How to Subtract Datetime in Python
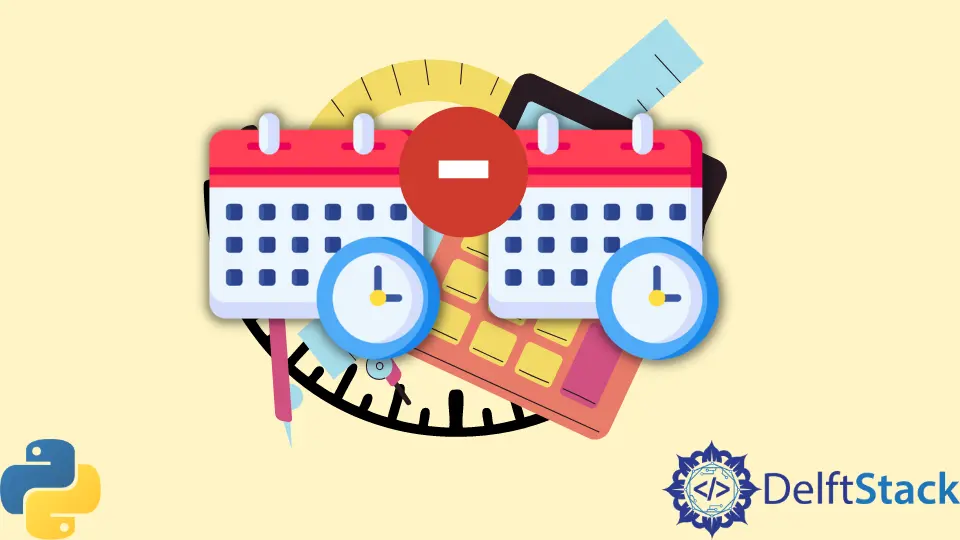
This tutorial will introduce how to perform datetime subtraction in Python.
We will explore different outputs after subtraction, like outputting the number of seconds, minutes, hours, or years of the difference of the two datetime objects provided.
Use the datetime
Module to Subtract Datetime in Python
datetime
is a module in Python that will support functions that manipulate the datetime
object.
Initializing a datetime
object takes three required parameters as datetime.datetime(year, month, day)
. It also accepts optional parameters in the form of hours, minutes, seconds, microseconds, and timezone.
datetime
also has the function now()
, which initializes the current date and time into the object.
Subtracting two datetime objects will return the difference of the number of days, and the difference in time, if there is any.
from datetime import datetime
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print(now - then)
Output:
12055 days, 5:10:00
The result means that the two dates are 12055 days and 5 hours and 10 minutes apart.
To convert this result into different formats, we would first have to convert the timedelta
into seconds. The datetime object has a built-in method datetime.total_seconds()
converting the object into the total seconds it contains.
Here’s the full source code for datetime subtraction using the datetime
module and its functions.
from datetime import datetime
def getDifference(then, now=datetime.now(), interval="secs"):
duration = now - then
duration_in_s = duration.total_seconds()
# Date and Time constants
yr_ct = 365 * 24 * 60 * 60 # 31536000
day_ct = 24 * 60 * 60 # 86400
hour_ct = 60 * 60 # 3600
minute_ct = 60
def yrs():
return divmod(duration_in_s, yr_ct)[0]
def days():
return divmod(duration_in_s, day_ct)[0]
def hrs():
return divmod(duration_in_s, hour_ct)[0]
def mins():
return divmod(duration_in_s, minute_ct)[0]
def secs():
return duration_in_s
return {
"yrs": int(yrs()),
"days": int(days()),
"hrs": int(hrs()),
"mins": int(mins()),
"secs": int(secs()),
}[interval]
then = datetime(1987, 12, 30, 17, 50, 14) # yr, mo, day, hr, min, sec
now = datetime(2020, 12, 25, 23, 13, 0)
print("The difference in seconds:", getDifference(then, now, "secs"))
print("The difference in minutes:", getDifference(then, now, "mins"))
print("The difference in hours:", getDifference(then, now, "hrs"))
print("The difference in days:", getDifference(then, now, "days"))
print("The difference in years:", getDifference(then, now, "yrs"))
The first thing that the code does is to initialize 2 separate dates for subtraction and calling the function multiple times with different outputs each time. In the case of all
, it will return an output that gets the difference based on all the cases from years to seconds.
The divmod()
function accepts two numbers as the dividend and divisor and returns a tuple of the quotient and the remainder between them. Only the quotient is needed, and that’s why only the 0
index is used through the code.
Output:
The difference in seconds: 1041052966
The difference in minutes: 17350882
The difference in hours: 289181
The difference in days: 12049
The difference in years: 33
Now we have the difference between two datetime objects within several different time frames.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn