How to Fix Python IndexError: list assignment index out of range
-
Python
IndexError: list assignment index out of range
-
Fix the
IndexError: list assignment index out of range
in Python -
Fix
IndexError: list assignment index out of range
Usingappend()
Function -
Fix
IndexError: list assignment index out of range
Usinginsert()
Function - Conclusion
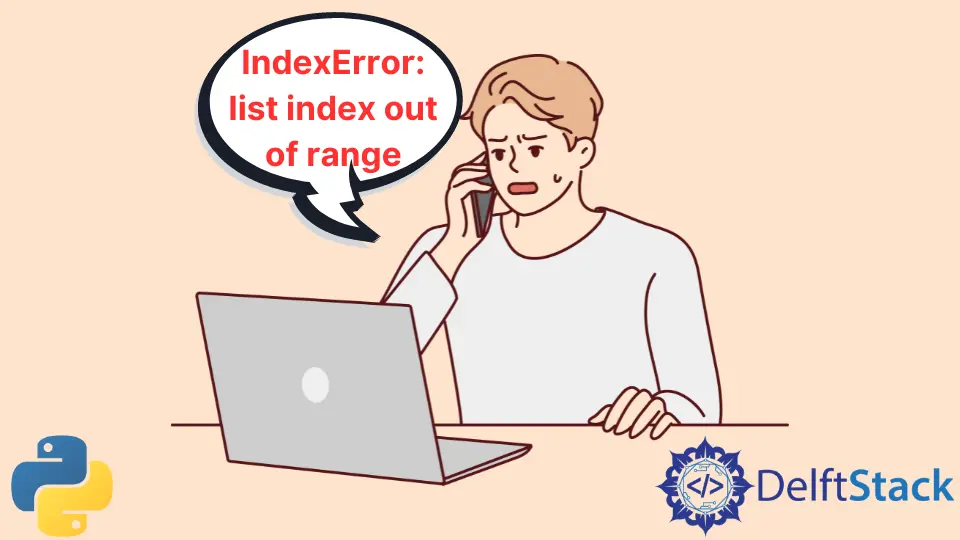
In Python, the IndexError: list assignment index out of range
is raised when you try to access an index of a list that doesn’t even exist. An index is the location of values inside an iterable such as a string, list, or array.
In this article, we’ll learn how to fix the Index Error list assignment index out-of-range error in Python.
Python IndexError: list assignment index out of range
Let’s see an example of the error to understand and solve it.
Code Example:
# error program --> IndexError: list assignment index out of range
i = [7, 9, 8, 3, 7, 0] # index range (0-5)
j = [1, 2, 3] # index range (0-3)
print(i, "\n", j)
print(f"\nLength of i = {len(i)}\nLength of j = {len(j)}")
print(f"\nValue at index {1} of list i and j are {i[1]} and {j[1]}")
print(
f"\nValue at index {3} of list i and j are {i[3]} and {j[3]}"
) # error because index 3 isn't available in list j
Output:
[7, 9, 8, 3, 7, 0]
[1, 2, 3]
Length of i = 6
Length of j = 3
Value at index 1 of list i and j are 9 and 2
IndexError: list assignment index out of range
The reason behind the IndexError: list assignment index out of range
in the above code is that we’re trying to access the value at the index 3
, which is not available in list j
.
Fix the IndexError: list assignment index out of range
in Python
To fix this error, we need to adjust the indexing of iterables in this case list. Let’s say we have two lists, and you want to replace list a
with list b
.
Code Example:
a = [1, 2, 3, 4, 5, 6]
b = []
k = 0
for l in a:
b[k] = l # indexError --> because the length of b is 0
k += 1
print(f"{a}\n{a}")
Output:
IndexError: list assignment index out of range
You cannot assign values to list b
because the length of it is 0
, and you are trying to add values at kth
index b[k] = I
, so it is raising the Index Error. You can fix it using the append()
and insert()
.
Fix IndexError: list assignment index out of range
Using append()
Function
The append()
function adds items (values, strings, objects, etc.) at the end of the list. It is helpful because you don’t have to manage the index headache.
Code Example:
a = [1, 2, 3, 4, 5, 6]
b = []
k = 0
for l in a:
# use append to add values at the end of the list
j.append(l)
k += 1
print(f"List a: {a}\nList b: {a}")
Output:
List a: [1, 2, 3, 4, 5, 6]
List b: [1, 2, 3, 4, 5, 6]
Fix IndexError: list assignment index out of range
Using insert()
Function
The insert()
function can directly insert values to the k'th
position in the list. It takes two arguments, insert(index, value)
.
Code Example:
a = [1, 2, 3, 5, 8, 13]
b = []
k = 0
for l in a:
# use insert to replace list a into b
j.insert(k, l)
k += 1
print(f"List a: {a}\nList b: {a}")
Output:
List a: [1, 2, 3, 4, 5, 6]
List b: [1, 2, 3, 4, 5, 6]
In addition to the above two solutions, if you want to treat Python lists like normal arrays in other languages, you can pre-defined your list size with None
values.
Code Example:
a = [1, 2, 3, 4, 5, 6]
b = [None] * len(i)
print(f"Length of a: {len(a)}")
print(f"Length of b: {len(b)}")
print(f"\n{a}\n{b}")
Output:
Length of a: 6
Length of b: 6
[1, 2, 3, 4, 5, 6]
[None, None, None, None, None, None]
Once you have defined your list with dummy values None
, you can use it accordingly.
Conclusion
There could be a few more manual techniques and logic to handle the IndexError: list assignment index out of range
in Python. This article overviews the two common list functions that help us handle the Index Error in Python while replacing two lists.
We have also discussed an alternative solution to pre-defined the list and treat it as an array similar to the arrays of other programming languages.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python