Python Math.factorial() Method
-
Syntax of Python
math.factorial()
Method -
Example Code: Use of the
math.factorial()
Method -
Example Code: Cause of the
ValueError
Exception When Using themath.factorial()
Method -
Example Code: Cause of the
TypeError
Exception When Using themath.factorial()
Method -
Example Code: Use the
math.factorial()
Method to Find the Permutation -
Example Code: Easiness of the
math.factorial()
Method -
Example Code: Runtime Complexities of the
math.factorial()
Method
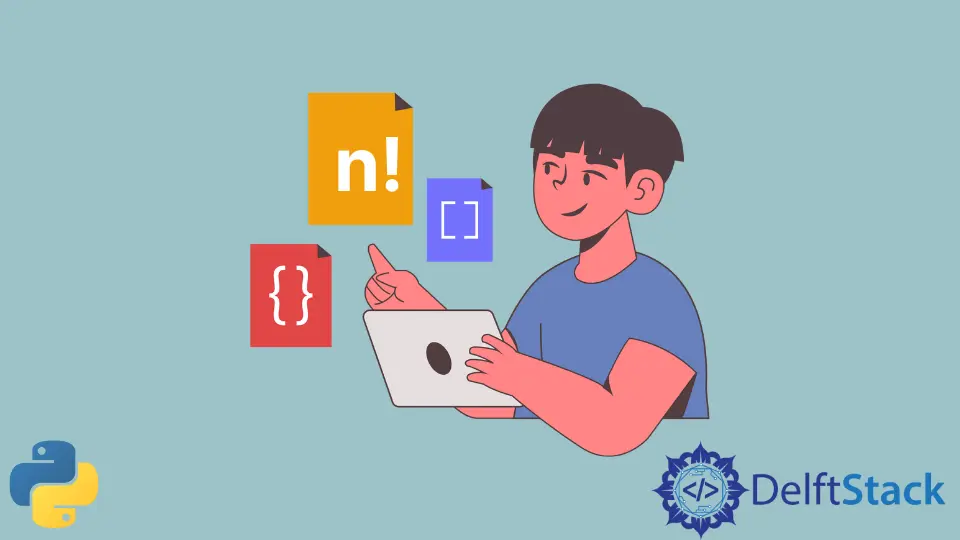
In Python, we use the math.factorial()
method to return the factorial of a number. The method math.factorial()
is introduced in Python version 2.6 and is part of theoretical and representation functions in Python.
This method takes a positive integer value and returns the factorial of the specified positive integer. This method returns ValueError
when we pass a non-positive or a decimal number to the factorial()
method.
TypeError
occurs when the parameter is not even a number.
A number’s factorial is the sum of all the multiplication starting from the specified positive number to 1, which includes all the whole numbers within the range. For example, the factorial of 7 is 7x6x5x4x3x2x1 = 5040.
Syntax of Python math.factorial()
Method
math.factorial(number)
Parameter
number |
This is a required parameter, a positive integer value. This parameter does not allow negative or decimal number values. |
Return
This method returns the factorial as a positive integer value.
Example Code: Use of the math.factorial()
Method
To use the factorial()
method in Python, we must import the math
module. One of the methods provided by the math
module is the math.factorial()
method, which only takes the positive integer value and returns the positive integer value.
This method does not take any other type of data value.
# import math module to use the math.factorial() method
import math
# returns the factorial of a number
def evaluate_factorial(number):
return math.factorial(number)
num = 13
print("The factorial of 13 is ", end="")
print(evaluate_factorial(num))
Output:
The factorial of 13 is 6227020800
Example Code: Cause of the ValueError
Exception When Using the math.factorial()
Method
We will pass the positive integer value as a parameter in the math.factorial()
method. When we pass a non-positive value to the method, the factorial()
method returns the ValueError
exception.
We can handle such exceptions with the try
and except
statements.
import math
def evaluate_factorial(number):
return math.factorial(number)
# not a positive integer number
num = 13.1
# exception caught when the value of num is decimal
try:
print("The factorial of 13.1 is ", evaluate_factorial(num))
except ValueError as ve:
print(f"You entered a {num} number, which is not a positive integer number.")
Output:
You entered a 13.1 number, which is not a positive integer number.
Example Code: Cause of the TypeError
Exception When Using the math.factorial()
Method
Similar to the above example, the math.factorial()
method returns the TypeError
exception when the data type is not a number as a parameter. We can also handle the TypeError
exception inside the source code.
import math
def evaluate_factorial(number):
return math.factorial(number)
# invalid string type for factorial() method
word = "Hello"
# exception caught when the parameter type is a string
try:
print("The factorial of 33 is ", evaluate_factorial(word))
except TypeError as ve:
print(f"You entered a {word} word, which is a string.")
Output:
You entered a Hello word, which is a string.
Example Code: Use the math.factorial()
Method to Find the Permutation
We use the math.factorial()
method to solve different formulas in mathematics. The formula is n!/(n-r)!
, where n
is the total items in the set and r
is the items from the permutation.
The formula is used to find the permutation of a number. The formula cannot take a value of r
greater than or equal to the value of n
.
import math
# returns result for formula n!/(n-r)!
def permutation(n, r):
return math.factorial(n) / math.factorial(n - r)
# inputs 'n' and 'r' are needed for the formula
n = int(input("First input: Enter value of n: "))
while True:
r = int(input("Second input: Enter value of r: "))
if r >= n:
print("The value of r cannot be greater or equal to n. Input again")
continue
break
print("The result of n! / (n-r)! is", permutation(n, r))
Output:
First input: Enter value of n: 5
# first input cannot be greater than the second input
Second input: Enter value of r: 7
The value of r cannot be greater or equal to n. Input again
Second input: Enter value of r: 3
The result of n! / (n-r)! is 60.0
Example Code: Easiness of the math.factorial()
Method
This method provides the easiness of a single statement by taking a required parameter and returning the factorial. If we do not use this method inside the math
module, we need to write a lot of statements.
# math.factorial does this in a single statement
def factorial(num):
if num == 0 or num == 1:
return 1
else:
return num * factorial(num - 1)
print("The factorial of 4 is", factorial(4))
Output:
The factorial of 4 is 24
Example Code: Runtime Complexities of the math.factorial()
Method
In a factorial, the number gets multiplied by the specified number to 1, including all whole numbers between the range. For a larger number, factorial gets very large and grows exponentially.
So, there is always a need to calculate the Big-O notation when there is a larger multiplication. For this reason, the program’s structure is an important factor that impacts the run time difference.
We write two basic factorial()
programs with the same parameter but different program logic to differentiate in runtime complexities.
Program 1:
import math
import time
begin = time.time()
def factorial(a):
if a == 0 or a == 1:
return 1
else:
return a * factorial(a - 1)
print("Factorial:", factorial(30))
stop = time.time()
print("The time elapsed for the Recursive method is " + str(stop - begin))
Output:
Factorial: 265252859812191058636308480000000
The time elapsed for the Recursive method is 0.000997781753540039
Program 2:
import math
import time
begin = time.time()
# logic is different in the second program for finding factorials.
def fact(a):
facto = 1
for number in range(2, a + 1):
facto *= number
return facto
print("Factorial:", fact(30))
stop = time.time()
print("The time elapsed for the iterative method is " + str(stop - begin))
Output:
# same factorial but the difference in the run time of both programs.
Factorial: 265252859812191058636308480000000
The time elapsed for the iterative method is 0.00099945068359375