TypeScript 中的接口与类型
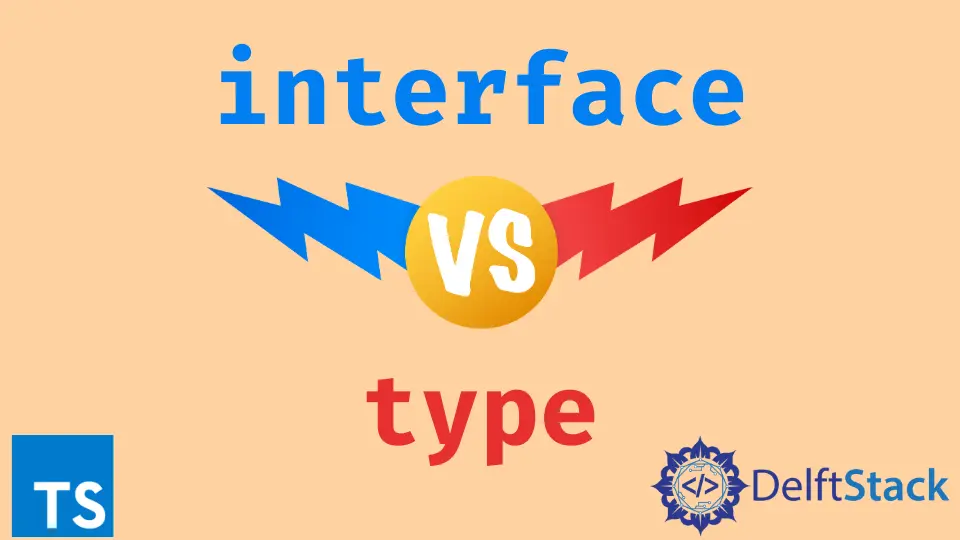
TypeScript 是 Microsoft 维护的一种编程语言,是 JavaScript 的超集,支持强类型。TypeScript 对于拥有大型代码库的组织变得非常有用。
TypeScript 有助于防止在 JavaScript 错误推断类型的情况下不可避免的运行时错误。
支持 TypeScript 的适当工具和 IDE 可以建议代码完成、检测类型中的错误、自动修复错误等等。TypeScript 可以在接口和类型的帮助下提供所有这些支持。
本文重点介绍接口和类型的各个方面以及何时使用它们。TypesSript 中有一些基本类型使用接口和类型被构造。
TypeScript 中接口和类型的相似之处
以下代码段显示了 TypeScript 中的接口示例。
interface Point {
x : number;
y : number;
}
interface Circle{
center : Point;
radius : number;
}
const circle : Circle = {
center : {
x : 2,
y : 3
},
radius: 5
}
console.log(`Circle = [center :{${circle.center.x}, ${circle.center.y}}, \
radius : ${circle.radius} ]`)
现在我们可以使用 Types 实现相同的构造。以下代码段显示了这一点。
type Point = {
x : number;
y : number;
}
type Circle = {
center : Point;
radius : number;
}
两种构造都将给出相同的输出。
输出:
Circle = [center :{2, 3}, radius : 5 ]
属性名称中的简单拼写将使编译失败并防止运行时错误。
TypeScript 中接口和类型的区别
类型和接口都可以扩展其他类型,从而支持强类型 OOP 范式。
但是,扩展的语法是不同的。以下代码段显示了这是如何实现的。
type PointType = {
x : number
y : number
}
interface RadiusType {
radius : number
}
// valid
type Circle = RadiusType & PointType;
// valid
interface Circle extends RadiusType, PointType {}
// use it as
const circle : Circle = {
x : 3,
y : 4,
radius : 3
};
TypeScript 中的接口和类充当静态蓝图,因此不能在类型定义中使用 union
运算符扩展类型别名。例如:
type Animal = string;
type Living = bool;
type AnimalOrLiving = Animal | Living;
// will show error in compilation
interface Creature extends AnimalOrLiving {}
// will show error in compilation
class Creature extends AnimalOrLiving {}
最后,TypeScript 中的接口支持声明合并,这不适用于类型别名。多次声明的接口会合并为一个,而多次声明类型别名会出错。
它在生成公共 API 的情况下变得有用,以便消费者可以通过再次声明 API 来进一步添加 API。例如:
interface Animal {
type: string
}
interface Animal {
legs : number
}
interface Animal {
eyes : number
}
// use it as
const Dog : Animal = {
type : 'dog',
legs : 2,
eyes : 2
}
结论
因此,总而言之,在 TypeScript 中使用类型和接口时需要牢记一些细微的差异。
在设计 API 库或声明对象时首选接口。在声明常量和作为函数的返回类型时,类型更受青睐。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
相关文章 - TypeScript Interface
- TypeScript 中的接口类型检查
- 在 TypeScript 中使用嵌套属性扩展接口
- TypeScript 中的接口与类
- TypeScript 中的接口默认值
- 在 TypeScript 中从接口创建对象