How to Save Dictionary to JSON in Python
-
Save Dictionary to JSON Using the
pickle.dump()
Method in Python -
Save Dictionary to JSON Using the
json.dump()
Method in Python
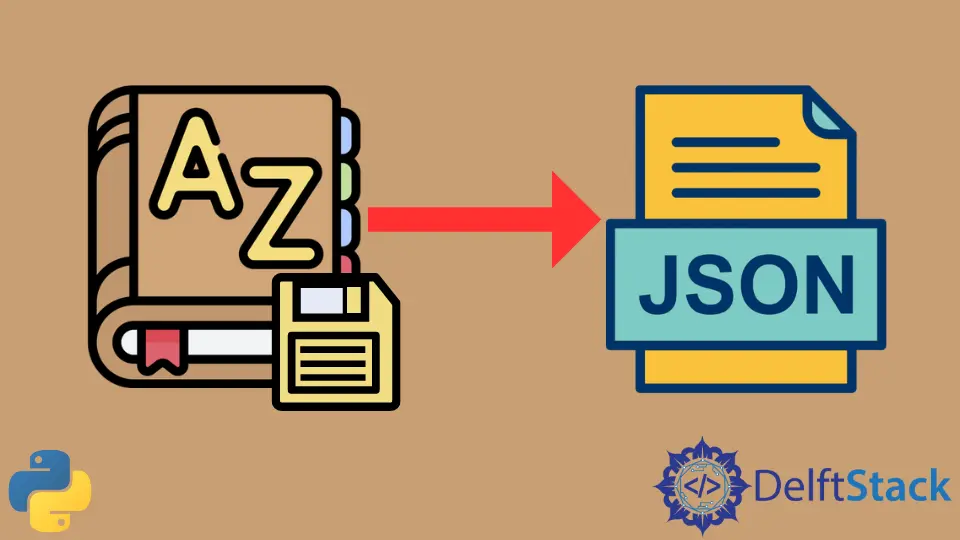
This tutorial will explain various methods to save a dictionary as a JSON file in Python. The JSON format is a prevalent lightweight file format; it is primarily used to store and transfer data between web servers and applications. It is a complete language-independent file format and is easy to understand for a human.
Save Dictionary to JSON Using the pickle.dump()
Method in Python
The dump(obj, file, ..)
method of the pickle
module writes the data object obj
to the opened file object file
. To save the dictionary to JSON format, we will need the file object of the .json
file and the dictionary that we need to save and pass them to the dump()
method.
We can also load the saved dictionary from the .json
file using the load()
method of the pickle
library. The pickle.load(file, ..)
method reads the file and returns the object of data type used to save the data, like a dictionary, list or set, etc.
The example code below demonstrates how to save dictionary as JSON file in Python using the dump()
method:
import pickle
my_dict = {"Ali": 9, "Sid": 1, "Luna": 7, "Sim": 12, "Pooja": 4, "Jen": 2}
with open("data.json", "wb") as fp:
pickle.dump(my_dict, fp)
with open("data.json", "rb") as fp:
data = pickle.load(fp)
print(data)
print(type(data))
Output:
{'Ali': 9, 'Sid': 1, 'Luna': 7, 'Sim': 12, 'Pooja': 4, 'Jen': 2}
<class 'dict'>
Save Dictionary to JSON Using the json.dump()
Method in Python
The dump(obj, file, ..)
method of the json
module also writes the data object obj
to the open file object file
. And the load(file, ..)
method of the json
module also reads the file and returns the object of the data type using which data was saved. In our case, it will be a dictionary.
As explained above, to save the dictionary as a JSON file, we will need the opened file object of the .json
file to save the dictionary data into it. It is also needed to load the data from the .json
file.
The code example below demonstrates how to save and load the dictionary to JSON file in Python using the json.dump()
and json.load()
methods:
import json
my_dict = {"Ali": 9, "Sid": 1, "Luna": 7, "Sim": 12, "Pooja": 4, "Jen": 2}
with open("data.json", "w") as fp:
json.dump(my_dict, fp)
with open("data.json", "r") as fp:
data = json.load(fp)
print(data)
print(type(data))
Output:
{'Ali': 9, 'Sid': 1, 'Luna': 7, 'Sim': 12, 'Pooja': 4, 'Jen': 2}
<class 'dict'>
pickle
and json
methods, as shown in the above code example, is that pickle
methods require file objects in binary mode to read and write, and json
methods require file objects in simple read and write mode.Related Article - Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- How to Convert a Dictionary to a List in Python
- How to Get All the Files of a Directory
- How to Find Maximum Value in Python Dictionary
- How to Sort a Python Dictionary by Value
- How to Merge Two Dictionaries in Python 2 and 3