Python Math.expm1() Method
-
Syntax of Python
math.expm1()
Method -
Example Code: Use of the
math.expm1()
Method -
Example Code: Difference Between
math.expm1()
andmath.exp()-1
-
Example Code: Same Method With
numpy.expm1()
-
Example Code: Compute the
log(1-exp(x))
Expression -
Example Code: Compute the
log(1-expm1(x))
Expression
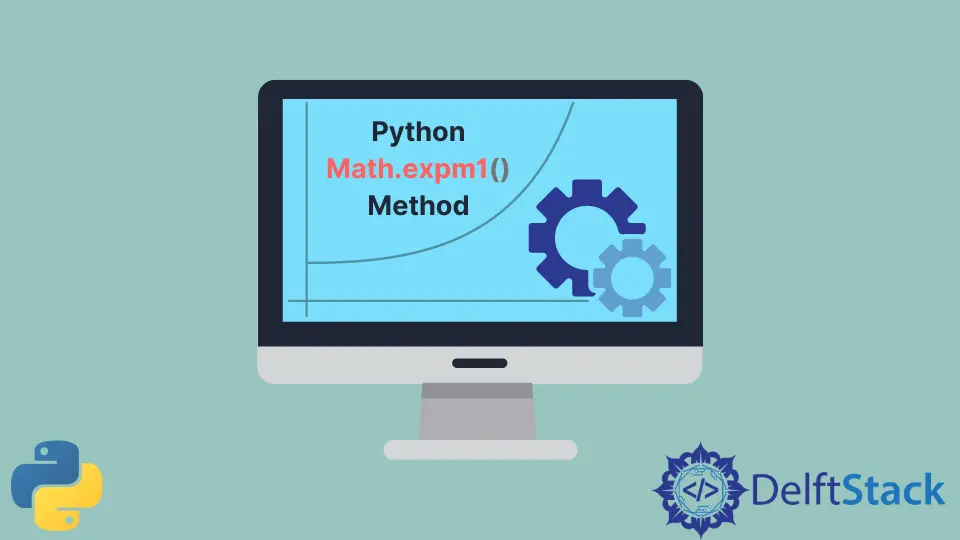
In Python, we utilize mathematical functions using the math
module, which contains various logarithmic functions. One of the methods is math.expm1()
, which is quite similar to math.exp()
.
The math.exp(x)
method uses ex, while math.expm1()
uses ex-1. This method is available in Python 3.2 and later versions.
The math.expm1()
operates with full precision. The argument x
can be a smaller number than exp(x)-1
, providing a significant loss of precision.
The method expm1()
handles this situation and returns results to full precision. The value of the e
is approximately 2.7182, which is the base of the natural logarithm.
How does the calculator solve ex? Suppose the value of x
is close to 0, then the output would be approximately equal to 1+x
because the expression ex-1 / x
is equal to zero.
Syntax of Python math.expm1()
Method
math.expm1(number)
Parameter
number |
Required parameter with a floating value. We compute the exponential of this number minus 1. |
Return
This method returns the exponential value of the provided parameter with a -1
result.
Example Code: Use of the math.expm1()
Method
To use the method expm1()
, we must import the math
module. We pass the four different data types as a parameter to test the math.expm1()
method.
The method shows that the negative numbers (either integer or float) result in a negative number. The method needs to run in Python 3.2 or later versions.
# use math import for the use of the expm1() method
import math
# method return results differently for the data
def check_input(number):
return math.expm1(number)
# test method for four types of data
positive_integer = 6
negative_integer = -8
positive_decimal_number = 14.5
negative_decimal_number = -9.3
print(
"The expm1 method returns the value for the positive integer "
+ str(check_input(positive_integer))
)
print(
"The expm1 method returns the value for the negative integer "
+ str(check_input(negative_integer))
)
print(
"The expm1 method returns the value for the positive decimal number "
+ str(check_input(positive_decimal_number))
)
print(
"The expm1 method returns the value for the negative decimal number "
+ str(check_input(negative_decimal_number))
)
Output:
# tests four types of data here
The expm1 method returns the value for the positive integer 402.4287934927351
The expm1 method returns the value for the negative integer -0.9996645373720975
The expm1 method returns the value for the positive decimal number 1982758.2635375687
The expm1 method returns the value for the negative decimal number -0.9999085757685219
Example Code: Difference Between math.expm1()
and math.exp()-1
As we discuss the method math.expm1()
results in exp()-1
. So, the important thing to demonstrate here is that if the math.expm1()
method does exp()-1
, then what is the method’s basic functionality?
One of the reasons is that the method math.expm1()
is often used in many mathematical and science applications. The second reason is when the value of x
(given parameter) is small, then the method expm1()
provides better results as compared to exp()-1
.
import math
# test the value to compare with both methods
test_value = 2e-14
print("The result with exp()-1 method is " + str(math.exp(test_value) - 1))
print("The result with expm1() method is " + str(math.expm1(test_value)))
Output:
# method math.expm1() handles the precision more accurately
The result with exp()-1 method is 1.9984014443252818e-14
The result with expm1() method is 2.00000000000002e-14
Example Code: Same Method With numpy.expm1()
This method provides excellent precision than the exp(x)-1
method with a small value of x
. The value of x
is not a single value but an array-like parameter.
The numpy.expm1()
method has more than a single argument like out
(for storing the result), where
(optional bool value), etc. The numpy
module contains both the methods exp()
and expm1()
for a single and array value, respectively.
import numpy as np
# array method for expm1() method.
def numpy_array(x):
return np.expm1(x)
test_value = 1e-11
print("The result with expm1() method is ", numpy_array(test_value))
Output:
The result with expm1() method is 1.000000000005e-11
Example Code: Compute the log(1-exp(x))
Expression
The method logarithm()
provides a way to find the numerically stable computation of the log(1-exp(x))
expression. The method computes the log(1-exp(x))
by the use of the math.log(-math.expm1(x))
equation.
import math
# finds the numerically stable computation of log(1-exp(x)) expression
def logarithm(x):
# below comparison returns True. The rest of them are not compared.
if x < -1:
return math.log1p(-math.exp(x))
elif x < 0:
return math.log(-math.expm1(x))
elif x == 0:
return -np.inf
# input number must be non-positive; otherwise, else part will be returned.
else:
raise ValueError("Non-positive argument is required")
print("The mumerically stable computation of log(1-exp(x)) is", logarithm(-1e2))
Output:
The mumerically stable computation of log(1-exp(x)) is -3.720075976020836e-44
Example Code: Compute the log(1-expm1(x))
Expression
The method stable_implementation()
provides a way to find the numerically stable computation of the log(1-expm1(x))
expression. The method computes the log(1-expm1(x))
using the math.log(-math.expm1(x))
equation.
The method also takes the negative number as a parameter; otherwise, it returns the result as not a number.
import math
# this is a more stable implementation than the previous example
def stable_implementation(num):
# returns Nan, if the comparison shows True value
if num >= 0.0:
return "Not a number"
elif num > 0:
return math.log(-math.expm1(num))
else:
return math.log1p(-math.exp(num))
print(
"The numerically stable implementation of `log(1 - exp(val)) is",
stable_implementation(-4e-2),
)
Output:
The numerically stable implementation of `log(1 - exp(val)) is -3.2388091590903993