How to Fix the Iteration Over a 0-D Array Error in Python NumPy
Vaibhav Vaibhav
Feb 02, 2024
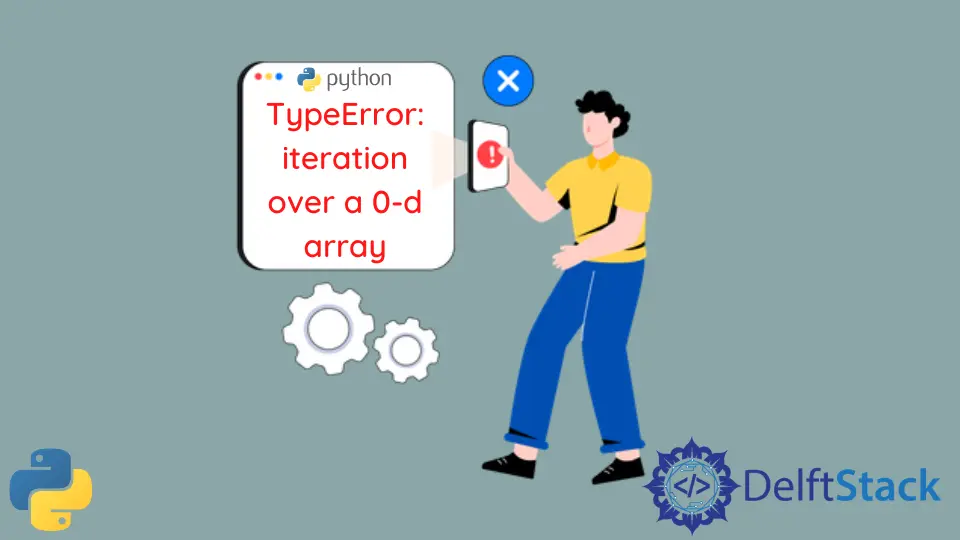
The error TypeError: iteration over a 0-d array
occurs when iteration is performed over an iterable of 0
dimension. In this article, we will learn how to fix the TypeError: iteration over a 0-d array
error in Python NumPy.
How to Fix the TypeError: iteration over a 0-d array
Error Due in Python NumPy
The following Python code depicts a scenario where we can run into this error.
import numpy as np
data = {
"AB": 1.01,
"CD": 2.02,
"EF": 3.03,
"GH": 4.04,
"IJ": 5.05,
}
keys, values = np.array(data.items()).T
print(keys)
print(values)
Output:
Traceback (most recent call last):
File "<string>", line 11, in <module>
TypeError: iteration over a 0-d array
The reason behind this error is the data type of data.items()
, which is <class 'dict_items'>
. To avoid this error, we have to convert its data type to a list or a tuple. The following Python code shows how to fix this error using a list and a tuple.
Solution using a list
.
import numpy as np
data = {
"AB": 1.01,
"CD": 2.02,
"EF": 3.03,
"GH": 4.04,
"IJ": 5.05,
}
print(type(list(data.items())))
keys, values = np.array(list(data.items())).T
print(keys)
print(values)
Output:
<class 'list'>
['AB' 'CD' 'EF' 'GH' 'IJ']
['1.01' '2.02' '3.03' '4.04' '5.05']
Below is a solution using a tuple
.
import numpy as np
data = {
"AB": 1.01,
"CD": 2.02,
"EF": 3.03,
"GH": 4.04,
"IJ": 5.05,
}
print(type(tuple(data.items())))
keys, values = np.array(tuple(data.items())).T
print(keys)
print(values)
Output:
<class 'tuple'>
['AB' 'CD' 'EF' 'GH' 'IJ']
['1.01' '2.02' '3.03' '4.04' '5.05']
Author: Vaibhav Vaibhav
Related Article - Python Array
- How to Initiate 2-D Array in Python
- How to Count the Occurrences of an Item in a One-Dimensional Array in Python
- How to Downsample Python Array
- How to Sort 2D Array in Python
- How to Create a BitArray in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python