How to Define an Infinite Value in Python
- Traditional Way to Define an Infinite Value in Python
- Define an Infinite Value Using the Math Module in Python
-
Define an Infinite Value Using the
Decimal()
Function in Python - Define an Infinite Value Using the NumPy Module in Python
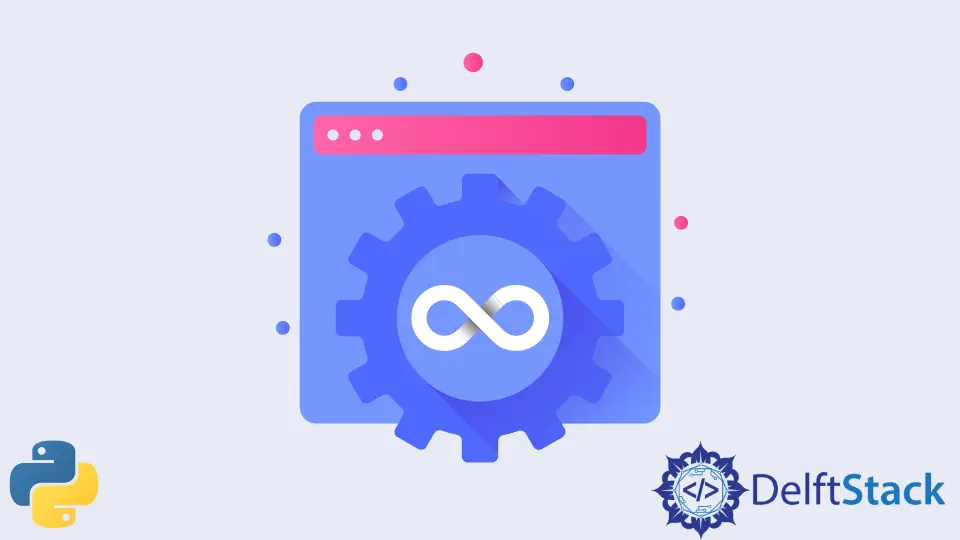
In this article, we will learn what is an infinite value in Python and why we use an infinite value. We will also learn how to define an infinite value with the help of different ways in Python.
Traditional Way to Define an Infinite Value in Python
When you hear infinity, most of you think there is something that never ends, but how do we treat infinity in Python? Suppose you need to store an infinity number in Python script; then, you can store a file with a bigger number even though you can store numbers with multiple files.
Since the Python language is dynamic, 999999999999
or a bigger number stored in multiple files will not be enough to define an infinite number in Python.
In Math, you can use finite and infinite numbers. Python brings a feature where we can define a finite number in a specific range and an infinite number, as in Math; we can use negative and positive infinity.
We cannot define an infinite number in other programming languages. In other programming languages, we can define a specific set of values.
To define infinity, we can use float("inf")
to define a positive infinite number and for a negative infinite number, we use float("-inf")
. Now, we will look at how it works in Python.
Suppose we have a value in variable a
that stores a large number, and the requirement says to check whether the a
variable is bigger than float("inf")
or not.
>>> a=99999999999999999
>>> a_INF=float("inf")
>>> a_INF > a
True
>>> a_INF
inf
We can see that a_INF
is greater than a
because this is an infinite number, so here is one simple way to define an infinite number without importing an additional module.
We can also define a negative infinite number. An example is given below.
>>> Neg_INF=float("-inf")
>>> Neg_INF
-inf
Define an Infinite Value Using the Math Module in Python
Another feature to define an infinite number is math.inf
. In the math
module, inf
is a variable where an infinite value is located.
First, we will need to import it, and then we can declare a variable as an infinite using math.inf
. Let’s look at how we can do this.
>>> import math
>>> M_INF=math.inf
>>> M_INF
inf
Like how we can define positive and negative infinity without importing the math
module, we can also define either positive or negative infinite using the math
module. Let’s take a look.
>>> M_NEG_INF=-math.inf
>>> M_NEG_INF
-inf
Define an Infinite Value Using the Decimal()
Function in Python
The third method available in Python to define an infinite number is using the decimal
module. The decimal
module is another new data type.
The reason why they created this data type is that floating point numbers were not accurate. A decimal is a floating point number, but it is handled differently.
While importing the decimal
module, we can use the Decimal()
function to define either a positive or negative infinite value.
In the Decimal()
function, we can pass Infinity
as a string value, but this will be for a positive value. If we want to define negative, we will include the minus sign like -Infinity
.
Let’s look at how to use the Decimal()
function and its syntax. For a better understanding, we will create an example with a small piece of code.
First, we will import the Decimal
function from the decimal
module so that we can store an infinite number in memory using a variable. Let’s take a look.
>>> from decimal import Decimal
>>> INF_POS_VAL=Decimal('infinity')
>>> INF_Neg_VAL=Decimal('-infinity')
>>> print(INF_POS_VAL)
Infinity
>>> print(INF_Neg_VAL)
-Infinity
So this way, we get an infinite value and use it in different programs for different purposes.
Define an Infinite Value Using the NumPy Module in Python
The last and most common way to define an infinite value is using the numpy
module. The numpy
module also brings a feature to define an infinite value using numpy.inf
.
This works the same as the math
module does. Just the module name is changed.
Let’s take a look.
>>> import numpy
>>> Num_Pos_INF=numpy.inf
>>> Num_Neg_INF=-numpy.inf
>>> print(Num_Pos_INF)
inf
>>> print(Num_Neg_INF)
-inf
In different Python scripts where we handle the scientific calculation, we will need to check whether a value is infinite or not. We can check this using Python’s isinf()
method.
We cannot call it normally, but we can call it after importing the math
because isinf()
is a function of the math
module. Since the isinf()
function returns Boolean values like true or false, we will put a value to determine whether the value is infinite or not.
If we check a value made with integers or float using the isinf()
function, this function returns false because this is a finite value.
>>> import math
>>> val=999999999999
>>> math.isinf(val)
False
In which case will this return true?
It will return true when we pass either a negative or positive infinite value. In both cases, we will get a true value.
>>> math.isinf(-numpy.inf)
True
So this way, we can define the larger and smaller value in Python. For more details, you can visit here.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn