NumPy numpy.loadtxt() Function
-
Syntax of
numpy.loadtxt()
: -
Example Codes: NumPy Read
txt
File Usingnumpy.loadtxt()
Function -
Example Codes: Set
dtype
Parameter innumpy.loadtxt()
Function While Reading thetxt
File -
Example Codes: Set
delimiter
Parameter innumpy.loadtxt()
Function While Readingtxt
Files -
Example Codes: Set
usecols
Parameter innumpy.loadtxt()
Function While Reading thetxt
File -
Example Codes: Set
unpack
Parameter innumpy.loadtxt()
Function While Readingtxt
Files
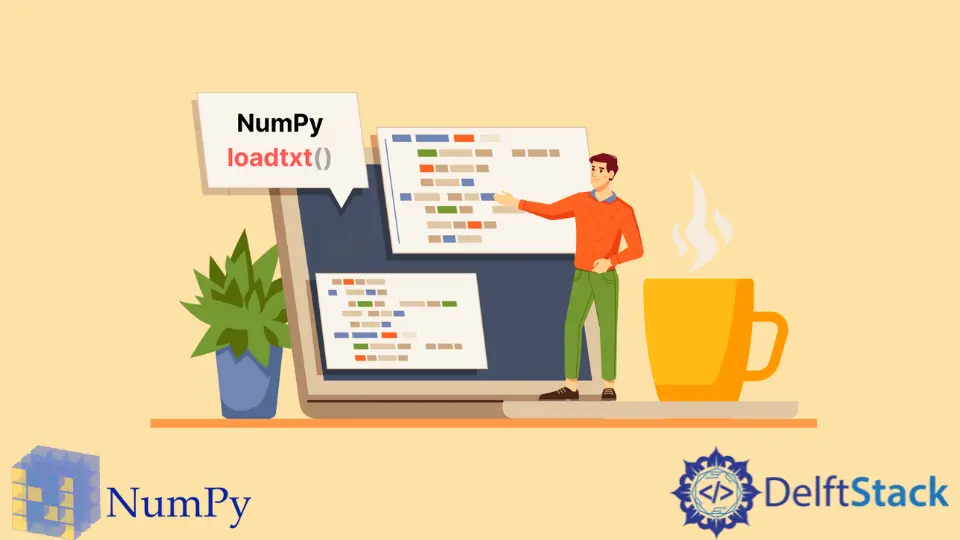
Python Numpy numpy.loadtxt()
function loads data from a text file and provides fast approach for simple text files.
Syntax of numpy.loadtxt()
:
numpy.loadtxt(fname,
dtype= < class 'float' > ,
comments='#',
delimiter=None,
converters=None,
skiprows=0,
usecols=None,
unpack=False,
ndmin=0,
encoding='bytes',
max_rows=None)
Parameters
fname |
Path of txt file to be imported |
dtype |
Data type of the resulting array |
comments |
Characters or list of characters used to indicate the start of a comment |
delimiter |
Delimiter to use for parsing the content of txt file |
converters |
Dictionary mapping column number to a function that will parse the column string into the desired value. |
skiprows |
Which row/rows to skip |
usecols |
The column indices to be read |
unpack |
Transpose returned array, so that arguments may be unpacked using x, y, z = loadtxt(...) . [unpack=True] |
ndim |
Minimum number of dimensions in returned array |
encoding |
Encoding used to decode the input file. |
max_rows |
Maximum number of rows to read after skiprows lines |
Return
N-dimensional array read from the txt
file.
Example Codes: NumPy Read txt
File Using numpy.loadtxt()
Function
import numpy as np
from io import StringIO
f = StringIO("3 6 8 \n12 9 1 \n 2 3 4")
a = np.loadtxt(f)
print("The loaded array is:")
print(a)
Output:
The loaded array is:
[[ 3. 6. 8.]
[12. 9. 1.]
[ 2. 3. 4.]]
It loads the txt
file into the NumPy array.
Here, StringIO
acts like a file object.
We can also provide the file path as an argument to the np.loadtxt
function using both absolute and relative paths.
Example Codes: Set dtype
Parameter in numpy.loadtxt()
Function While Reading the txt
File
By default, the data type of values of the array read from a txt
file is float
. We can manually set the data type of elements using the dtype
parameter.
import numpy as np
from io import StringIO
f = StringIO("3 6 8 \n12 9 1 \n 2 3 4")
a = np.loadtxt(f,dtype="int")
print("The loaded array is:")
print(a)
Output:
The loaded array is:
[[ 3 6 8]
[12 9 1]
[ 2 3 4]]
The above code loads all the elements into an array from txt
file as integers.
Example Codes: Set delimiter
Parameter in numpy.loadtxt()
Function While Reading txt
Files
By default, the delimiter
to separate the values is whitespace. We can manually set delimiter
using the delimiter
parameter.
import numpy as np
from io import StringIO
f = StringIO("3, 6, 8 \n12, 9, 1 \n 2, 3, 4")
a = np.loadtxt(f,dtype="int",delimiter=",")
print("The loaded array is:")
print(a)
Output:
The loaded array is:
[[ 3 6 8]
[12 9 1]
[ 2 3 4]]
As the txt
file’s values are separated by ,
, we have to use ,
as the delimiter to separate values while reading from the txt
file into the array.
Example Codes: Set usecols
Parameter in numpy.loadtxt()
Function While Reading the txt
File
import numpy as np
from io import StringIO
f = StringIO("3 6 8 \n12 9 1 \n 2 3 4")
a = np.loadtxt(f,dtype="int",usecols =(0, 1))
print("The loaded array is:")
print(a)
Output:
The loaded array is:
[[ 3 6]
[12 9]
[ 2 3]]
The usecols
specifies which columns to be read from the txt
file.
It reads only the first and second column from the txt
file into the array.
Example Codes: Set unpack
Parameter in numpy.loadtxt()
Function While Reading txt
Files
import numpy as np
from io import StringIO
f = StringIO("3 6 8 \n12 9 1 \n 2 3 4")
(x,y,z) = np.loadtxt(f,dtype="int",unpack=True)
print(x)
print(y)
print(z)
Output:
[ 3 12 2]
[6 9 3]
[8 1 4]
It transposes the array and unpacks the rows of the transposed array into specified variables.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn