Python Math.erfc() Method
-
Syntax of Python
math.erfc()
Method -
Example Code: Use of the
math.erfc()
Method -
Example Code: Cause of the
TypeError
When Using themath.erfc()
Method -
Example Code: Use the
math.erfc()
Method to Determine the Quantiles of the Gaussian Distribution -
Example Code: Use the
math.erfc()
Method to Find the Area Under the Gaussian Probability Function
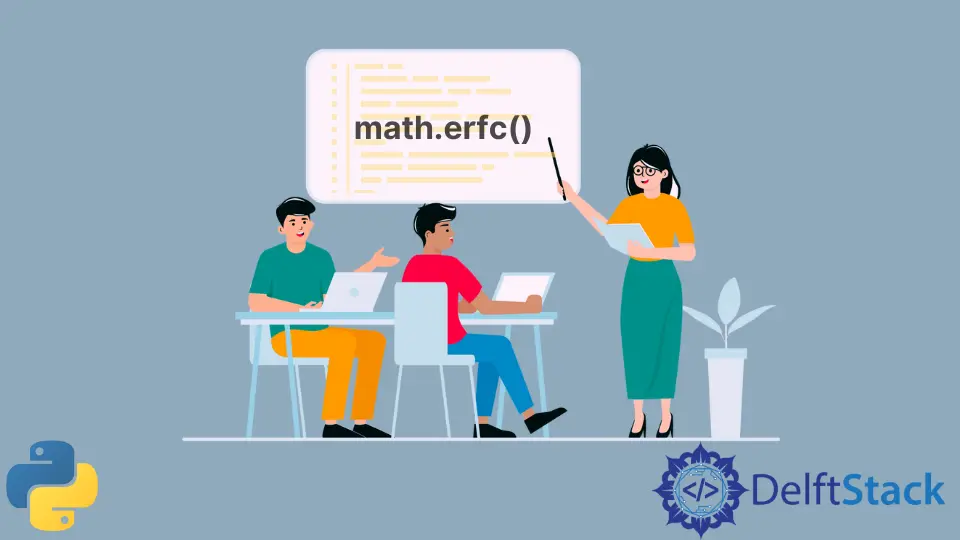
In Python, the math
module provides many mathematical utility functions, and one of these methods is math.erfc()
. This method aims to find the complementary error function of the specified parameter.
This complementary error function is also known as the Gaussian error function. This method throws an error if the parameter is not a number.
This method is mainly done to find the cause of the loss of significance. This method takes the values from -Infinity to +Infinity and returns the value range between 0 and 2.
This method is an error function, usually in statistics, probability, and partial differential equations.
Syntax of Python math.erfc()
Method
math.erfc(number)
Parameter
number |
This method takes a single parameter as a floating value. The method throws an error if any other data is given. |
Return
This method returns the error function of a number as a float value.
Example Code: Use of the math.erfc()
Method
To use the method math.erfc()
, we have to use Python version 3.2 or above. The method throws an error if the parameter data type is not a numeric or a float number.
The below example shows four parameters passed to the math.erfc()
method, which are positive/negative integer and decimal numbers. The resultant values range from 0 to 2.
# math import for the use of built-in mathematical functions
import math
# returns the complementary error function
def complementary_error_function(number):
return math.erfc(number)
print("The complementary error function for 20 is", complementary_error_function(20))
print(
"The complementary error function for 9.88 is", complementary_error_function(9.88)
)
print("The complementary error function for -6 is", complementary_error_function(-6))
print(
"The complementary error function for -2.44 is", complementary_error_function(-2.44)
)
Output:
# result shows that the value range between 0 to 2.
# result when +integer number is passed as a parameter
The complementary error function for 20 is 5.395865611607901e-176
# result when +decimal number is passed as a parameter
The complementary error function for 9.88 is 2.296551005681906e-44
# result when -integer number is passed as a parameter
The complementary error function for -6 is 2.0
# result when a -decimal number is passed as a parameter
The complementary error function for -2.44 is 1.9994408261164485
Example Code: Cause of the TypeError
When Using the math.erfc()
Method
In Python version 3.2 and above, if the math.erf()
method’s parameter is not the floating value, the method returns a TypeError
. The TypeError
usually occurs when the methods need some other type of data, unlike the provided one.
The math.erfc()
method accepts only the floating value as a parameter.
import math
# methods returns the TypeError exception error
def complementary_error_function(number):
return math.erfc(number)
# the passing parameter is a string, not a number
print("The complementary error function for 20 is", complementary_error_function("a"))
Output:
# Not a valid argument. We need to change the type to float value
TypeError: must be a real number, not str
Example Code: Use the math.erfc()
Method to Determine the Quantiles of the Gaussian Distribution
The math.erfc()
method can be used for different mathematical work as it determines the error function. The below implementation is a use case of the math.erfc()
method, where we have a function named gaussian_qualites()
.
The function takes four variables: acquisition function parameter, current minimum value, vector of mean and standard deviation.
import math
# numpy module for using NumPy array
import numpy as np
# returns the acquisition function values
def gaussian_qualites(acqui, mi, means, sd):
if isinstance(sd, np.ndarray):
sd[sd < 1e-10] = 1e-10
elif sd < 1e-10:
sd = 1e-10
u = (mi - means - acqui) / sd
phi = np.exp(-0.5 * u ** 2) / np.sqrt(2 * np.pi)
# here, we have the vector form of erfc
Phi = 0.5 * math.erfc(-u / np.sqrt(2))
return (phi, Phi, u)
print(
"The quantiles of the Gaussian distribution are ",
gaussian_qualites(10, 2.4, 6.62, 99),
)
Output:
# return output as a vector
The quantiles of the Gaussian distribution are (0.39484806419518365, 0.44289381217966217, -0.14363636363636365)
Example Code: Use the math.erfc()
Method to Find the Area Under the Gaussian Probability Function
We often use Python’s math
module to find complex mathematical problem solutions. The latest Python version, like 3.2 or above, tends to solve any problems with their automated solutions.
In the below example, we use the math.erfc()
method to find the area under the Gaussian probability density function.
The method gaussian_probability()
takes arguments from -Infinity to a specified value n
. We will pass a positive and a negative float value to the method to validate it.
The input -4.0
in the below code did not return a negative value because the value first passes through the abs()
method, which makes it a positive number before it goes to the math.erfc()
method.
import math
# method returns the area under the probability density function
def gaussian_probability(number):
square_root = math.sqrt(2) / 2
a = float(number) * square_root
# abs() method always returns the positive number
absolute_value = abs(a)
if absolute_value < square_root:
b = 0.5 + 0.5 * math.erf(a)
else:
b = 0.5 * math.erfc(absolute_value)
if a > 0:
b = 1 - b
return b
# positive and negative float number as an argument
print(
"The area under the Gaussian probability for a positive number is",
gaussian_probability(2.8),
)
print(
"The area under the Gaussian probability for a negative number is",
gaussian_probability(-4.0),
)
Output:
The area under the Gaussian probability for a positive number is 0.997444869669572
The area under the Gaussian probability for a negative number is 3.167124183311989e-05