TypeScript 中的介面型別檢查
- TypeScript 中的型別保護
-
在 TypeScript 中使用
typeof
來檢查型別 -
在 TypeScript 中使用
instanceof
-
在 TypeScript 中使用
in
關鍵字來檢查型別
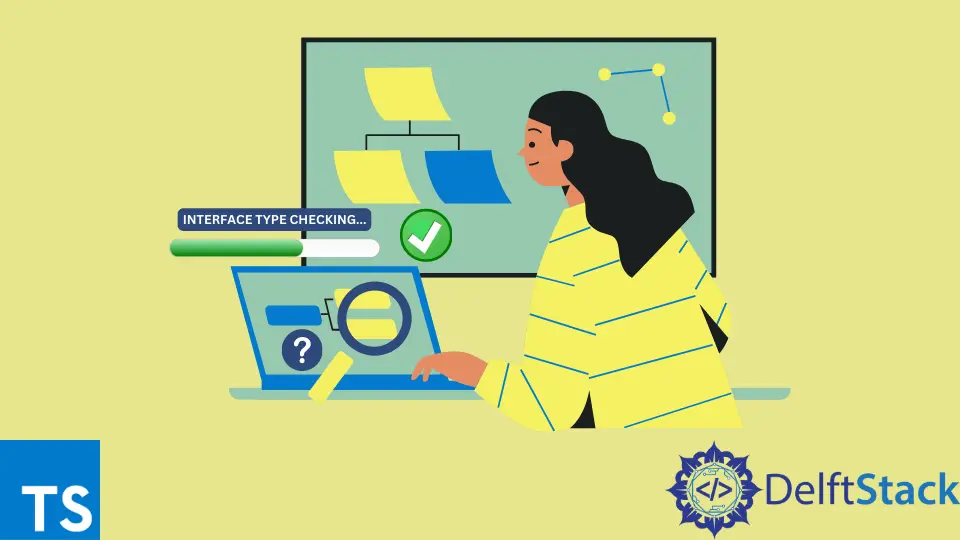
介面是 TypeScript 的重要組成部分,用於將型別與 TypeScript 中的變數和元素相關聯,以確保型別安全,從而避免執行時錯誤。類和其他介面可以實現介面,因此這可以用於實際程式碼中的動態行為。
因此,有時可能需要知道實際的介面型別以確保型別安全,就像 Java 中的 instanceof
關鍵字一樣。本教程將演示如何檢查介面的型別以確保型別安全。
TypeScript 中的型別保護
型別保護是對 TypeScript 中存在的不同型別的檢查,以確保型別安全。它是條件語句的形式。
當某種型別是一種或多種型別的組合時尤其需要。可以使用各種關鍵字來檢查介面,例如 typeof
、instanceof
、in
,甚至可以製作自定義型別保護。
interface Animal {
name : string;
legs : number;
eyes : number;
}
interface Dog extends Animal {
bark : () => void
}
interface Cat extends Animal {
meow : () => void;
}
function getAnimal() : Dog | Cat {
let choice = Math.random();
if ( choice > 0.5){
const dog : Dog = {
name : 'Labrador',
legs : 4,
eyes : 2,
bark : () => console.log("Woof")
}
return dog;
}else {
const cat : Cat = {
name : 'Labrador',
legs : 4,
eyes : 2,
meow : () => console.log("Meow")
}
return cat;
}
}
const animal = getAnimal();
// Property 'meow' does not exist on type 'Dog | Cat'.
animal.meow();
// Property 'bark' does not exist on type 'Dog | Cat'.
animal.bark();
在上面的示例中,屬性 meow
或 bark
函式無法解析,因為 TypeScript 編譯器不確定要推斷什麼。getAnimal
函式返回兩種型別的聯合,Dog | Cat
,這會使編譯器感到困惑。
必須引入型別保護或型別檢查以使編譯器理解適當的型別。
if ( 'meow' in animal){
animal.meow();
} else if ( 'bark' in animal){
animal.bark();
}
以上是如何強制執行型別檢查以確保型別安全的示例。meow
和 bark
屬性是否存在於 animal
物件中並被相應地呼叫。
在 TypeScript 中使用 typeof
來檢查型別
typeof
關鍵字可用於確定變數的型別;但是,它的範圍非常有限。它可用於檢查原始型別。
typeof
關鍵字返回的值可以是 string
、number
、bigint
、boolean
、symbol
、undefined
、object
或 function
。
typeof
關鍵字將所有複雜型別和 null
值作為物件返回。
const displayBill = ( amount : string | number ) => {
if ( typeof amount === 'string') {
amount = Number(amount);
}
let tax = (18.5 * amount) / 100;
console.log('Bill : '+ amount + " $");
console.log('Tax : '+ tax + " $");
console.log('Total Payable : '+ (amount + tax) + " $");
}
displayBill(23.5);
輸出:
"String conversion being done!"
"Bill : 23.5 $"
"Tax : 4.3475 $"
"Total Payable : 27.8475 $"
因此在上面的例子中,typeof
關鍵字被用來檢查變數 amount
的型別,並且在檢查之後進行了轉換。這個檢查對於計算 tax
變數是必要的,它要求 amount
是 number
型別。
在 TypeScript 中使用 instanceof
instanceof
關鍵字檢查與某些類對應的變數。以下型別保護用於那些被例項化為類物件的變數。
class User {
name : string;
amountDue : number;
constructor( name : string, amount : number){
this.name = name;
this.amountDue = amount;
}
}
class UserCredit extends User {
constructor( user : User) {
super(user.name, user.amountDue);
}
generateCredit(amount : number) {
this.amountDue += amount;
return this.amountDue;
}
}
class UserDebit extends User {
constructor( user : User) {
super(user.name, user.amountDue);
}
settleDebt(){
this.amountDue = 0;
}
}
const TransactionSystem = () => {
const user : User = {
name : 'Alex',
amountDue : 0
}
const option = Math.random();
if ( option > 0.5) {
// settle debts
const userDebt = new UserDebit(user);
userDebt.settleDebt();
return userDebt;
} else {
// increase credit
const userCredit = new UserCredit(user);
userCredit.generateCredit(500);
return userCredit;
}
}
const UserTransaction = TransactionSystem();
if ( UserTransaction instanceof UserDebit) {
console.log('Credit balance successfully debited');
} else if (UserTransaction instanceof UserCredit) {
console.log("Credit limit increased")
}
在 TypeScript 中使用 in
關鍵字來檢查型別
in
可用於檢查某個屬性是否存在於型別或介面中,因此可以與諸如 if-else
之類的條件語句一起使用來檢查型別,從而採取行動並執行進一步的操作。下面顯示瞭如何實現這一點的示例。
interface Person {
name : string;
age : number;
}
const person : Person = {
name : "Alex",
age : 30
}
console.log("name" in person); // true
console.log("address" in person); // false