How to Calculate Dot Product in Python
-
Use the
*
Sign to Calculate the Dot Product of Two Scalars in Python -
Use the
numpy.dot()
Function to Calculate the Dot Product of Two Arrays or Vectors in Python -
Use the
sum()
Function to Calculate the Dot Product of Two Arrays or Vectors in Python -
Use the
map()
Function Along With themul()
Function to Calculate the Dot Product of Two Arrays or Vectors in Python -
Use the
more_itertools
Library to Calculate the Dot Product of Two Arrays or Vectors in Python
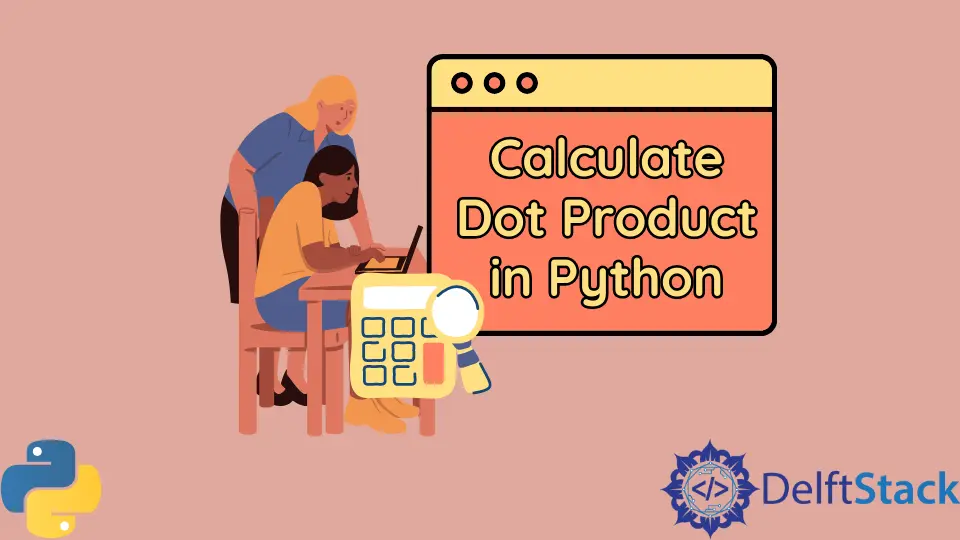
This tutorial introduces the different ways to calculate the dot product of two arrays or vectors in Python.
Before we move on to the different methods to implement this, we will first learn about the dot product in Python.
As you may know, a dot product, sometimes even referred to as a scalar product, is an algebraic operation carried out between any two specified arrays; they could be scalar or vector. The output is always a single number.
Python allows the calculation of the dot product of two arrays, provided the length-sequences of both the arrays are similar.
Use the *
Sign to Calculate the Dot Product of Two Scalars in Python
The scalars are also known as 0-dimensional arrays and are simply treated like standard numeric values. The dot product in between them would be the same as the product of both the scalars.
Although the below-mentioned methods would also work for scalars, this is a relatively simpler way of carrying out the process without having to do any extra work.
The following code uses the *
sign to calculate the dot product of two scalars in Python.
dotp = 2 * 5
print(dotp)
The above code provides the following output:
10
Use the numpy.dot()
Function to Calculate the Dot Product of Two Arrays or Vectors in Python
The term NumPy
is an acronym for Numerical Python. This library makes the use of arrays possible in Python. It also provides functions that help in manipulating these arrays.
The numpy.dot()
function is a function that is specially designed to carry out the purpose of finding the dot product between two arrays. The NumPy
module needs to be imported to the Python code to run smoothly without errors.
To explain this implementation in the Python code, we will take two lists and return the dot product.
The following code uses the numpy.dot()
function to calculate the dot product of two arrays or vectors in Python.
import numpy as np
x = [5, 10]
y = [4, -7]
dotp = np.dot(x, y)
print(dotp)
The above code provides the following output:
-50
The above code works for two-dimensional arrays as well. You can easily confirm the answers by quickly finding the dot product on your own in real life. This function gives accurate results if used correctly.
The same function can be used for scalars in the following way:
import numpy as np
dotp = np.dot(2, 5)
print(dotp)
The above code provides the following output:
10
Python 3.5 introduced the @
operator to calculate the dot product of n-dimensional arrays created using NumPy
. This method is widely utilized in the newer version of Python. We should note that it does not work on general lists.
Use the sum()
Function to Calculate the Dot Product of Two Arrays or Vectors in Python
A more ancient pythonic way would be to utilize the sum()
function and make some general tweaks to calculate the dot product between two arrays in Python.
The zip()
function is an in-built function offered in Python and is utilized here along with the sum()
function to combine the given arrays.
Here, we will also use list comprehension to make the code more compact.
The following code uses the sum()
function to calculate the dot product of two arrays or vectors in Python.
x = [5, 10]
y = [4, -7]
print(sum([i * j for (i, j) in zip(x, y)]))
The above code provides the following Output:
-50
Use the map()
Function Along With the mul()
Function to Calculate the Dot Product of Two Arrays or Vectors in Python
The map()
function is utilized for returning a map object after applying any given function to all the items of the selected iterable.
The mul()
function, as its name suggests, is an in-built function for carrying out the task of multiplication of any two numbers. The mul()
function can be found and used by importing it from the operator
library.
The following code uses the map()
function along with the mul()
function to calculate the dot product of two arrays or vectors in Python.
from operator import mul
x = [5, 10]
y = [4, -7]
print(sum(map(mul, x, y)))
The above code provides the following output:
-50
Use the more_itertools
Library to Calculate the Dot Product of Two Arrays or Vectors in Python
The more_iteratertools
is a third-party library with a huge presence on the Github
platform. It simply implements the general dotproduct
itertools
recipe that already exists.
The following code uses the more_itertools
library to calculate the dot product of two arrays or vectors in Python.
import more_itertools as mit
a = [5, 10]
b = [4, -7]
print(mit.dotproduct(a, b))
The above code provides the following output:
-50
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python Array
- How to Initiate 2-D Array in Python
- How to Count the Occurrences of an Item in a One-Dimensional Array in Python
- How to Downsample Python Array
- How to Sort 2D Array in Python
- How to Create a BitArray in Python
- How to Fix the Iteration Over a 0-D Array Error in Python NumPy