口的属性。
考虑 Square
中 color
属性的错误名称示例;我们会收到一条错误消息。
// @errors: 2551
interface SquareConfig {
color?: string;
width?: number;
}
function Square(config: SquareConfig): { color: string; area: number } {
let newSquare = { color: "white", area: 100 };
if (config.clor) {
// Error: Property 'clor' does not exist on type 'SquareConfig'
newSquare.color = config.clor;
}
if (config.width) {
newSquare.area = config.width * config.width;
}
return newSquare;
}
let SquareVal = Square({ color: "black" });
没有其他方法可以为接口或类型别名提供默认值,因为它们只是编译时的,而对于默认值,我们需要运行时支持。上述可选属性提供了该问题的替代解决方案。
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn