Python math.acos() Method
-
Syntax of Python
math.acos()
Method -
Example Codes: Use Python
math.acos()
to Find Arccosine of a Value -
Example Codes: Use Python
math.acos()
to Find Arccosine and Cosine
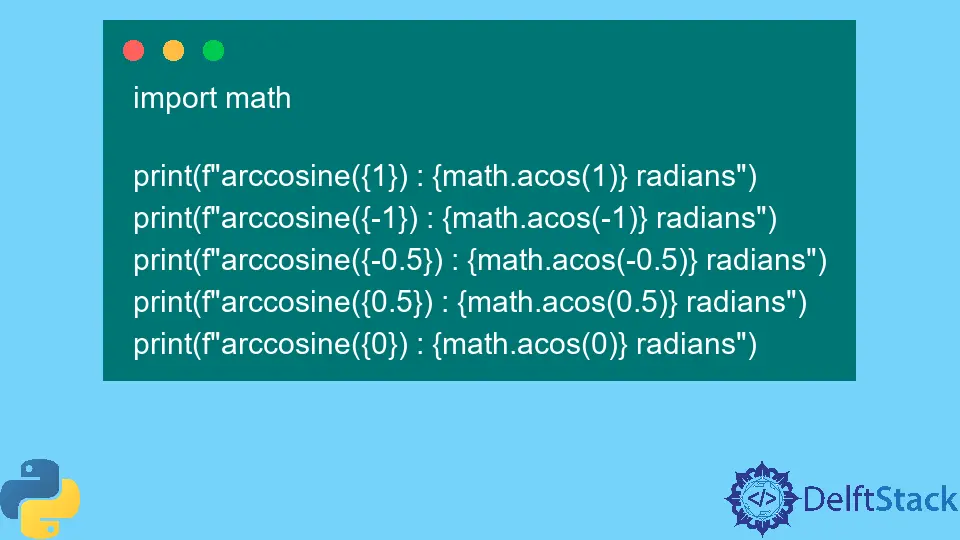
Python has a built-in math module specifically for performing mathematics operations. Additionally, it also supports trigonometric and inverse trigonometric functions.
The inverse of cosine is known as arccosine or cos⁻¹θ
. Theoretically, the cosine of an angle θ
is the ratio of its adjacent side and the hypotenuse and the domain and range of arccosine are [-1, 1]
and [0, π]
, respectively.
cos(θ) = (adjacent side) / (hypotenuse)
arccosine(θ) = cos⁻¹((adjacent side) / (hypotenuse))
This article will discuss the acos()
method from the math module that helps calculate arccosine for angles.
Syntax of Python math.acos()
Method
math.acos(x)
Parameters
Type | Description | |
---|---|---|
x |
Integer | A value between -1 and 1, both inclusive. |
Returns
The acos()
method returns the inverse of cosine or arccosine of x
in radians. The result is between the range 0
and π
, both inclusive.
Example Codes: Use Python math.acos()
to Find Arccosine of a Value
import math
print(f"arccosine({1}) : {math.acos(1)} radians")
print(f"arccosine({-1}) : {math.acos(-1)} radians")
print(f"arccosine({-0.5}) : {math.acos(-0.5)} radians")
print(f"arccosine({0.5}) : {math.acos(0.5)} radians")
print(f"arccosine({0}) : {math.acos(0)} radians")
Output:
arccosine(1) : 0.0 radians
arccosine(-1) : 3.141592653589793 radians
arccosine(-0.5) : 2.0943951023931957 radians
arccosine(0.5) : 1.0471975511965979 radians
arccosine(0) : 1.5707963267948966 radians
The code above computes the inverse of cosine or arccosine for 1
, -1
, -0.5
, 0.5
, and 0
. All the results are in the range [0, π]
.
Example Codes: Use Python math.acos()
to Find Arccosine and Cosine
import math
x = -1
acos = math.acos(x)
print(f"arccosine({x}):", acos)
print(f"cos({acos}):", math.cos(acos))
x = 0
acos = math.acos(x)
print(f"arccosine({x}):", acos)
print(f"cos({acos}):", math.cos(acos))
x = 1
acos = math.acos(x)
print(f"arccosine({x}):", acos)
print(f"cos({acos}):", math.cos(acos))
Output:
arccosine(-1): 3.141592653589793
cos(3.141592653589793): -1.0
arccosine(0): 1.5707963267948966
cos(1.5707963267948966): 6.123233995736766e-17
arccosine(1): 0.0
cos(0.0): 1.0
The code above calculates the arccosine of some value x
and uses its result to re-compute x
using cosine. It performs the above operation for three values, -1
, 0
, and 1
.
Note that for x = 0
, the cosine output is 6.123233995736766e-17
, which is a minimal value and close to zero. Generally, such values are approximated to the nearest integer for simplicity’s sake, which is, in this case, 0
.