TypeScript의 인터페이스 유형 확인
- TypeScript의 유형 가드
-
typeof
를 사용하여 TypeScript에서 유형 확인 -
TypeScript에서
instanceof
사용 -
in
키워드를 사용하여 TypeScript에서 유형 확인
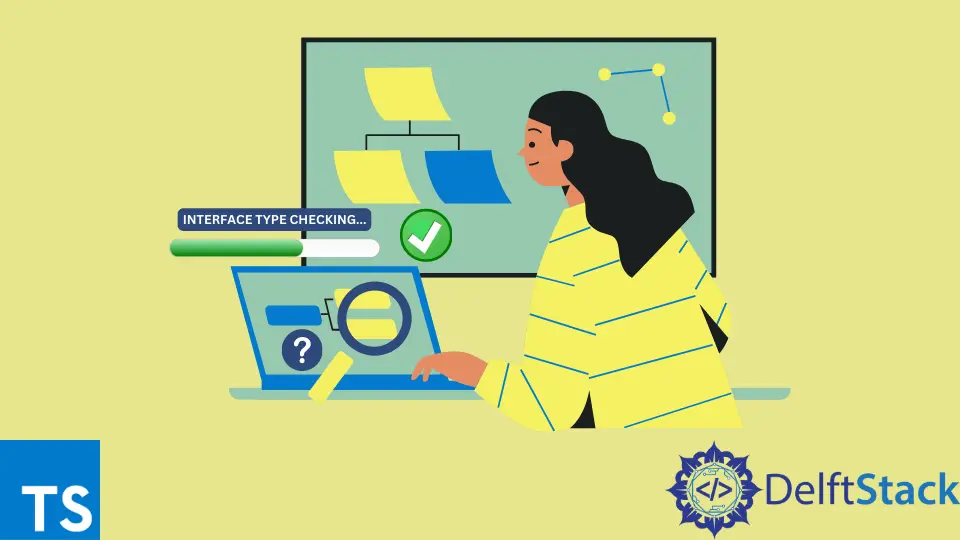
인터페이스는 유형 안전성을 보장하고 런타임 오류를 피하기 위해 TypeScript의 변수 및 요소에 유형을 연결하는 TypeScript의 중요한 부분입니다. 클래스 및 기타 인터페이스는 인터페이스를 구현할 수 있으므로 실제 코드에서 동적 동작에 사용할 수 있습니다.
따라서 Java의 instanceof
키워드와 마찬가지로 유형 안전성을 보장하기 위해 실제 인터페이스 유형을 알아야 할 수도 있습니다. 이 튜토리얼은 인터페이스 유형을 확인하여 유형 안전성을 보장하는 방법을 보여줍니다.
TypeScript의 유형 가드
타입 가드는 타입 안전성을 보장하기 위해 TypeScript에 존재하는 다양한 타입에 대한 검사입니다. 조건문 형식으로 되어 있습니다.
특정 유형이 하나 이상의 유형으로 구성된 경우 특히 필요합니다. typeof
, instanceof
, in
과 같이 인터페이스를 확인할 수 있는 다양한 키워드를 사용할 수 있으며 사용자 정의 유형 가드를 만들 수도 있습니다.
interface Animal {
name : string;
legs : number;
eyes : number;
}
interface Dog extends Animal {
bark : () => void
}
interface Cat extends Animal {
meow : () => void;
}
function getAnimal() : Dog | Cat {
let choice = Math.random();
if ( choice > 0.5){
const dog : Dog = {
name : 'Labrador',
legs : 4,
eyes : 2,
bark : () => console.log("Woof")
}
return dog;
}else {
const cat : Cat = {
name : 'Labrador',
legs : 4,
eyes : 2,
meow : () => console.log("Meow")
}
return cat;
}
}
const animal = getAnimal();
// Property 'meow' does not exist on type 'Dog | Cat'.
animal.meow();
// Property 'bark' does not exist on type 'Dog | Cat'.
animal.bark();
위의 예에서 속성 meow
또는 bark
기능은 TypeScript 컴파일러가 무엇을 유추해야 할지 확신할 수 없기 때문에 확인할 수 없습니다. getAnimal
함수는 Dog | 컴파일러를 혼동시키는 Cat
.
컴파일러가 적절한 유형을 이해할 수 있도록 유형 가드 또는 유형 검사를 도입해야 합니다.
if ( 'meow' in animal){
animal.meow();
} else if ( 'bark' in animal){
animal.bark();
}
위의 내용은 형식 안전성을 보장하기 위해 형식 검사를 시행하는 방법의 예입니다. meow
및 bark
속성이 animal
개체에 있는지 확인하고 그에 따라 호출됩니다.
typeof
를 사용하여 TypeScript에서 유형 확인
typeof
키워드는 변수 유형을 결정하는 데 사용할 수 있습니다. 그러나 범위가 매우 제한적입니다. 원시 유형을 확인하는 데 사용할 수 있습니다.
typeof
키워드에 의해 반환된 값은 string
, number
, bigint
, boolean
, symbol
, undefined
, object
또는 function
일 수 있습니다.
typeof
키워드는 모든 복합 유형과 null
값을 객체로 반환합니다.
const displayBill = ( amount : string | number ) => {
if ( typeof amount === 'string') {
amount = Number(amount);
}
let tax = (18.5 * amount) / 100;
console.log('Bill : '+ amount + " $");
console.log('Tax : '+ tax + " $");
console.log('Total Payable : '+ (amount + tax) + " $");
}
displayBill(23.5);
출력:
"String conversion being done!"
"Bill : 23.5 $"
"Tax : 4.3475 $"
"Total Payable : 27.8475 $"
따라서 위의 예에서는 typeof
키워드를 사용하여 amount
변수의 유형을 확인하고 확인 후 변환을 수행했습니다. 이 확인은 amount
이 number
유형이어야 하는 tax
변수를 계산하는 데 필요합니다.
TypeScript에서 instanceof
사용
instanceof
키워드는 일부 클래스에 해당하는 변수를 확인합니다. 다음 유형 보호는 클래스 개체로 인스턴스화되는 해당 변수에 사용됩니다.
class User {
name : string;
amountDue : number;
constructor( name : string, amount : number){
this.name = name;
this.amountDue = amount;
}
}
class UserCredit extends User {
constructor( user : User) {
super(user.name, user.amountDue);
}
generateCredit(amount : number) {
this.amountDue += amount;
return this.amountDue;
}
}
class UserDebit extends User {
constructor( user : User) {
super(user.name, user.amountDue);
}
settleDebt(){
this.amountDue = 0;
}
}
const TransactionSystem = () => {
const user : User = {
name : 'Alex',
amountDue : 0
}
const option = Math.random();
if ( option > 0.5) {
// settle debts
const userDebt = new UserDebit(user);
userDebt.settleDebt();
return userDebt;
} else {
// increase credit
const userCredit = new UserCredit(user);
userCredit.generateCredit(500);
return userCredit;
}
}
const UserTransaction = TransactionSystem();
if ( UserTransaction instanceof UserDebit) {
console.log('Credit balance successfully debited');
} else if (UserTransaction instanceof UserCredit) {
console.log("Credit limit increased")
}
in
키워드를 사용하여 TypeScript에서 유형 확인
in
은 유형 또는 인터페이스에 특정 속성이 있는지 확인하는 데 사용할 수 있으므로 if-else
와 같은 조건문과 함께 사용하여 유형을 확인하고 추가 작업을 수행하고 실행할 수 있습니다. 다음은 이를 달성할 수 있는 방법의 예를 보여줍니다.
interface Person {
name : string;
age : number;
}
const person : Person = {
name : "Alex",
age : 30
}
console.log("name" in person); // true
console.log("address" in person); // false