How to Reduce Fractions in Python
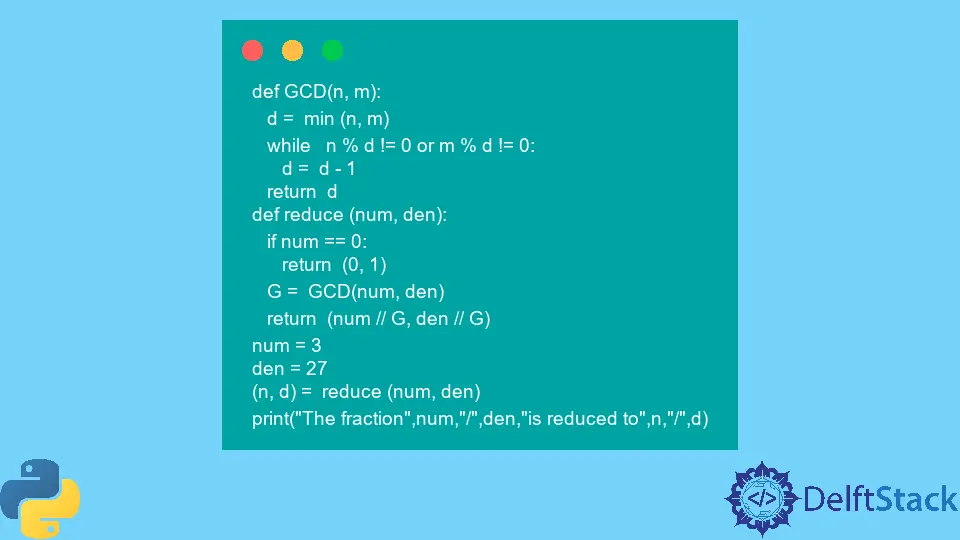
Python has functionality for arithmetic with rational numbers. It enables the creation of fraction instances from strings, numbers, decimals, floats, and integers.
A pair of integers, another rational number, a text, or a fraction can all be used to create a fraction instance. Since fraction instances can be hashed, they should be regarded as immutable.
Python Fractions
The Python Fraction
module provides arithmetic with rational numbers. With the help of this module, we may convert strings and other numeric variables, such as integers, floats, and decimals, into fractions.
The idea of a fraction instance exists. It is made up of two numbers acting as the numerator and denominator.
Undoubtedly, the Fraction
module is one of the least used parts of the Python standard library. Although it may not be widely recognized, it is a helpful tool since it can assist in fixing issues with binary floating-point arithmetic.
It is crucial if you intend to work with financial data or if your computations call for infinite precision.
Create Fractions in Python
You must import the Fraction
module from the fractions
library to create fractions in Python.
The fraction will change the decimal value 0.5
into 1/2
. Likewise, (21, 60)
will change into 7/20
, and the last fraction (0, 10)
will give a 0
output as the numerator is 0.
Example Code:
from fractions import Fraction
print(Fraction(0.5))
print(Fraction(21, 60))
print(Fraction(0, 10))
Output:
1/2
7/20
0
Reduce Fractions in Python
In the following example, we have reduced a fraction by simple steps.
First, we have defined a function GCD
(Greatest Common Divisor) and declared two integers n and m. Then set d
(denominator) to a minimum of m
and n
.
We use a while
loop to find the greatest common divisor. Then, we have defined another function, reduce()
, which returns the reduced fraction’s numerator and denominator.
The num
(fraction numerator) must be a non-zero integer. The reduced fraction is 0 if the num is 0; else, we find the greatest common divisor by calling the function.
We calculate the result by dividing the num
and den
by the GCD.
Example Code:
def GCD(n, m):
d = min(n, m)
while n % d != 0 or m % d != 0:
d = d - 1
return d
def reduce(num, den):
if num == 0:
return (0, 1)
G = GCD(num, den)
return (num // G, den // G)
num = 3
den = 27
(n, d) = reduce(num, den)
print("The fraction", num, "/", den, "is reduced to", n, "/", d)
Output:
The fraction 3 / 27 is reduced to 1 / 9
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn