How to Calculate Factorial in Python
- Calculate the Factorial of a Number Using Iteration in Python
- Calculate the Factorial of a Number Using Recursion in Python
-
Calculate the Factorial of a Number Using the
math.factorial()
Function in Python
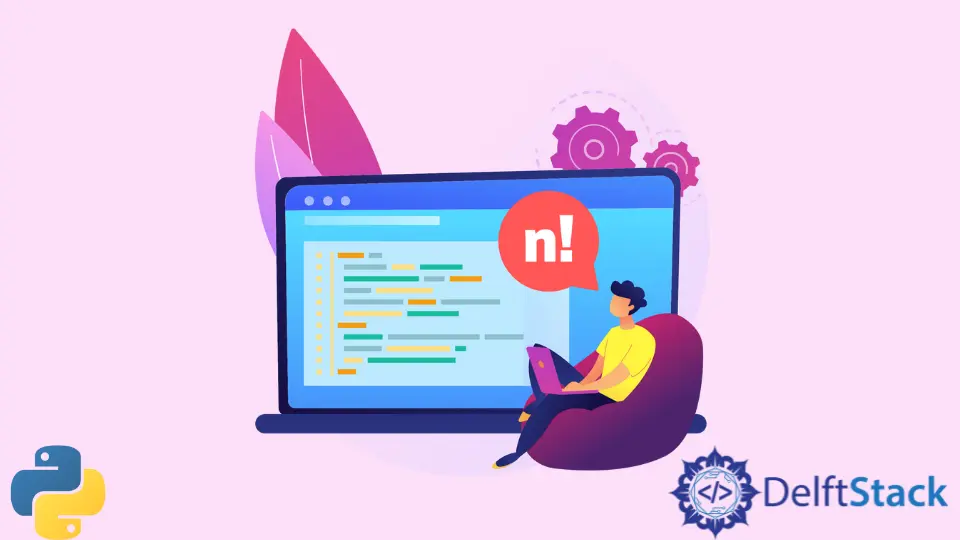
A factorial of a number is a product of all positive integers less than or equal to that number. For example, the factorial of 5 is the product of all the numbers which are less than and equal to 5, i.e 5 * 4 * 3 * 2 * 1
, which equals 120. Therefore, the factorial of number 5 is 120.
Now let’s write a Python function to calculate the factorial of a number. There are two ways in which we can write a factorial program in Python, one by using the iteration method and another by using the recursive method.
Calculate the Factorial of a Number Using Iteration in Python
The factorial program using the iteration method is nothing but using loops in our program like the for
loop or the while
loop. While writing an iterative program for factorial in Python we have to check three conditions.
- Given number is negative: If the number is negative, then we will simply say that we can’t find the factorial because the factorial of a negative number does not exist.
- Given number is zero: If the number is zero, then we will simply print
1
because the factorial of a number zero is1
. - Given number is positive: If the number is positive, then only we will find its factorial.
def factorial(num):
if num < 0:
print("Factorial of negative num does not exist")
elif num == 0:
return 1
else:
fact = 1
while num > 1:
fact *= num
num -= 1
return fact
num = 5
print("Factorial of", num, "is", factorial(num))
Output:
Factorial of 5 is 120
Calculate the Factorial of a Number Using Recursion in Python
Recursion is nothing but calling the same function again and again. Using recursion, we can write fewer lines of code, which will be much more readable than the code which we will be writing using the iterative method.
Whenever we call a recursion function, a recursion stack is created in memory. This recursion stack has something called a program counter, which keeps track of which instruction to be executed next after the recursion function finishes its execution.
def factorial(n):
return 1 if (n == 1 or n == 0) else n * factorial(n - 1)
num = 5
print("Factorial of", num, "is", factorial(num))
Output:
Factorial of 5 is 120
Calculate the Factorial of a Number Using the math.factorial()
Function in Python
Do you want to write a factorial function in just one line? Does it look impossible to you? There is a way of writing a factorial function in just one line of code. This can be done by using the math
module. Inside the math
module, there is a factorial
function to calculate a number’s factorial.
You have to import this function from the math module, call it inside your program and pass the number whose factorial you want to calculate. See the example below.
from math import factorial
print("Factorial is", factorial(5))
Output:
Factorial is 120
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn