How to Write a List to a File With Python
- Use a Loop to Write a List to a File in Python
-
Use the
Pickle
Module to Write a List to a File in Python -
Use
join()
Method to Write a List to a File
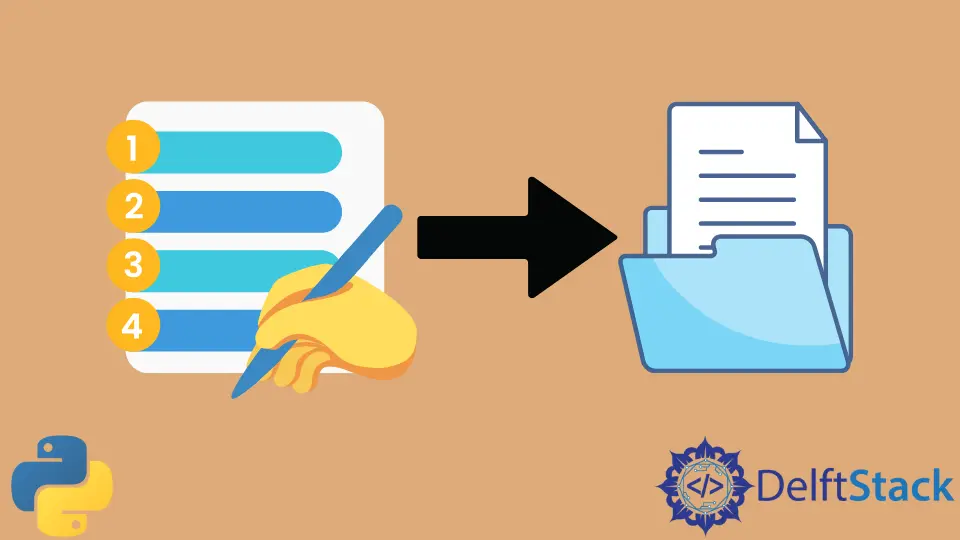
This tutorial explains how to write a list to a file with Python. Since there are multiple ways of writing a list to a file, the tutorial also lists various example codes to explain them further.
Use a Loop to Write a List to a File in Python
Using a loop to write a list to a file is a very trivial and most used approach. A loop is used to iterate over the list items, and the write()
method is used to write list items to the file.
We use the open()
method to open the destination file. The mode of opening the file shall be w
that stands for write
.
An example code is given below:
listitems = ["ab", "cd", "2", "6"]
with open("abc.txt", "w") as temp_file:
for item in listitems:
temp_file.write("%s\n" % item)
file = open("abc.txt", "r")
print(file.read())
Output:
{'6','2', 'ab', 'cd'}
For Python 2.x, you also use the following approach:
listitems = ["ab", "cd", "2", "6"]
with open("xyz.txt", "w") as temp_file:
for item in listitems:
print >> temp_file, item
Both of the codes will result in writing a list to a file with Python.
Use the Pickle
Module to Write a List to a File in Python
The Pickle
module of Python is used to serialize or de-serialize a Python object structure. If you want to serialize a list for later use in the same Python file, pickle
could be used. As the pickle
module implements binary protocols, so the file shall also be opened in the binary writing mode - wb
.
pickle.dump()
method is used to write a list to a file. It takes the list and reference of the file as its parameters.
An example code for this approach is given as:
import pickle
listitems = ["ab", "cd", "2", "6"]
with open("outputfile", "wb") as temp:
pickle.dump(listitems, temp)
with open("outfile", "rb") as temp:
items = pickle.load(temp)
print(items)
Output:
{'ab','cd', '6', '2'}
Use join()
Method to Write a List to a File
Another more straightforward approach to writing a list to a file in Python is by using the join()
method. It takes the items of the list as input.
An example code to use this method is shown below:
items = ["a", "b", "c", "d"]
with open("outputfile", "w") as opfile:
opfile.write("\n".join(items))
Use JSON
Module to Write a List to a File
The use of the pickle
module is very specific in Python. JSON makes different programs more efficient and easy to understand. JSON
module is used to write mixed variable types to a file.
It uses the dump()
method that takes a list of elements and references to the file as its input.
An example code is given as:
import json
itemlist = [21, "Tokyo", 3.4]
with open("opfile.txt", "w") as temp_op:
json.dump(itemlist, temp_op)
print(templist)
Output:
[21, "Tokyo", 3.4]
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python