如何用 Python 將列表寫入檔案
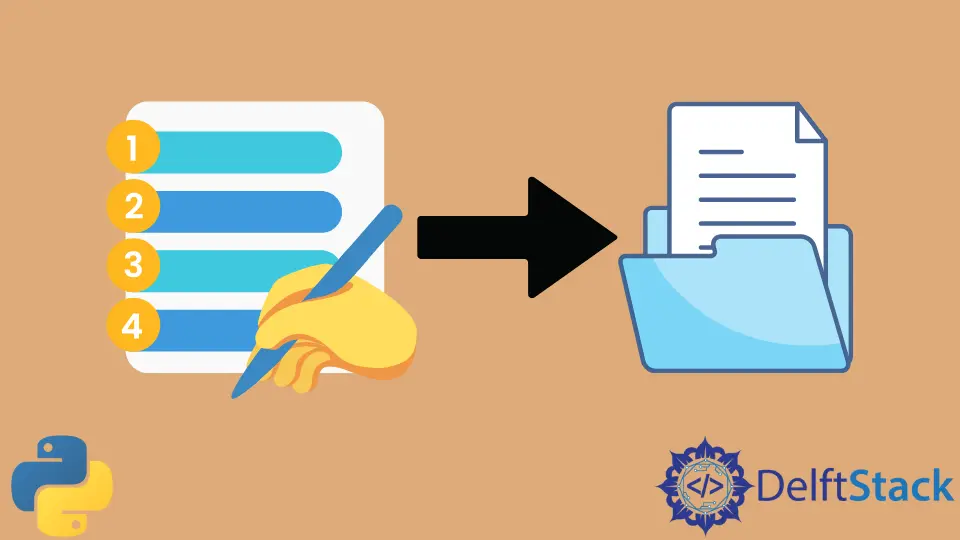
本教程說明了如何用 Python 將一個列表寫到檔案中。由於有多種方法可以將一個列表寫入檔案,本教程還列出了各種示例程式碼來進一步解釋它們。
在 Python 中使用迴圈把一個列表寫到檔案上
使用迴圈將列表寫到檔案中是一種非常瑣碎也是最常用的方法。迴圈用來迭代列表項,而 write()
方法則用來將列表項寫入檔案。
我們使用 open()
方法來開啟目標檔案。開啟檔案的模式應該是 w
,代表 write
。
示例程式碼如下:
listitems = ["ab", "cd", "2", "6"]
with open("abc.txt", "w") as temp_file:
for item in listitems:
temp_file.write("%s\n" % item)
file = open("abc.txt", "r")
print(file.read())
輸出:
{'6','2', 'ab', 'cd'}
對於 Python 2.x,你也可以使用下面的方法。
listitems = ["ab", "cd", "2", "6"]
with open("xyz.txt", "w") as temp_file:
for item in listitems:
print >> temp_file, item
這兩個程式碼的結果都是用 Python 把列表寫到一個檔案中。
在 Python 中使用 Pickle
模組把一個列表寫入一個檔案
Python 的 Pickle
模組用於序列化或去序列化一個 Python 物件結構。如果你想序列化一個列表,以便以後在同一個 Python 檔案中使用,可以使用 pickle
。由於 pickle
模組實現了二進位制協議,所以檔案也應該以二進位制寫入模式-wb
開啟。
pickle.dump()
方法用於將一個列表寫入檔案。它把列表和檔案的引用作為引數。
這種方法的示例程式碼如下:
import pickle
listitems = ["ab", "cd", "2", "6"]
with open("outputfile", "wb") as temp:
pickle.dump(listitems, temp)
with open("outfile", "rb") as temp:
items = pickle.load(temp)
print(items)
輸出:
{'ab','cd', '6', '2'}
使用 join()
方法將列表寫入檔案
另一種更直接的方法是使用 join()
方法在 Python 中把一個列表寫入檔案。它將列表中的元素作為輸入。
下面是一個使用該方法的示例程式碼。
items = ["a", "b", "c", "d"]
with open("outputfile", "w") as opfile:
opfile.write("\n".join(items))
使用 JSON
模組將列表寫入檔案
在 Python 中,pickle
模組的使用非常特殊。JSON 使不同的程式更加高效和容易理解。JSON
模組用於將混合變數型別寫入檔案。
它使用 dump()
方法,將元素列表和對檔案的引用作為輸入。
示例程式碼如下:
import json
itemlist = [21, "Tokyo", 3.4]
with open("opfile.txt", "w") as temp_op:
json.dump(itemlist, temp_op)
print(templist)
輸出:
[21, "Tokyo", 3.4]
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn相關文章 - Python List
- 在 Python 中將字典轉換為列表
- 從 Python 列表中刪除某元素的所有出現
- 在 Python 中從列表中刪除重複項
- 如何在 Python 中獲取一個列表的平均值
- Python 列表方法 append 和 extend 之間有什麼區別
- 如何在 Python 中將列表轉換為字串