How to Read a Text File Into a List in Python
- Method 1: Using the readlines() Function
- Method 2: Using a List Comprehension
- Method 3: Using the read() Method
- Method 4: Reading Large Files Using a Loop
- Conclusion
- FAQ
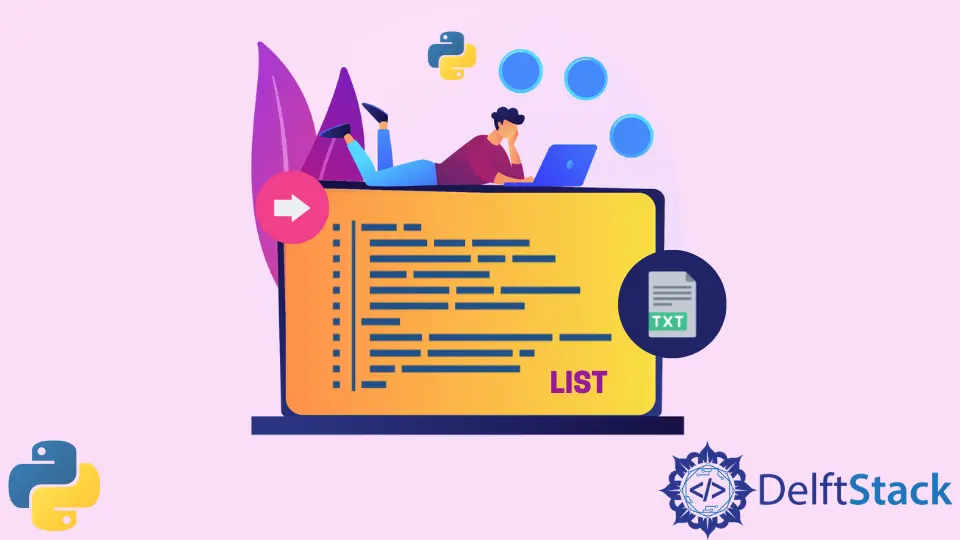
Reading a text file and converting its contents into a list is a fundamental task in Python programming. Whether you’re analyzing data, processing logs, or simply managing text files, knowing how to effectively read files is crucial.
In this tutorial, we will explore various methods to read a text file into a Python list. Each method will be accompanied by clear code examples and detailed explanations to help you understand the process thoroughly. By the end of this guide, you’ll have the tools and knowledge to manipulate text files efficiently in Python, enhancing your programming skills and project outcomes.
Method 1: Using the readlines() Function
One of the simplest ways to read a text file into a list in Python is by using the readlines()
function. This method reads each line of the file and returns a list where each element corresponds to a line in the file. Here’s how you can do it:
file_path = 'example.txt'
with open(file_path, 'r') as file:
lines = file.readlines()
print(lines)
Output:
['First line\n', 'Second line\n', 'Third line\n']
In this example, we first specify the path to the text file we want to read. The with open(file_path, 'r') as file:
statement opens the file in read mode. Using readlines()
, we read all the lines from the file, which are stored in the lines
variable as a list. Each line retains its newline character (\n
). This method is straightforward and efficient for smaller files, but keep in mind that it reads the entire file into memory, which might not be ideal for very large files.
Method 2: Using a List Comprehension
Another efficient way to read a text file into a list is by utilizing a list comprehension. This method allows you to read the file line by line and strip away any unwanted whitespace, including newline characters. Here’s how it works:
file_path = 'example.txt'
with open(file_path, 'r') as file:
lines = [line.strip() for line in file]
print(lines)
Output:
['First line', 'Second line', 'Third line']
In this code snippet, we again open the file using the with
statement. However, instead of using readlines()
, we iterate directly over the file object within a list comprehension. Each line is processed by line.strip()
, which removes any leading and trailing whitespace, including newline characters. This results in a cleaner list of strings, making it particularly useful when you need to manipulate or analyze the text data further. This method is efficient and works well for larger files since it reads one line at a time.
Method 3: Using the read() Method
The read()
method can also be used to read the entire contents of a file into a single string, which can then be split into a list. This method is ideal when you want to process the entire content at once before converting it into a list. Here’s how you can do it:
file_path = 'example.txt'
with open(file_path, 'r') as file:
content = file.read()
lines = content.splitlines()
print(lines)
Output:
['First line', 'Second line', 'Third line']
In this example, we first read the entire content of the file into the content
variable using file.read()
. We then use the splitlines()
method to break the string into a list of lines. This approach is beneficial when you need to perform operations on the entire content before splitting it into individual lines. However, be cautious with large files, as this method reads the entire file into memory, which can lead to performance issues if the file size is substantial.
Method 4: Reading Large Files Using a Loop
For large text files, reading the entire file at once may not be efficient. Instead, you can read the file line by line using a loop. This method allows you to process each line individually, minimizing memory usage. Here’s how to implement this:
file_path = 'example.txt'
lines = []
with open(file_path, 'r') as file:
for line in file:
lines.append(line.strip())
print(lines)
Output:
['First line', 'Second line', 'Third line']
In this code snippet, we initialize an empty list called lines
. We then open the file and iterate through it line by line using a for
loop. Each line is stripped of whitespace using line.strip()
before appending it to the lines
list. This method is particularly advantageous for large files, as it only loads one line into memory at a time, making it much more memory-efficient.
Conclusion
Reading a text file into a list in Python is a straightforward process that can be accomplished in several ways. Whether you choose to use readlines()
, a list comprehension, the read()
method, or a loop, each approach has its advantages depending on the size of the file and your specific needs. By mastering these techniques, you can efficiently handle text files in your Python projects, paving the way for more complex data manipulation and analysis tasks. With practice, you’ll find these methods become second nature, enhancing your programming toolkit.
FAQ
-
How do I read a text file without newline characters?
You can use thestrip()
method while reading each line to remove newline characters and other whitespace. -
What happens if the file does not exist?
If you attempt to open a file that does not exist, Python will raise aFileNotFoundError
. -
Can I read a text file in binary mode?
Yes, you can open a file in binary mode by using ‘rb’ instead of ‘r’ in theopen()
function. -
Is it possible to read large files without loading them into memory?
Yes, you can read large files line by line using a loop, which minimizes memory usage. -
What is the difference between readlines() and read()?
readlines()
returns a list of lines, whileread()
returns the entire file content as a single string.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python