Python Math.fabs() Method
-
Syntax of Python
math.fabs()
Method -
Example Code: Use of the
math.fabs()
Method -
Example Code: Difference Between the
math.fabs()
andabs()
Methods -
Example Code: Pass a Negative Number Input to the
math.fabs()
Method -
Example Code: Solve the
name 'math' is not defined
Error When Using themath.fabs()
Method
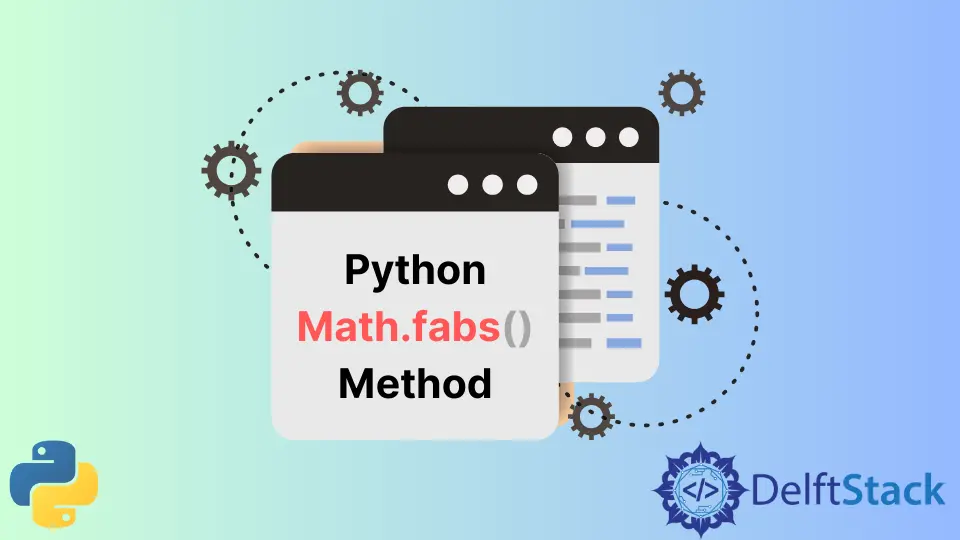
We use the math
module in Python for various mathematical functions. One of the methods is math.fabs()
method, which is similar to math.abs()
method.
This method, math.fabs()
, returns the absolute value as a float number. It removes the negative sign from the number, which means the method math.fabs()
returns the nonnegative number.
This method is available in Python 2.6 and above. In the previous versions, we used the built-in abs()
method to return the absolute value.
We use the float()
method to get the answer in a float value. The math.fabs()
method resolves the issue of using both; we use only one method.
Syntax of Python math.fabs()
Method
math.fabs(number)
Parameter
number |
It is a required parameter and a numeric value. |
Return
This method returns the absolute value of a specified numeric value as a float number. TypeError
is produced when the input is not a number.
Example Code: Use of the math.fabs()
Method
To use the fabs()
method, we need to import the static object with it, known as math
. In the below example, we pass the different types of data to the math.fabs()
method and output its result on the console.
fabs()
represents the float absolute value method, which takes the numeric value as the required parameter and returns the absolute value, which is a nonnegative number in float. We test the method math.fabs()
with different types of input values like positive/negative integer and float numbers.
This method is part of the theoretical and representation functions in Python.
# import math static object for fabs() method
import math
# returns floating nonnegative value for each type of specified data
def float_absolute(number):
return math.fabs(number)
# positive integer
print("The floating absolute value of 208 is", float_absolute(208))
# negative decimal number
print("The floating absolute value of -75.23 is", float_absolute(-75.23))
# postive decimal number
print("The floating absolute value of 90.66 is", float_absolute(90.66))
print("The floating absolute value of math.pi is", float_absolute(math.pi))
Output:
# output for different types of inputs
The floating absolute value of 208 is 208.0
The floating absolute value of -75.23 is 75.23
The floating absolute value of 90.66 is 90.66
The floating absolute value of math.pi is 3.141592653589793
Example Code: Difference Between the math.fabs()
and abs()
Methods
The abs()
method is a built-in utility function, while the fabs()
method is a math
module method introduced in Python 2.6. In the below example, when we pass the integer or float value to the fabs()
method, it always returns the result in float value.
While in the abs()
method, when we pass the integer value, it returns the integer value; similarly, when we provide the float value, it returns the float value.
import math
# test for negative integer values
print("The result of fabs method for integer value is ", math.fabs(-97))
print("The result of abs method for integer value is ", abs(-97))
# test for positive float values
print("The result of fabs method for float value is ", math.fabs(101.8))
print("The result of abs method for float value is ", abs(101.8))
Output:
# Difference between abs and fabs method with different inputs
The result of fabs method for integer value is 97.0
The result of abs method for integer value is 97
The result of fabs method for float value is 101.8
The result of abs method for float value is 101.8
The similarity is that both methods only take input in the form of integer and float values. The second example shows that when we pass any other type of data except the integer or float, the method returns the TypeError
.
import math
# returns TypeError for string data type
def check_type(value):
return math.fabs(value)
print("For a string value ", math.fabs("Hello"))
Output:
TypeError: must be real number, not str
Example Code: Pass a Negative Number Input to the math.fabs()
Method
In the below example, we take the two inputs from the user. The first input is a negative decimal number, and the other is a positive integer.
Both inputs are passed to the math.fabs()
method and later sum both numbers.
import math
# two inputs for positive and negative numbers
input1 = float(input("Enter a negative float number:"))
input2 = int(input("Enter a positive integer: "))
print("The absolute value of the first input is", math.fabs(input1))
print("The absolute value of the second input is", math.fabs(input2))
total = input1 + float(input2)
print("The sum of both inputs is", total)
print("The absolute value of the sum is", math.fabs(total))
Output:
Enter a negative float number:-8.96
Enter a positive integer: 34
The absolute value of the first input is 8.96
The absolute value of the second input is 34.0
The sum of both inputs is 25.04
The absolute value of the sum is 25.04
Example Code: Solve the name 'math' is not defined
Error When Using the math.fabs()
Method
The example below uses from math import *
in the Python code. The interpreter returns an error message saying the math
module is not defined.
# incorrect import type
from math import *
print("The absolute value of 1235 is", math.fabs(1235))
Output:
# fabs() method returns error on incorrect import type
NameError: name 'math' is not defined
To solve this error, we need to import the math
module directly by the use of import math
.
# correct import for the use of math module
import math
print("The absolute value of 1235 is", math.fabs(1235))
Output:
The absolute value of 1235 is 1235.0