How to Sort 2D Array in Python
-
Sort 2D Array by Column Number Using the
sort()
Function in Python -
Sort 2D Array by Column Number Using the
sorted()
Function in Python
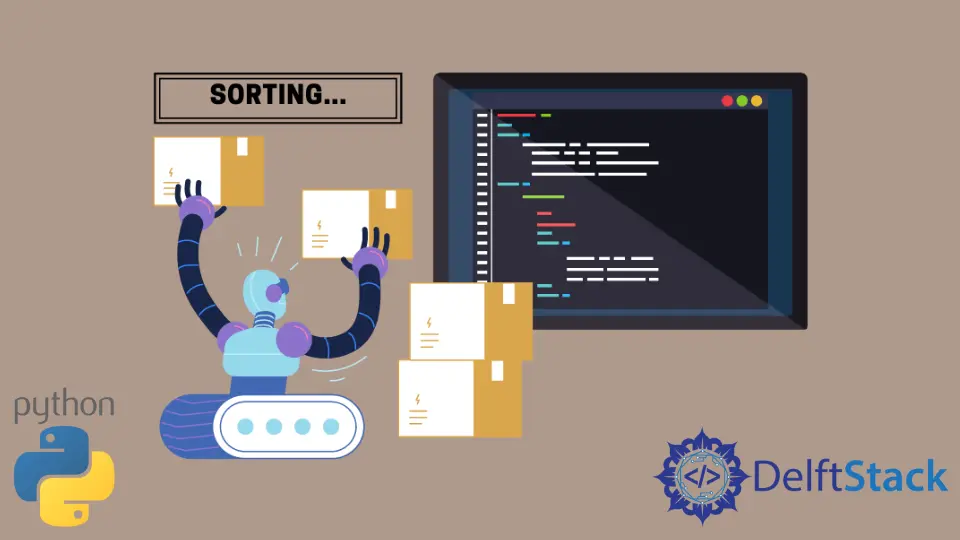
We will introduce different methods to sort multidimensional arrays in Python.
There are built-in function such as sort()
and sorted()
for array sort; these functions also allows us to take a specific key that we can use to define which column to sort if we want.
The sort()
method modifies the list in-place, and a sorted()
built-in function builds a new sorted list from an iterable. We will also look at the different methods to define iterable for sort()
and sorted()
functions.
Sort 2D Array by Column Number Using the sort()
Function in Python
In order to sort array by column number we have to define the key
in function sort()
such as,
lst = [["John", 5], ["Jim", 9], ["Jason", 0]]
lst.sort(key=lambda x: x[1])
print(lst)
Output:
[['Jason', 0], ['John', 5], ['Jim', 9]]
For sorting reasons, the key
parameter should be set to the value of a function that accepts a single argument and returns a key
that may be used in the sorting process. It is possible to do this strategy quickly because the key function is called just once for each input record.
A frequently used pattern is to sort complex objects using one or more of the object’s indices as a key.
lst = [
("john", "C", 15),
("jane", "A", 12),
("dave", "D", 10),
]
lst.sort(key=lambda lst: lst[2])
print(lst)
Output:
[('dave', 'D', 10), ('jane', 'A', 12), ('john', 'C', 15)]
In the above code at key=lambda lst:lst[2]
, the lst[2]
defines which column should be used for the sort basis. In our case, lst
is sorted by the third column.
Sort 2D Array by Column Number Using the sorted()
Function in Python
In order to sort array by column number we have to define the key
in function sorted()
such as,
li = [["John", 5], ["Jim", 9], ["Jason", 0]]
sorted_li = sorted(li, key=lambda x: x[1])
print(sorted_li)
Output:
[['Jason', 0], ['John', 5], ['Jim', 9]]
Note that the sorted()
function returns a new list in the preceding code, whereas the sort()
function replaces the original list.
The key
can also be defined alternatively using itemgetter
from the library operator
.
from operator import itemgetter
lst = [
("john", "C", 15),
("jane", "A", 12),
("dave", "D", 10),
]
sorted_lst = sorted(lst, key=itemgetter(1))
print(sorted_lst)
Output:
[('jane', 'A', 12), ('john', 'C', 15), ('dave', 'D', 10)]
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn