How to Calculate Inverse of Cosine in Python
-
Calculate Inverse of Cosine Using
math.acos()
Function in Python -
Calculate Inverse of Cosine Using
degrees()
andacos()
Function in Python - Calculate Inverse of Cosine Using Python NumPy Library
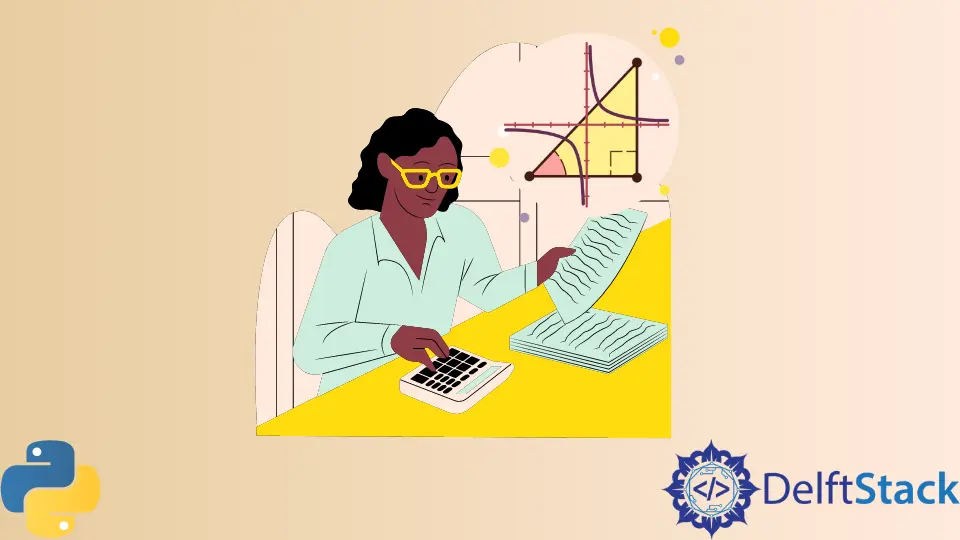
The inverse of cosine is the trigonometric function which is widely used to solve many problems in trignometry. The inverse of cosine is also called arc cosine. We can calculate the inverse of cosine in Python using the math.acos()
function and NumPy library.
Calculate Inverse of Cosine Using math.acos()
Function in Python
import math
print(math.acos(0.2))
print(math.acos(0.8))
print(math.acos(0))
print(math.acos(1))
Output:
1.369438406004566
0.6435011087932843
1.5707963267948966
0.0
First of all, we need to import the math library and then apply the math.acos()
function to our input value to find the inverse of cosine.Inverse of cosine using the acos()
function gives the result in radians.
Calculate Inverse of Cosine Using degrees()
and acos()
Function in Python
Inverse of cosine using the acos()
function gives the result in radians. If we need to find the inverse of cosine output in degrees instead of radian then we can use the degrees()
function with the acos()
function.
import math
result = math.acos(0.2) # radian
print(result)
print(math.degrees(result)) # degree
Output:
1.369438406004566
78.46304096718453
Here, we first get the inverse of cosine in radian and store it in the result
variable. Then we apply the degrees()
function to the result variable to get the final value in degrees.
We can see that we get the output as a floating-point number. We can fix it to any number of decimal places.
import math
result = math.acos(0.2)
print("%.2f" % result)
Output:
1.37
In this way, we can use the formatting of float values in Python to the inverse of cosine.
Calculate Inverse of Cosine Using Python NumPy Library
We can find the inverse of cosine using the NumPy library. The advantage of using the NumPy library is that we can find the inverse of cosine of range of elements all at once.
import numpy as np
print(np.arccos(0.2))
print(np.arccos([0.2, 0.8, 0, 0.5]))
Output:
1.369438406004566
[1.36943841 0.64350111 1.57079633 1.04719755]
Here, the NumPy library provides us with an arccos()
function to find the inverse of cosine. Either we can give a single value to the arccos()
function or a list of values.