NumPy numpy.dot Function
Suraj Joshi
Jan 30, 2023
NumPy
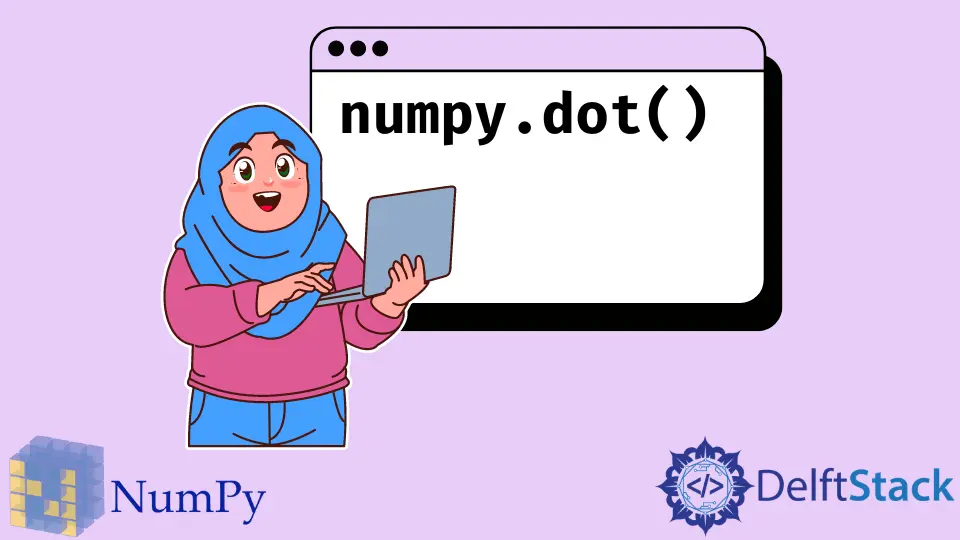
Python Numpynumpy.dot()
function calculates the dot product of two input arrays.
Syntax of numpy.dot()
:
numpy.dot(a, b, out=None)
Parameters
a |
Array-like. 1st array or scalar whose dot product is be calculated |
b |
Array-like. 2nd array or scalar whose dot product is be calculated |
out |
Array. An optional argument whose data-type must be the same as the expected data-type of output |
Return
It returns the dot product of input vectors. If both inputs are scalars, it produces a 1-D array otherwise an n-dimensional array.
Raise ValueError
if the last dimension of the first input array is not equal to the second-to-last dimension of the 2nd input array.
Example Codes: numpy.dot()
Method to Find Dot Product
When Both Inputs Are 1-D Arrays
import numpy as np
a=4
b=5
prod=np.dot(a,b)
print(prod)
Output:
20
Here, since both a
and b
are 1-D arrays, the np.dot()
function simply returns a scalar, which is a simple product of both the numbers.
When Both Inputs Are Vectors
import numpy as np
a=np.array([3,4])
b=np.array([4,5])
prod=np.dot(a,b)
print(prod)
Output:
32
It calculates the dot product of vectors.
The dot product of two vectors [x1,y1]
and [x2,y2]
is given by x1*x2+y1*y2
.
When Both Inputs Are 2-Dimensional Arrays
import numpy as np
a=np.array([[3,4],
[2,3]])
b=np.array([[4,5],
[2,3]])
prod=np.dot(a,b)
print(prod)
Output:
[[20 27]
[14 19]]
It calculates the product of matrices.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn