Python Math.erf() Method
-
Syntax of Python
math.erf()
Method -
Example Code: Use of the
math.erf()
Method in Python -
Example Code: Use the
math.erf()
Method for the Same Number With Different Signs -
Example Code: Use the
math.erf()
Method to Return the Gaussian Probability Density Function -
Example Code: Use the
math.erf()
Method to Solve the Gaussian Integral -
Example Code: Use the
math.erf()
Method to Find the Integral - Conclusion
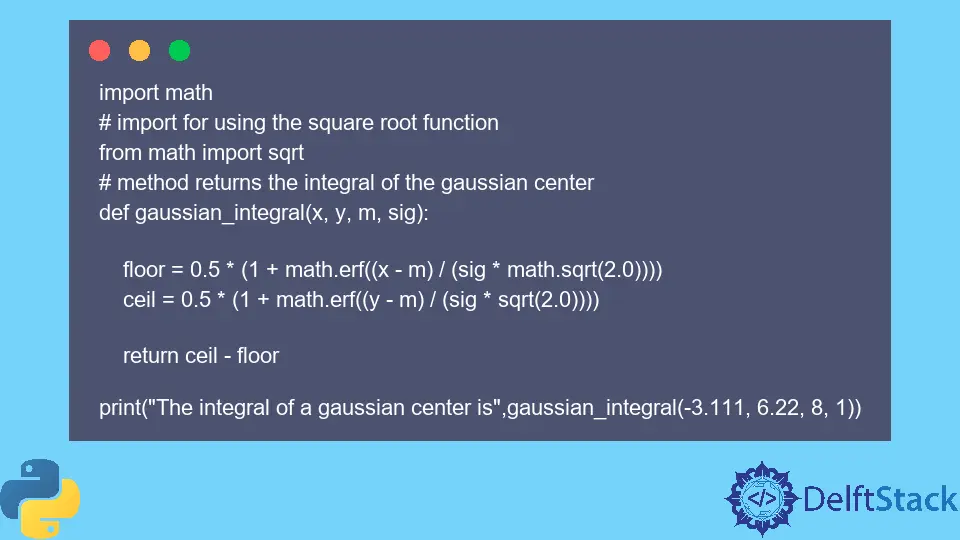
We use Python’s math
module to perform mathematical calculations and standards work. This module provides us with many different methods, such as the math.erf()
method, which takes a number and returns the error function for the provided argument.
The math.erf()
method was newly introduced in Python version 3.2. These error methods are usually related to computational problems and machine learning techniques.
The math.erf()
method accepts the value range from -Infinity
to +Infinity
. We use this method to solve various mathematical problems such as Gaussian and integrals of the numbers.
Syntax of Python math.erf()
Method
math.erf(number)
Parameter
Parameter | Description |
---|---|
number |
This is the number to find the error function and takes from -Infinity to +Infinity . |
Return
This method returns the floating value and shows the error value for the provided argument.
Example Code: Use of the math.erf()
Method in Python
In Python version 3.2 and later, we use the method math.erf()
to check the error function of the provided number. This method is used to compute the distribution function for the standard normal distribution, and it is actually integral to the normal distribution.
This method accepts a number ranging from -Infinity
to +Infinity
and returns a value ranging from -1
to +1
. The below example shows the output variation of the different arguments provided.
import math
def find_error_function_value(number):
return math.erf(number)
print("Error function of 65 =", find_error_function_value(65))
print("Error function of -3 =", find_error_function_value(-3))
print("Error function of -0.016 =", find_error_function_value(-0.016))
print("Error function of 19 =", find_error_function_value(19))
print("Error function of 1.98 =", find_error_function_value(1.98))
print("Error function of 908 =", find_error_function_value(908))
Output:
Error function of 65 = 1.0
Error function of -3 = -0.9999779095030014
Error function of -0.016 = -0.018052526178150646
Error function of 19 = 1.0
Error function of 1.98 = 0.9948920003868136
Error function of 908 = 1.0
In our code, we utilize Python’s math
module to calculate error functions using the math.erf()
method. This method takes a numerical argument and returns the corresponding error function value.
First, we showcase the versatility of this function by evaluating error functions for various input values. For instance, we calculate the error function of 65
, -3
, -0.016
, 19
, 1.98
, and 908
.
The output reveals the computed error function values for each respective input, demonstrating the broad applicability of the math.erf()
method in handling a range of numerical computations.
Example Code: Use the math.erf()
Method for the Same Number With Different Signs
In the below example, we will provide the same number to the math.erf()
method with a positive and negative sign. The output shows that if the provided number has a +
sign, the result is positive and vice versa.
These two examples show that the result of the method math.erf()
always returns a value ranging between -1
and +1
.
import math
def test_postive_negative_number(number):
return math.erf(number)
print(
"Error function of a number with a positive sign =",
test_postive_negative_number(9.28),
)
print(
"Error function of a number with a negative sign =",
test_postive_negative_number(-9.28),
)
Output:
Error function of a number with a positive sign = 1.0
Error function of a number with a negative sign = -1.0
In this code, with the test_positive_negative_number
function, we examine the behavior of the error function when provided with a number featuring both positive and negative signs. By applying the method to a number with a positive sign (9.28
) and another with a negative sign (-9.28
), we observe that the output mirrors the sign of the input.
Specifically, the error function of a positive number yields a positive result, while the error function of a negative number produces a negative result. This underscores the symmetry of the error function with respect to sign, a characteristic fundamental to its mathematical properties.
Example Code: Use the math.erf()
Method to Return the Gaussian Probability Density Function
In the below example, the math.erf()
method helps to return the area under the Gaussian probability density function.
The method integrates from -Infinity
to the provided number. The argument number
can be a simple real or complex number.
The ndtr()
method returns the scalar value of the provided number. The method compares the provided value’s absolute and square root and returns the result.
import math
def ndtr(number):
square_root = math.sqrt(2) / 2
initial_result = float(number) * square_root
absolute = abs(initial_result)
if absolute < square_root:
result = 0.5 + 0.5 * math.erf(initial_result)
else:
result = 0.5 * math.erfc(absolute)
if initial_result > 0:
result = 1 - result
return result
print(
"The result of the area under the Gaussian probability density function is",
ndtr(5.33),
)
Output:
The result of the area under the Gaussian probability density function is 0.9999999508936167
In this code, we utilize the math
module in Python to define a function named ndtr
. This function calculates the area under the Gaussian probability density function for a given numerical input, represented by the variable number
.
We begin by computing a scaled value, initial_result
, which is the product of the input and a pre-defined square root constant. The function then evaluates whether the absolute value of this scaled result is less than another square root constant.
Depending on this condition, we determine the final result using the error function (math.erf()
) and its complementary function (math.erfc()
). The provided example applies the ndtr
function to the number 5.33
and prints the result, showcasing its application in computing the area under the Gaussian probability density function.
The output demonstrates the calculated result for this specific input, illustrating the practical use of the ndtr
function in probabilistic computations.
Example Code: Use the math.erf()
Method to Solve the Gaussian Integral
In Python version 3.2 and above, the math.erf()
method can also be used to solve the Gaussian integral. For this purpose, we code a method known as gaussian_integral()
with four parameters: x
, y
, mean
, and sigma
.
The method contains two statements for the integral of negative/positive infinity to x
and for the integral of negative/positive infinity to y
. The method returns the substitution of the ceiling-to-floor statement.
import math
from math import sqrt
def gaussian_integral(x, y, m, sig):
floor = 0.5 * (1 + math.erf((x - m) / (sig * math.sqrt(2.0))))
ceil = 0.5 * (1 + math.erf((y - m) / (sig * sqrt(2.0))))
return ceil - floor
print("The integral of a gaussian center is", gaussian_integral(-3.111, 6.22, 8, 1))
Output:
The integral of a gaussian center is 0.0375379803485168
In this code, we utilize Python’s math
module to define a function named gaussian_integral
. This function calculates the integral of a Gaussian distribution centered around a mean (m
) with a given standard deviation (sig
).
The integral is computed over the range from x
to y
. We use the error function (math.erf()
) to determine the cumulative distribution function (CDF) values at the upper and lower bounds of the range.
The output is then obtained by subtracting the lower CDF value from the upper CDF value. The example provided applies the gaussian_integral
function to the values -3.111
(as x
), 6.22
(as y
), 8
(as mean), and 1
(as standard deviation).
The printed output represents the result of this Gaussian integral calculation, providing a concise illustration of the function’s application in computing the area under a Gaussian distribution curve.
Example Code: Use the math.erf()
Method to Find the Integral
The method math.erf()
is also used to find the integral of two given (float number) parameters and returns the float value of the calculated integral. The below example takes two floating values as a parameter to the default_integral()
method and returns the float value for the integrals.
import math
def default_integral(a, b):
return 0.5 * (math.erf(b) - math.erf(a))
print("The value of the integral is", default_integral(9.188, 3.56))
Output:
The value of the integral is -2.394234760449976e-07
In this code, we employ the math
module in Python to define a function named default_integral
. This function calculates the integral of the error function (math.erf()
) between two specified points, denoted by the parameters a
and b
.
The formula utilized involves subtracting the error function value at a
from the error function value at b
and scaling the result by 0.5
. The printed output statement then displays the computed value of this integral for the input range [9.188, 3.56]
.
The output provides a concise representation of the result, demonstrating the application of the default_integral
function in determining the integral of the error function over a specified interval.
Conclusion
The math.erf()
method is a powerful tool for calculating the error function, which finds applications in various mathematical and computational domains. Introduced in Python version 3.2, this method efficiently handles a wide range of numerical inputs, enabling the computation of Gaussian distributions, integrals, and probability density functions.
The examples presented demonstrate its versatility in solving problems related to machine learning, probability, and mathematical analysis. Incorporating this method into Python code provides a reliable means to address complex computational challenges involving error functions and contributes to the broader spectrum of scientific computing capabilities in the language.